Learn how to create and display a scene from a web scene stored in ArcGIS.
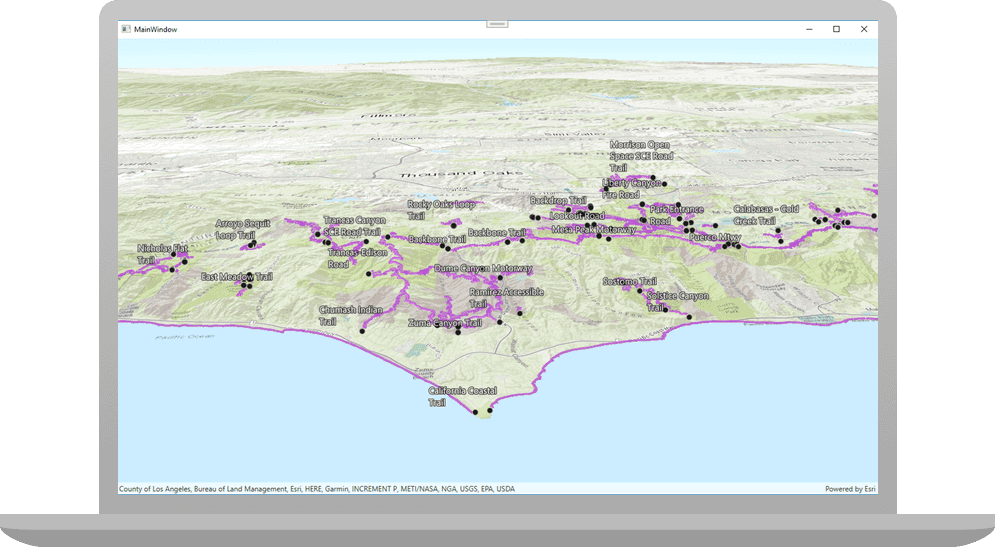
This tutorial shows you how to create and display a scene from a web scene. All web scenes are stored in ArcGIS with a unique item ID. You will access an existing web scene by item ID and display its layers. The web scene contains feature layers for the Santa Monica Mountains in California.
Prerequisites
Before starting this tutorial:
-
You need an ArcGIS Location Platform or ArcGIS Online account.
-
Your system meets the system requirements.
-
The ArcGIS Maps SDK for Qt, version 200.4.0 or later is installed.
-
The Qt 6.5.1 software development framework is installed.
Open the project in Qt Creator
-
To start this tutorial, complete the Display a scene tutorial or download and unzip the solution.
-
Open the display_a_scene project in Qt Creator.
-
If you downloaded the solution, get an access token and set the API key.
An API Key gives your app access to secure resources used in this tutorial.
-
Go to the Create an API key tutorial to obtain a new API key access token. Ensure that the following privilege is enabled: Location services > Basemaps > Basemap styles service. Copy the access token as it will be used in the next step.
-
In the Projects window, in the Sources folder, open the main.cpp file.
-
Modify the code to set the API key to the access token. Save and close the file.
main.cppUse dark colors for code blocks Change line // 2. API key authentication: Get a long-lived access token that gives your application access to // ArcGIS location services. Go to the tutorial at https://links.esri.com/create-an-api-key. // Copy the API Key access token. const QString accessToken = QString("");
-
Get the web scene item ID
You can use ArcGIS tools to create and view web scenes. Use the Scene Viewer to identify the web scene item ID. This item ID will be used later in the tutorial.
- Go to the LA Trails and Parks web scene in the Scene Viewer in ArcGIS Online. This web scene displays trails, trailheads and parks in the Santa Monica Mountains.
- Make a note of the item ID at the end of the browser's URL. The item ID should be 579f97b2f3b94d4a8e48a5f140a6639b
Remove unneeded code
A web scene can define all of the content created in this code, such as the basemap, elevation layer, and initial viewpoint. Accordingly, the following code needs to be removed or changed.
-
In Projects, double-click Sources > Display_a_scene.cpp to open the file.
-
Remove the unnecessary
#include
statements for:ArcGISTiled
,Elevation Source.h Camera.h
,Elevation
,Source List Model.h Map
, andTypes.h Surface.h
. Removing these lines of code will keep you from having compiler warnings.Display_a_scene.cppUse dark colors for code blocks Remove line Remove line Remove line Remove line Remove line Remove line Remove line #include "Display_a_scene.h" #include "ArcGISTiledElevationSource.h" #include "Camera.h" #include "ElevationSourceListModel.h" #include "MapTypes.h" #include "Scene.h" #include "SceneQuickView.h" #include "Surface.h" #include <QUrl>
-
Change the constructor to remove the 2nd argument. This means the full syntax for the current multi-line statement will become just
QObject(parent)
.Display_a_scene.cppUse dark colors for code blocks Change line Remove line Display_a_scene::Display_a_scene(QObject* parent /* = nullptr */): QObject(parent), m_scene(new Scene(BasemapStyle::ArcGISImagery, this))
-
Remove the following lines since you will not be using an
A
from ar GISTiled Elevation Source QUrl
and applying it to theScene
.Display_a_scene.cppUse dark colors for code blocks Remove line Remove line Remove line Remove line Remove line Remove line // create a new elevation source from Terrain3D rest service ArcGISTiledElevationSource* elevationSource = new ArcGISTiledElevationSource( QUrl("https://elevation3d.arcgis.com/arcgis/rest/services/WorldElevation3D/Terrain3D/ImageServer"), this); // add the elevation source to the scene to display elevation m_scene->baseSurface()->elevationSources()->append(elevationSource);
-
Remove the following lines to remove the
Camera
object and all of its arguments.Display_a_scene.cppUse dark colors for code blocks Remove line Remove line Remove line Remove line Remove line Remove line Remove line Remove line // Set the view (created in QML) void Display_a_scene::setSceneView(SceneQuickView* sceneView) { if (!sceneView || sceneView == m_sceneView) { return; } m_sceneView = sceneView; m_sceneView->setArcGISScene(m_scene); constexpr double latitude = 33.909; constexpr double longitude = -118.805; constexpr double altitude = 5330.0; constexpr double heading = 355.0; constexpr double pitch = 72.0; constexpr double roll = 0.0; const Camera sceneCamera(latitude, longitude, altitude, heading, pitch, roll); m_sceneView->setViewpointCameraAndWait(sceneCamera);
-
Remove all of the existing code from the
setup
method.Scene Display_a_scene.cppUse dark colors for code blocks Remove line Remove line Remove line Remove line Remove line void Display_a_scene::setupScene() { ArcGISTiledElevationSource* elevationSource = new ArcGISTiledElevationSource(QUrl("https://elevation3d.arcgis.com/arcgis/rest/services/WorldElevation3D/Terrain3D/ImageServer"), this); Surface* elevationSurface = new Surface(this); elevationSurface->elevationSources()->append(elevationSource); elevationSurface->setElevationExaggeration(2.5); m_scene->setBaseSurface(elevationSurface); }
Loading a scene from a web scene usually requires less code and makes it easier to update a scene used by several apps. Furthermore, web scenes can provide a more consistent experience for your user.
Display a web scene using its portal item URL
You can display a web scene using the web scene's item ID. Create a scene from the web scene portal item, and display it in your app.
-
Add code to the
setup
method to create the web scene portal URL. Concatenate the web scene's unique item ID and the URL for ArcGIS Online.Scene Display_a_scene.cppUse dark colors for code blocks Add line. Add line. Add line. Add line. Add line. void Display_a_scene::setupScene() { // Create an item_id to reference an item at a web server const QString item_id("579f97b2f3b94d4a8e48a5f140a6639b"); // Create a QUrl from the web scene server URL and the item_id const QUrl portal_url(QString("https://arcgis.com/sharing/rest/content/items/" + item_id));
-
Create the
Scene
using the web scene's URL.Display_a_scene.cppUse dark colors for code blocks Add line. Add line. void Display_a_scene::setupScene() { // Create an item_id to reference an item at a web server const QString item_id("579f97b2f3b94d4a8e48a5f140a6639b"); // Create a QUrl from the web scene server URL and the item_id const QUrl portal_url(QString("https://arcgis.com/sharing/rest/content/items/" + item_id)); // Create the scene, referencing the QUrl m_scene = new Scene(portal_url, this);
-
Press Ctrl + R to run the app.
Your app displays the scene that you viewed earlier in the Scene Viewer.
What's Next?
Learn how to use additional API features, ArcGIS location services, and ArcGIS tools in these tutorials: