Learn how to implement user authentication to access a secure ArcGIS service with OAuth credentials.
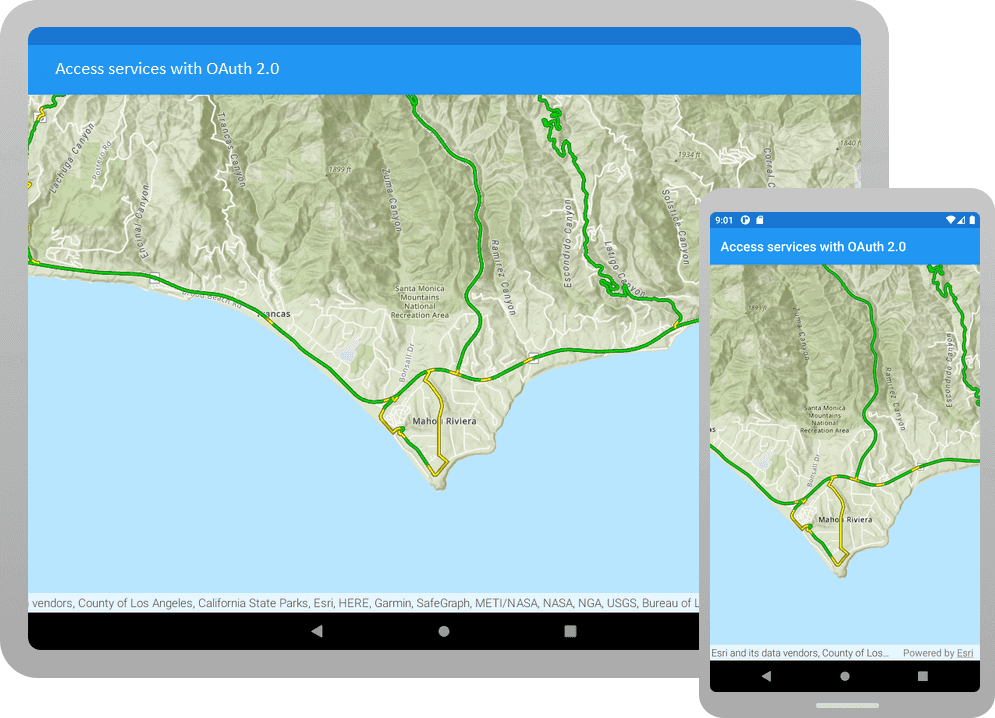
You can use different types of authentication to access secured ArcGIS services. To implement OAuth credentials for user authentication, you can use your ArcGIS account to register an app with your portal and get a Client ID, and then configure your app to redirect users to login with their credentials when the service or content is accessed. This is known as user authentication. If the app uses premium ArcGIS Online services that consume credits, for example, the app user's account will be charged.
In this tutorial, you will build an app that implements user authentication using OAuth credentials so users can sign in and be authenticated through ArcGIS Online to access the ArcGIS World Traffic service.
Prerequisites
Before starting this tutorial, you need the following:
-
An ArcGIS Location Platform or ArcGIS Online account.
-
A development and deployment environment that meets the system requirements.
-
An IDE for Android development in Kotlin.
Steps
Create OAuth credentials for user authentication
OAuth credentials are required to implement user authentication. These credentials are created and stored as an Application item in your organization's portal.
-
Go to the Create OAuth credentials for user authentication tutorial and create OAuth credentials using your ArcGIS Location Platform or ArcGIS Online account.
-
Copy the
Client ID
andRedirect URL
as you will use them to implement user authentication later in this tutorial. TheClient ID
is found on the Application item's Overview page, while theRedirect URL
is found on the Settings page.
The Client ID
uniquely identifies your app on the authenticating server. If the server cannot find an app with the provided Client ID, it will not proceed with authentication.
The Redirect URL
(also referred to as a callback url) is used to identify a response from the authenticating server when the system returns control back to your app after an OAuth login. Since it does not necessarily represent a valid endpoint that a user could navigate to, the redirect URL can use a custom scheme, such as my-app
. You can configure several redirect URLs in your application definition and can remove or edit them. It's important to make sure the redirect URL used in your app's code matches a redirect URL configured for the application.
- The redirect URL consists of three parts:
- the scheme
- the separator string "://"
- the host
- Scheme: You are probably familiar with standard schemes used in internet URLs:
http
,https
,ftp
, etc. In OAuth 2.0, you can create a private-use URI scheme (also known as custom URL scheme), which will only be used by the authorization server and your app. This is an arbitrary string whose allowed characters are declared in the Uniform Resource Locators (URL) Spec . Though not strictly required, making your scheme unique is a good practice. Some organization use the app ID or the reverse domain of their company (com.mycompany.appname) as the URL scheme. - Host: An arbitrary string occupying the host portion of the URL. It allows you to use the same URL scheme with different URL hosts.
Open an Android Studio project with Gradle
-
Open the project you created by completing the Display a map tutorial.
-
Continue with the following instructions to access the World Traffic Service using OAuth 2.0 and display it as a feature layer.
-
Modify the old project for use in this new tutorial.
-
On your file system, delete the .idea folder, if present, at the top level of your project.
-
In the Android view, open app > res > values > strings.xml.
In the
<string name="app
element, change the text content to Access services with OAuth 2.0._name" > strings.xmlUse dark colors for code blocks <resources> <string name="app_name">Access services with OAuth 2.0</string> </resources>
-
In the Android view, open Gradle Scripts > settings.gradle.kts.
Change the value of
root
to "Access services with OAuth 2.0".Project.name settings.gradle.ktsUse dark colors for code blocks dependencyResolutionManagement { repositoriesMode.set(RepositoriesMode.FAIL_ON_PROJECT_REPOS) repositories { google() mavenCentral() maven { url = uri("https://esri.jfrog.io/artifactory/arcgis") } } } rootProject.name = "Access services with OAuth 2.0" include(":app")
-
Click File > Sync Project with Gradle files. Android Studio will recognize your changes and create a new .idea folder.
-
-
Open the app > kotlin+java > com.example.app > MainActivity.kt. Delete the code in the
Main
class, leaving only the class declaration.Activity MainActivity.ktUse dark colors for code blocks Copy class MainActivity : ComponentActivity() {
Add imports
-
Open app > kotlin+java > com.example.app > screens > MainScreen.kt. Modify import statements to reference the packages and classes required for this tutorial.
MainScreen.ktUse dark colors for code blocks @file:OptIn(ExperimentalMaterial3Api::class) package com.example.app.screens import androidx.compose.foundation.layout.fillMaxSize import androidx.compose.foundation.layout.padding import androidx.compose.material3.ExperimentalMaterial3Api import androidx.compose.material3.Scaffold import androidx.compose.material3.Text import androidx.compose.material3.TopAppBar import androidx.compose.runtime.Composable import androidx.compose.runtime.remember import androidx.compose.ui.Modifier import androidx.compose.ui.res.stringResource import com.arcgismaps.mapping.ArcGISMap import com.arcgismaps.mapping.BasemapStyle import com.arcgismaps.mapping.Viewpoint import com.arcgismaps.mapping.layers.ArcGISMapImageLayer import com.arcgismaps.toolkit.geoviewcompose.MapView import com.example.app.R
Add a traffic layer to the map
You will add a layer to display the ArcGIS World Traffic service, a dynamic map service that presents historical and near real-time traffic information for different regions in the world. This is a secure service and requires an ArcGIS Online organizational subscription.
ArcGIS World Traffic service data is updated every five minutes to provide traffic speed and traffic incident visualization and identification.
Traffic speeds are displayed as a percentage of free-flow speeds, which is frequently the speed limit or how fast cars tend to travel when unencumbered by other vehicles. The streets are color coded as follows:
- Green (fast): 85 - 100% of free flow speeds
- Yellow (moderate): 65 - 85%
- Orange (slow); 45 - 65%
- Red (stop and go): 0 - 45%
-
In MainScreen.kt, create an
ArcGISMapImageLayer
to display the traffic service.MainScreen.ktUse dark colors for code blocks fun createMap(): ArcGISMap { // Create a layer to display the ArcGIS World Traffic service val trafficLayer = ArcGISMapImageLayer("https://traffic.arcgis.com/arcgis/rest/services/World/Traffic/MapServer") return ArcGISMap(BasemapStyle.ArcGISTopographic).apply { initialViewpoint = Viewpoint( latitude = 34.02700, longitude = -118.80543, scale = 72000.0 ) } }
-
Add the layer to the map's collection of data layers (operational layers).
MainScreen.ktUse dark colors for code blocks fun createMap(): ArcGISMap { // Create a layer to display the ArcGIS World Traffic service val trafficLayer = ArcGISMapImageLayer("https://traffic.arcgis.com/arcgis/rest/services/World/Traffic/MapServer") return ArcGISMap(BasemapStyle.ArcGISTopographic).apply { initialViewpoint = Viewpoint( latitude = 34.02700, longitude = -118.80543, scale = 72000.0 ) operationalLayers.add(trafficLayer) } }
Integrate OAuth credentials into your app
The ArcGIS Maps SDK for Kotlin toolkit contains classes and composable functions that abstract some of the detail of implementing OAuth 2.0 authentication in your app.
First you will create an AuthenticatorState
and then instantiate OAuthUserConfiguration
and assign it to the Authenticator
property. You will need your OAuth Client ID
and Redirect URL
when you instantiate O
.
Then you will call the Dialog
composable to display the a log-in screen.
The Dialog
composable displays an Authentication UI when an authentication challenge is issued. The Authenticator
interface handles authentication challenges and exposes the state for the Dialog
to display to the user. Both are defined in the authentication
component of the ArcGIS Maps SDK for Kotlin Toolkit.
-
In
Main
add anActivity.kt authenticator
property to the MainActivity class.MainActivity.ktUse dark colors for code blocks class MainActivity : ComponentActivity() { private val authenticatorState = AuthenticatorState()
-
Add the
on
lifecycle method to the MainActivity class.Create() MainActivity.ktUse dark colors for code blocks class MainActivity : ComponentActivity() { private val authenticatorState = AuthenticatorState() override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) enableEdgeToEdge() setContent { TutorialTheme { MainScreen() } } } }
-
In
on
, instantiateCreate() OAuthUserConfiguration
, passing yourclient
and yourId redirect
.Url MainActivity.ktUse dark colors for code blocks class MainActivity : ComponentActivity() { private val authenticatorState = AuthenticatorState() override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) authenticatorState.oAuthUserConfiguration = OAuthUserConfiguration( portalUrl = "https://www.arcgis.com", clientId = "your_client_id", redirectUrl = "your_redirect_url" ) enableEdgeToEdge() setContent { TutorialTheme { MainScreen() } } } }
The value of
portal
in the code block isUrl https
. Alternatively, it could be the URL of your ArcGIS Online organization. In both cases, the Android Custom Tab will display a log-in for the user's credentials.://www.arcgis.com Note that any other values for
portal
will result in a default log-in screen for the user credentials, which are then authenticated using ArcGIS token instead of OAuth. If you see the following screen in this tutorial, you are not being authenticated with OAuth. Verify the paramters you pass to theUrl O
constructor inAuth Configuration Main
and theActivity android
and:scheme android
attributes of the:host data
element inAndroid
.Manifest.xml -
Inside the
Tutorial
block, call theTheme DialogAuthenticator
composable, passing inauthenticator
.State MainActivity.ktUse dark colors for code blocks class MainActivity : ComponentActivity() { private val authenticatorState = AuthenticatorState() override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) authenticatorState.oAuthUserConfiguration = OAuthUserConfiguration( portalUrl = "https://www.arcgis.com", clientId = "your_client_id", redirectUrl = "your_redirect_url" ) enableEdgeToEdge() setContent { TutorialTheme { MainScreen() DialogAuthenticator(authenticatorState) } } } }
-
Open app > manifests > AndroidManifest.xml and locate the
<activity
tag for the OAuth user sign-in activity. The> OAuthUserSignInActivity
is defined in the ArcGIS Maps SDK for Kotlin toolkit.The
OAuthUserSignInActivity
class is an activity that launches a CustomTabs activity and receives the redirect intent that is sent when the user completes the CustomTabs prompt. For more information, see theREADME
for theauthentication
component in the ArcGIS Maps SDK for Kotlin Toolkit. -
The
<data
element of> OAuthUserSignInActivity
has anandroid
attribute and and:scheme android
where you must specify the scheme and the host of your OAuth redirect URL. See Create OAuth credentials for user authentication above for details on redirect URL, scheme, and host.:host AndroidManifest.xmlUse dark colors for code blocks <activity android:name="com.arcgismaps.toolkit.authentication.OAuthUserSignInActivity" android:configChanges="keyboard|keyboardHidden|orientation|screenSize" android:exported="true" android:launchMode="singleTop" > <intent-filter> <action android:name="android.intent.action.VIEW" /> <category android:name="android.intent.category.DEFAULT" /> <category android:name="android.intent.category.BROWSABLE" /> <data android:scheme="your_redirect_url_scheme" android:host="your_redirect_url_host" /> </intent-filter> </activity>
-
Click Run > Run > app to run the app.
When running your app, you will be prompted for username and password in an Android Custom Tab.
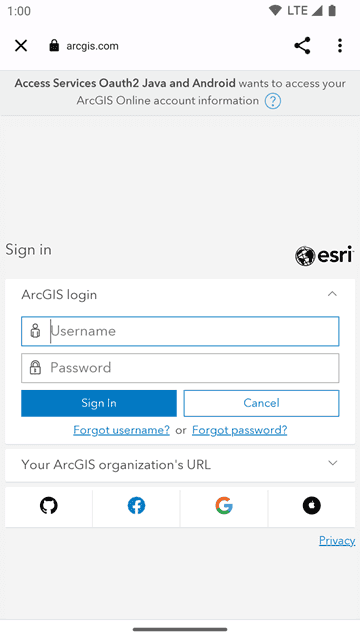
After successfully logging in with your ArcGIS Online credentials, you should see the map with the topographic basemap layer centered on the Santa Monica Mountains in California. You will also see the traffic layer, with its symbology of green, yellow, orange, and red roads to indicate current traffic flow. This is a secured layer, which is visible in your app because the user has entered valid ArcGIS Online username and password.
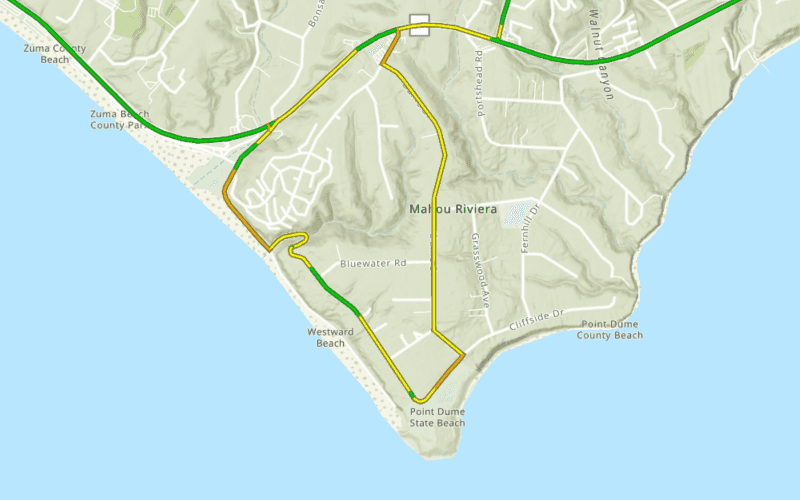
What's next?
Learn how to use additional API features, ArcGIS location services, and ArcGIS tools in these tutorials: