Learn how to find a route and directions with the route service.
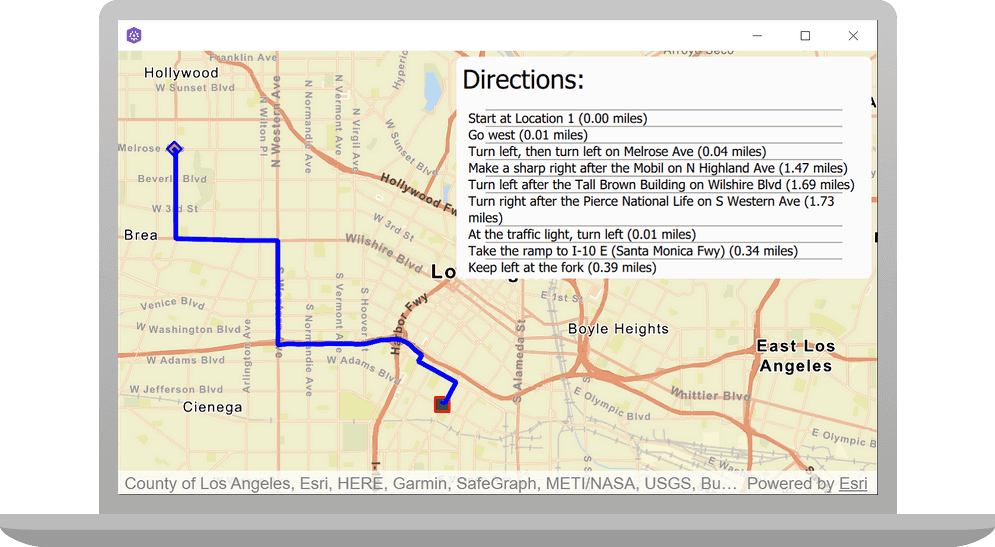
Routing is the process of finding the path from an origin to a destination in a street network. You can use the Routing service to find routes, get driving directions, calculate drive times, and solve complicated, multiple vehicle routing problems. To create a route, you typically define a set of stops (origin and one or more destinations) and use the service to find a route with directions. You can also use a number of additional parameters such as barriers and mode of travel to refine the results.
In this tutorial, you define an origin and destination by clicking on the map. These values are used to get a route and directions from the route service. The directions are also displayed on the map.
Prerequisites
The following are required for this tutorial:
- An ArcGIS account to access API keys. If you don't have an account, sign up for free.
- Your system meets the system requirements.
- The ArcGIS Maps SDK for Qt, version 200.4.0 or later is installed.
- The Qt 6.5.1 software development framework is installed.
Steps
Open the project in Qt Creator
-
To start this tutorial, complete the Display a map tutorial or download and unzip the solution.
-
Open the display_a_map project in Qt Creator.
-
If you downloaded the Display a map solution, set your API key.
An API Key enables access to services, web maps, and web scenes hosted in ArcGIS Online.
-
Go to your developer dashboard to get your API key. For these tutorials, use your default API key. It is scoped to include all of the services demonstrated in the tutorials.
-
In the Projects window, in the Sources folder, open the main.cpp file.
-
Modify the code to set the API key. Paste the API key, acquired from your dashboard, between the quotes. Then save and close the file.
main.cppUse dark colors for code blocks Change line // 2. API key authentication: Get a permanent key that gives your application access to Esri // location services. Create a new API key or access existing API keys from // your ArcGIS for Developers dashboard (https://links.esri.com/arcgis-api-keys). const QString apiKey = QString("");
-
Declare classes, functions, variables, enumerations and signals
-
In the display_a_map project, double click on Headers > Display_a_map.h to open the file. Add the four class declarations shown.
Display_a_map.hUse dark colors for code blocks Add line. Add line. Add line. Add line. namespace Esri::ArcGISRuntime { class Map; class MapQuickView; class Graphic; class GraphicsOverlay; class PictureMarkerSymbol; class RouteTask;
-
Create an enum to monitor user route selections and maintain the route builder status. This will be initialized in Display_a_map.cpp in a later step.
Display_a_map.hUse dark colors for code blocks Add line. Add line. Add line. Add line. Add line. Add line. class Graphic; class GraphicsOverlay; class PictureMarkerSymbol; class RouteTask; } enum RouteBuilderStatus { NotStarted, SelectedStart, SelectedStartAndEnd, };
-
Add these
#include
statements and a class declaration.Display_a_map.hUse dark colors for code blocks Add line. Add line. Add line. Add line. enum RouteBuilderStatus { NotStarted, SelectedStart, SelectedStartAndEnd, }; #include <QObject> #include "RouteParameters.h" class QAbstractListModel; Q_MOC_INCLUDE("QAbstractListModel") Q_MOC_INCLUDE("MapQuickView.h")
-
Use
Q_
to create a member variablePROPERTY m_
.directions Display_a_map.hUse dark colors for code blocks Add line. class Display_a_map : public QObject { Q_OBJECT Q_PROPERTY(Esri::ArcGISRuntime::MapQuickView* mapView READ mapView WRITE setMapView NOTIFY mapViewChanged) Q_PROPERTY(QAbstractListModel* directions MEMBER m_directions NOTIFY directionsChanged)
-
Add the following signal declaration; this will be used to prompt updates to route directions.
Display_a_map.hUse dark colors for code blocks Add line. public: explicit Display_a_map(QObject* parent = nullptr); ~Display_a_map() override; signals: void mapViewChanged(); void directionsChanged();
-
Declare the following private methods.
Display_a_map.hUse dark colors for code blocks Add line. Add line. Add line. private: Esri::ArcGISRuntime::MapQuickView* mapView() const; void setMapView(Esri::ArcGISRuntime::MapQuickView* mapView); void setupViewpoint(); void setupRouteTask(); void findRoute(); void resetState();
-
Declare and initialize the following pointers, object, and enumeration. Then save and close the file.
Display_a_map.hUse dark colors for code blocks Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. void setupRouteTask(); void findRoute(); void resetState(); Esri::ArcGISRuntime::Map* m_map = nullptr; Esri::ArcGISRuntime::MapQuickView* m_mapView = nullptr; Esri::ArcGISRuntime::GraphicsOverlay* m_graphicsOverlay = nullptr; Esri::ArcGISRuntime::RouteTask* m_routeTask = nullptr; Esri::ArcGISRuntime::Graphic* m_startGraphic = nullptr; Esri::ArcGISRuntime::Graphic* m_endGraphic = nullptr; Esri::ArcGISRuntime::Graphic* m_lineGraphic = nullptr; QAbstractListModel* m_directions = nullptr; Esri::ArcGISRuntime::RouteParameters m_routeParameters; RouteBuilderStatus m_currentState;
Include header files to access needed classes
-
In the display_a_map project, double click on Sources > Display_a_map.cpp to open the file. Add
#include
statements for the classes shown.Display_a_map.cppUse dark colors for code blocks Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. #include "Display_a_map.h" #include "Map.h" #include "MapTypes.h" #include "MapQuickView.h" #include "Point.h" #include "Viewpoint.h" #include "SpatialReference.h" #include <QFuture> #include "DirectionManeuverListModel.h" #include "Graphic.h" #include "GraphicListModel.h" #include "GraphicsOverlay.h" #include "GraphicsOverlayListModel.h" #include "Polyline.h" #include "RouteTask.h" #include "RouteResult.h" #include "RouteParameters.h" #include "Route.h" #include "SimpleLineSymbol.h" #include "SimpleMarkerSymbol.h" #include "Stop.h" #include "Symbol.h" #include "SymbolTypes.h" #include <QGeoPositionInfoSource> #include <QList> #include <QUrl> #include <QUuid>
Update the constructor
-
Update the constructor as shown. Set
BasemapStyle
toArcGISStreets
and initialize theRoute
enumeration.Builder Status Display_a_map.cppUse dark colors for code blocks Change line Add line. Display_a_map::Display_a_map(QObject* parent /* = nullptr */): QObject(parent), m_map(new Map(BasemapStyle::ArcGISStreets, this)), m_currentState(RouteBuilderStatus::NotStarted)
-
Call the
setup
method within the constructor. This will be populated in a later step.Route Task() Display_a_map.cppUse dark colors for code blocks Add line. Display_a_map::Display_a_map(QObject* parent /* = nullptr */): QObject(parent), m_map(new Map(BasemapStyle::ArcGISStreets, this)), m_currentState(RouteBuilderStatus::NotStarted) { setupRouteTask();
Change the map's view point
-
In
setup
change the map'sViewpoint() Point
andViewpoint
to place the map over over downtown Los Angeles.Display_a_map.cppUse dark colors for code blocks Change line Change line MapQuickView* Display_a_map::mapView() const { return m_mapView; } void Display_a_map::setupViewpoint() { const Point center(-118.24532, 34.05398, SpatialReference::wgs84()); const Viewpoint viewpoint(center, 144447.638572);
Change setupViewpoint() to respond to mouse clicks and find the route
-
Add a
connect
statement tosetup
to detect user mouse clicks, set and display point graphics, respond to the first and second click (using theViewpoint() switch
statement), and callfind
on the second mouse click.Route Display_a_map.cppUse dark colors for code blocks Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. void Display_a_map::setupViewpoint() { const Point center(-118.24532, 34.05398, SpatialReference::wgs84()); const Viewpoint viewpoint(center, 144447.638572); m_mapView->setViewpointAsync(viewpoint); connect(m_mapView, &MapQuickView::mouseClicked, this, [this](QMouseEvent& mouse) { const Point mapPoint = m_mapView->screenToLocation(mouse.position().x(), mouse.position().y()); switch (m_currentState) { case RouteBuilderStatus::NotStarted: resetState(); m_currentState = RouteBuilderStatus::SelectedStart; m_startGraphic->setGeometry(mapPoint); break; case RouteBuilderStatus::SelectedStart: m_currentState = RouteBuilderStatus::SelectedStartAndEnd; m_endGraphic->setGeometry(mapPoint); findRoute(); break; case RouteBuilderStatus::SelectedStartAndEnd: // Ignore touches while routing is in progress break; } });
Create route graphics
-
Add code to
setup
to create the route's starting pointViewpoint() Graphic
, determined by the user's first mouse click. This consists of aSimpleLineSymbol
, color blue, size 2, that outlines aSimple
, diamond shaped and orange in color. Create the route's ending pointMarker Symbol Graphic
, determined by the user's second mouse click. This consists of aSimpleLineSymbol
, color red, size 2, that outlines aSimple
, square shaped and green in color. Create a lineMarkersymbol Graphic
connecting the route's starting and ending points using aSimpleLineSymbol
, color blue, size 4. Then append the starting point graphic, ending point graphic, and route line graphic to aGraphicsOverlay
.Display_a_map.cppUse dark colors for code blocks Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. case RouteBuilderStatus::SelectedStartAndEnd: // Ignore touches while routing is in progress break; } }); m_graphicsOverlay = new GraphicsOverlay(this); m_mapView->graphicsOverlays()->append(m_graphicsOverlay); SimpleLineSymbol* startOutlineSymbol = new SimpleLineSymbol(SimpleLineSymbolStyle::Solid, QColor("blue"), 2/*width*/, this); SimpleMarkerSymbol* startSymbol = new SimpleMarkerSymbol(SimpleMarkerSymbolStyle::Diamond, QColor("orange"), 12/*width*/, this); startSymbol->setOutline(startOutlineSymbol); m_startGraphic = new Graphic(this); m_startGraphic->setSymbol(startSymbol); SimpleLineSymbol* endOutlineSymbol = new SimpleLineSymbol(SimpleLineSymbolStyle::Solid, QColor("red"), 2/*width*/, this); SimpleMarkerSymbol* endSymbol = new SimpleMarkerSymbol(SimpleMarkerSymbolStyle::Square, QColor("green"), 12/*width*/, this); endSymbol->setOutline(endOutlineSymbol); m_endGraphic = new Graphic(this); m_endGraphic->setSymbol(endSymbol); SimpleLineSymbol* lineSymbol = new SimpleLineSymbol(SimpleLineSymbolStyle::Solid, QColor("blue"), 4/*width*/, this); m_lineGraphic = new Graphic(this); m_lineGraphic->setSymbol(lineSymbol); m_graphicsOverlay->graphics()->append(QList<Graphic*> {m_startGraphic, m_endGraphic, m_lineGraphic}); }
Create the setRouteTask() method
A task makes a request to a service and returns the results. Use the Route
class to access a routing service. Create a Route
with a string URL to reference the routing service.
-
Begin implementing the
set
method. Point theRoute Task() Route
to an online service. Call the create default parameters async method obtain the route parameters.Task Display_a_map.cppUse dark colors for code blocks Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. void Display_a_map::setupRouteTask() { // create the route task pointing to an online service m_routeTask = new RouteTask(QUrl("https://route-api.arcgis.com/arcgis/rest/services/World/Route/NAServer/Route_World"), this); // Create the default parameters which will load the route task implicitly. m_routeTask->createDefaultParametersAsync().then(this,[this](const RouteParameters& routeParameters) { // Store the resulting route parameters. m_routeParameters = routeParameters; }); }
Create the findRoute() method
-
Begin implementing the
find
method. Add code to first confirm thatRoute() RouteTask
has loaded andRouteParameters
is not empty. Then setRouteParameters
to return directions, and clear stops from previous routes. Then createStop
objects for the route from the geometries of the start and end graphics, and pass those toRouteParameters
. Callsolve
, passing inRoute Async() RouteParameters
. With the route completed, set the route graphic's geometry and resetRoute
to prepare for a new route task. Then display the route directions.Builder Status Display_a_map.cppUse dark colors for code blocks Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. void Display_a_map::findRoute() { if (m_routeTask->loadStatus() != LoadStatus::Loaded || m_routeParameters.isEmpty()) return; // Set parameters to return directions. m_routeParameters.setReturnDirections(true); // Clear previous stops from the parameters. m_routeParameters.clearStops(); // Set the stops to the parameters. const Stop stop1(Point(m_startGraphic->geometry())); const Stop stop2(Point(m_endGraphic->geometry())); m_routeParameters.setStops(QList<Stop> { stop1, stop2 }); // Solve the route with the parameters. m_routeTask->solveRouteAsync(m_routeParameters).then(this,[this](const RouteResult& routeResult) { // Add the route graphic once the solve completes. const Route generatedRoute = routeResult.routes().at(0); m_lineGraphic->setGeometry(generatedRoute.routeGeometry()); m_currentState = RouteBuilderStatus::NotStarted; // Set the direction maneuver list model. m_directions = generatedRoute.directionManeuvers(this); emit directionsChanged(); }); }
Create the resetState() method
This method resets all graphics and directions, and the Route
enumeration. This happens at the beginning of every new route task.
-
Reset all graphics with empty
Point
objects, setm_
todirections nullptr
, and resetRoute
. Then save the file.Builder Status Display_a_map.cppUse dark colors for code blocks Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. void Display_a_map::resetState() { m_startGraphic->setGeometry(Point()); m_endGraphic->setGeometry(Point()); m_lineGraphic->setGeometry(Point()); m_directions = nullptr; m_currentState = RouteBuilderStatus::NotStarted; }
Create the GUI
-
In the display_a_map project, double click on Resources > qml\qml.qrc > /qml > Display_a_mapForm.qml to open the file. Add the following import.
display_a_mapForm.qmlUse dark colors for code blocks Add line. import QtQuick import QtQuick.Controls import Esri.Display_a_map import QtQuick.Shapes
-
Add the code highlighted below. This builds out the application GUI and displays the route and route directions.
display_a_mapForm.qmlUse dark colors for code blocks Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. // Declare the C++ instance which creates the map etc. and supply the view. Display_a_map { id: model mapView: view } // Create window for displaying the route directions. Rectangle { id: directionWindow anchors { right: parent.right top: parent.top margins: 5 } radius: 5 visible: model.directions width: Qt.platform.os === "ios" || Qt.platform.os === "android" ? 250 : 350 height: parent.height / 2 color: "#FBFBFB" clip: true ListView { id: directionsView anchors { fill: parent margins: 5 } header: Component { Text { height: 40 text: "Directions:" font.pixelSize: 22 } } // Set the model to the DirectionManeuverListModel returned from the route. model: model.directions delegate: directionDelegate } } Component { id: directionDelegate Rectangle { id: rect width: parent.width height: textDirections.height color: directionWindow.color // separator for directions Shape { height: 2 ShapePath { strokeWidth: 1 strokeColor: "darkgrey" strokeStyle: ShapePath.SolidLine startX: 20; startY: 0 PathLine { x: parent.width - 20 ; y: 0 } } } Text { id: textDirections text: qsTr("%1 (%2 miles)".arg(directionText).arg((length * 0.00062137).toFixed(2))) wrapMode: Text.WordWrap anchors { leftMargin: 5 left: parent.left right: parent.right } } } }
-
Press Ctrl + R to run the app.
The map should support two clicks to create an origin and destination point and then use the route service to display the resulting route and turn-by-turn directions.
What's next?
Learn how to use additional API features, ArcGIS location services, and ArcGIS tools in these tutorials: