Learn how to display a map from a mobile map package (MMPK).
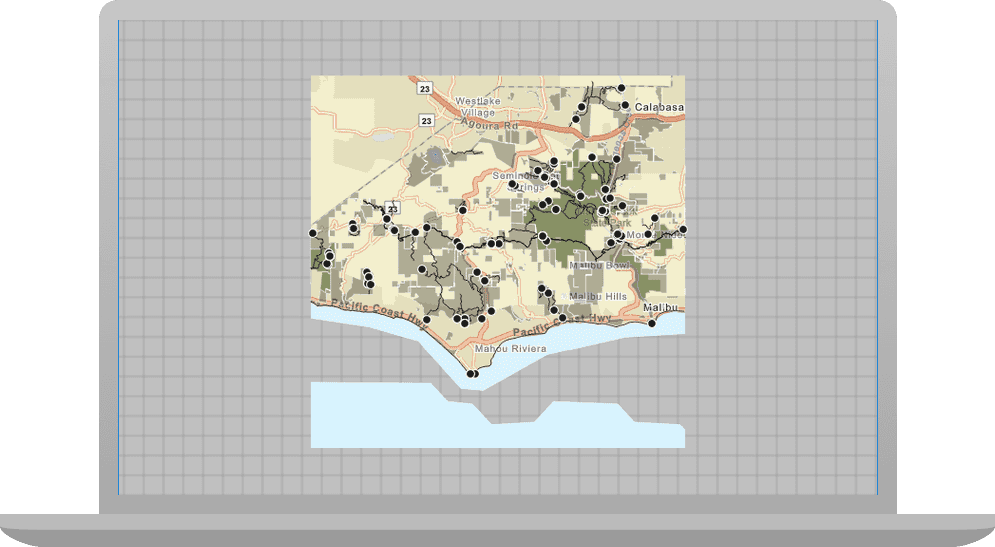
In this tutorial you will display a fully interactive map from a mobile map package (MMPK). The map contains a basemap layer and data layers and does not require a network connection.
Prerequisites
Before starting this tutorial:
-
You need an ArcGIS Location Platform or ArcGIS Online account.
-
Your system meets the system requirements.
-
The ArcGIS Maps SDK for Qt, version 200.4.0 or later is installed.
-
The Qt 6.5.1 software development framework is installed.
Steps
Get the mobile map package
-
Download or create the MahouRivieraTrails.mmpk mobile map package. You can download the mobile map package from here: MahouRivieraTrails.mmpk. Otherwise, complete the steps in the Create a mobile map package tutorial, using ArcGIS Pro to create the package.
-
Copy the file MahouRivieraTrails.mmpk to a local path on your hard drive (for example: C:/tutorial_mmpk/MahouRivieraTrails.mmpk). Make a note of this path as you will need supply it in the function
setup
towards the end of this tutorial.M a p From Mmpk()
Create a new ArcGIS Maps Qt Creator Project
-
Select the ArcGIS Maps 200.4.0 Qt Quick C++ app project template (or a later version) and click Choose.
You may have several selections for the ArcGIS project type. Be sure to select ArcGIS Maps 200.4.0 Qt Quick C++ app (or a later version).
-
Name your project display_an_mmpk and specify the "Create in" directory a location of your choosing. Click Next.
-
In the Define Build System dialog, select qmake for your build system. Click Next.
-
In the Define Project Details dialog, if desired give this app a description or keep the default text. Leave the rest of this dialog as is. Then click Next.
-
On the Kit Selection dialog, check the kit(s) you previously set up when you installed the API. You should select a Desktop kit to run this tutorial (for example Desktop Qt 6.5.0 MSVC2019 64bit). Then click Next.
-
At the Project Management dialog, the option to Add as a subproject to root project is only available if you have already created a root project. Ignore this dialog for this tutorial. Click Next.
Modify the project to remove unused template code from the header file
The project you created from the ArcGIS Maps Qt Quick C++ app project template includes a class for the Map
. Because the mobile map package (MMPK) contains the map you will remove the unneeded code from your project.
-
In the Edit tab, double-click Headers > Display_an_mmpk.h to open the file. Remove the code that forward declares the
Map
class.Display_an_mmpk.hUse dark colors for code blocks #ifndef DISPLAY_AN_MMPK_H #define DISPLAY_AN_MMPK_H namespace Esri::ArcGISRuntime { class Map;
-
Now remove the code that creates the
Map
pointer variablem_
.map Display_an_mmpk.hUse dark colors for code blocks private: Esri::ArcGISRuntime::MapQuickView* mapView() const; void setMapView(Esri::ArcGISRuntime::MapQuickView* mapView); Esri::ArcGISRuntime::Map* m_map = nullptr;
Modify the project to remove the unused template code from the C++ file
The project you created from the ArcGIS Maps Qt Quick C++ app project template includes: references to Map
and Map
header files, a call to a constructor to initialize a new Map
based on a Basemap
, and a call to the Map
method that are not needed. Because the mobile map package (MMPK) contains the map you will remove the unneeded code from your project.
-
In Edit tab, double-click Sources > Display_an_mmpk.cpp to open the file. Remove the lines
#include "Map.h"
and#include "Map
.Types.h" Display_an_mmpk.cppUse dark colors for code blocks #include "Display_an_mmpk.h" #include "Map.h" #include "MapTypes.h" #include "MapQuickView.h"
-
Remove the comma after
QObject(parent)
and then modify the constructor to remove initialization withBasemap
and theStyle Map
. (Note that theBasemap
configured for your project may be different than that shown here.)Style Display_an_mmpk.cppUse dark colors for code blocks using namespace Esri::ArcGISRuntime; Display_an_mmpk::Display_an_mmpk(QObject* parent /* = nullptr */): QObject(parent), m_map(new Map(BasemapStyle::ArcGISStreets, this))
-
Remove the line of code that assigns the variable
m_
tomap m_
.map View Display_an_mmpk.cppUse dark colors for code blocks // Set the view (created in QML) void Display_a_map::setMapView(MapQuickView* mapView) { if (!mapView || mapView == m_mapView) { return; } m_mapView = mapView; m_mapView->setMap(m_map);
Modify the project to use a mobile map package in the header file
The mobile map package contains the map
, basemap
, and all the data layers it requires. We will modify the header file to declare the new function to implement loading the mobile map package (MMPK).
-
Under
private
, declare the new function you will implement to load the mobile map package. Then save and close the file.Display_an_mmpk.hUse dark colors for code blocks Add line. private: Esri::ArcGISRuntime::MapQuickView* mapView() const; void setMapView(Esri::ArcGISRuntime::MapQuickView* mapView); void setupMapFromMmpk();
Modify the project to use a mobile map package in the C++ file
The mobile map package contains the map
, basemap
, and all the data layers it requires. We will modify the C++ file to add the required include statements, provide the function that performs the work to load the mobile map package (MMPK), and call the function.
-
Add
#include
statements for theMobileMapPackage
, andError
classes.Display_an_mmpk.cppUse dark colors for code blocks Add line. Add line. #include "Display_an_mmpk.h" #include "MapQuickView.h" #include "MobileMapPackage.h" #include "Error.h"
-
Add a call to a function that reads the map from the mobile map package. You will implement this function in the next step.
Display_an_mmpk.cppUse dark colors for code blocks Add line. // Set the view (created in QML) void Display_an_mmpk::setMapView(MapQuickView* mapView) { if (!mapView || mapView == m_mapView) { return; } m_mapView = mapView; setupMapFromMmpk(); emit mapViewChanged();
-
Add code to implement
setup
. This function defines the path to the MMPK file, instantiates the mobile map package using theM a p From Mmpk() MobileMapPackage
constructor, loads the mobile map package, and once loaded, sets the first map in the mobile map package to theMapView
. This also checks that the MMPK file loaded correctly. IMPORTANT: Make sure and provide correct path to the MMPK file on your hard drive for the string <PATH_TO_MMPK_FILE>/MahouRivieraTrails.mmpk (for example: C:/tutorial_mmpk/MahouRivieraTrails.mmpk).Display_an_mmpk.cppUse dark colors for code blocks Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. setupMapFromMmpk(); emit mapViewChanged(); } void Display_an_mmpk::setupMapFromMmpk() { // Instantiate a MobileMapPackage object and establish the path to the MMPK file. MobileMapPackage* m_mobileMapPackage = new MobileMapPackage("<PATH_TO_MMPK_FILE>/MahouRivieraTrails.mmpk", this); // Use connect to signal when the package is done loading so that m_mapView can be set to the first map (0). // Check that the mmpk file has loaded correctly. connect(m_mobileMapPackage, &MobileMapPackage::doneLoading, this, [m_mobileMapPackage, this](Error error) { // Check that the mmpk file has loaded correctly if (!error.isEmpty()) { qDebug() << "Error:" << error.message()<< error.additionalMessage(); return; } // Get the first map in the list of maps, and set the map on the map view to display. // This could be set to any map in the list. m_mapView->setMap(m_mobileMapPackage->maps().at(0)); }); m_mobileMapPackage->load(); }
A
MobileMapPackage
can contain many maps in aMaps
list. Loading the mobile map package is an asynchronous process. The file is read on a thread that does not block the UI. -
Press Ctrl + R to run the app.
You see a map of trailheads, trails, and parks for the area south of the Santa Monica mountains. Drag, zoom in, and zoom out to explore the map.
What's next?
Learn how to use additional API features, ArcGIS location services, and ArcGIS tools in these tutorials: