Learn how to display point, line, and polygon graphics in a map.
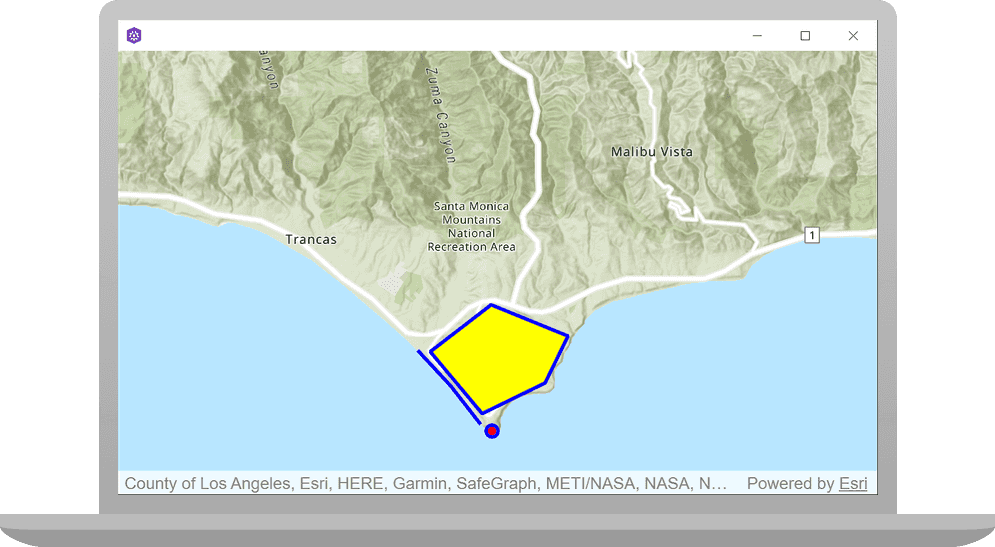
You typically use graphics to display geographic data that is not connected to a database and that is not persisted, like highlighting a route between two locations, displaying a search buffer around a point, or tracking the location of a vehicle in real-time. Graphics are composed of a geometry, symbol, and attributes.
In this tutorial, you display points, lines, and polygons on a map as graphics.
Prerequisites
Before starting this tutorial:
-
You need an ArcGIS Location Platform or ArcGIS Online account.
-
Your system meets the system requirements.
-
The ArcGIS Maps SDK for Qt, version 200.4.0 or later is installed.
-
The Qt 6.5.1 software development framework is installed.
Open the project in Qt Creator
-
To start this tutorial, complete the Display a map tutorial or download and unzip the solution.
-
Open the display_a_map project in Qt Creator.
-
If you downloaded the solution, get an access token and set the API key.
An API Key gives your app access to secure resources used in this tutorial.
-
Go to the Create an API key tutorial to obtain a new API key access token. Ensure that the following privilege is enabled: Location services > Basemaps > Basemap styles service. Copy the access token as it will be used in the next step.
-
In the Projects window, in the Sources folder, open the main.cpp file.
-
Modify the code to set the API key to the copied access token. Save and close the file.
main.cppUse dark colors for code blocks Change line // 2. API key authentication: Get a long-lived access token that gives your application access to // ArcGIS location services. Go to the tutorial at https://links.esri.com/create-an-api-key. // Copy the API Key access token. const QString accessToken = QString("");
-
Add GraphicsOverlay class, declare member function
GraphicsOverlay
is a container for temporary graphics to display on your map view. The graphics drawn in graphics overlays are created at runtime and are not persisted when your application closes. Learn more about GraphicsOverlay
.
-
In the display_a_map project, double click Headers > Display_a_map.h to open the file. Add the
Graphics
class to theOverlay namespace ArcGISRuntime
declaration.Display_a_map.hUse dark colors for code blocks Add line. Add line. class Map; class MapQuickView; class GraphicsOverlay; }
-
Add the
create
private member function declaration. Then save and close the header file.Graphics Display_a_map.hUse dark colors for code blocks Add line. private: Esri::ArcGISRuntime::MapQuickView* mapView() const; void setMapView(Esri::ArcGISRuntime::MapQuickView* mapView); void setupViewpoint(); void createGraphics(Esri::ArcGISRuntime::GraphicsOverlay* overlay);
Create a graphics overlay
A graphics overlay is a container for graphics. It is added to a map view to display graphics on a map. You can add more than one graphics overlay to a map view. Graphics overlays are displayed on top of all the other layers.
-
Double click on Sources > Display_a_map.cpp to open the file. Include the classes shown.
Display_a_map.cppUse dark colors for code blocks Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. #include "Display_a_map.h" #include "Map.h" #include "MapQuickView.h" #include "MapTypes.h" #include "Point.h" #include "SpatialReference.h" #include <QFuture> #include "Viewpoint.h" #include "Graphic.h" #include "GraphicListModel.h" #include "GraphicsOverlay.h" #include "GraphicsOverlayListModel.h" #include "PolylineBuilder.h" #include "PolygonBuilder.h" #include "SimpleFillSymbol.h" #include "SimpleLineSymbol.h" #include "SimpleMarkerSymbol.h" #include "SymbolTypes.h"
-
In the
Display_
member function, add three lines of code to create aa_ map: :set M a p View GraphicsOverlay
, call thecreate
method (implemented in following steps), and append the overlay to the map view.Graphics Display_a_map.cppUse dark colors for code blocks Add line. Add line. Add line. // Set the view (created in QML) void Display_a_map::setMapView(MapQuickView* mapView) { if (!mapView || mapView == m_mapView) { return; } m_mapView = mapView; m_mapView->setMap(m_map); setupViewpoint(); GraphicsOverlay* overlay = new GraphicsOverlay(this); createGraphics(overlay); m_mapView->graphicsOverlays()->append(overlay);
-
Create a new method named
Display_
, right after thea_ map: :create Graphics Display_
method.a_ map: :setup Viewpoint Display_a_map.cppUse dark colors for code blocks Add line. Add line. Add line. Add line. void Display_a_map::setupViewpoint() { const Point center(-118.80543, 34.02700, SpatialReference::wgs84()); const Viewpoint viewpoint(center, 100000.0); m_mapView->setViewpointAsync(viewpoint); } void Display_a_map::createGraphics(GraphicsOverlay *overlay) { }
Add a point graphic
A point graphic is created using a point and a marker symbol. A point is defined with x and y coordinates for longitude and latitude coordinates, and a spatial reference. The spatial reference is WGS84.
-
Create a
Point
and aSimpleMarkerSymbol
. To create thePoint
, provide longitude (x) and latitude (y) coordinates, and aSpatialReference
.Point graphics support a number of symbol types such as
SimpleMarkerSymbol
, PictureMarkerSymbol_qt andTextSymbol
. SeeSymbol
in the API documentation to learn more about symbols.Display_a_map.cppUse dark colors for code blocks Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. void Display_a_map::setupViewpoint() { const Point center(-118.80543, 34.02700, SpatialReference::wgs84()); const Viewpoint viewpoint(center, 100000.0); m_mapView->setViewpointAsync(viewpoint); } void Display_a_map::createGraphics(GraphicsOverlay *overlay) { // Create a point const Point dume_beach(-118.80657463861, 34.0005930608889, SpatialReference::wgs84()); // Create symbols for the point SimpleLineSymbol* point_outline = new SimpleLineSymbol(SimpleLineSymbolStyle::Solid, QColor("blue"), 3, this); SimpleMarkerSymbol* point_symbol = new SimpleMarkerSymbol(SimpleMarkerSymbolStyle::Circle, QColor("red"), 10, this); point_symbol->setOutline(point_outline); // Create a graphic to display the point with its symbology Graphic* point_graphic = new Graphic(dume_beach, point_symbol, this); // Add point graphic to the graphics overlay overlay->graphics()->append(point_graphic);
-
Press Ctrl + R to run the app.
You should see a point graphic at Point Dume State Beach, California.
Add a polyline graphic
A line graphic is created using a polyline and a line symbol. A polyline is defined as a sequence of points.
Polylines have one or more distinct parts. Each part is defined by two points. To create a continuous line with just one part, use the Polyline
constructor. To create a polyline with more than one part, use a PolylineBuilder
. Polyline graphics support a number of symbol types, such as SimpleLineSymbol
and TextSymbol
. See Symbol
in the API documentation to learn more about symbols.
-
Create a
Polyline
and aSimpleLineSymbol
.To create the
Polyline
, create a newPointCollection
with aSpatialReference
and usePolylineBuilder
to add a newPoint
objects to it. Add the highlighted code.Display_a_map.cppUse dark colors for code blocks Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. // Create a graphic to display the point with its symbology Graphic* point_graphic = new Graphic(dume_beach, point_symbol, this); // Add point graphic to the graphics overlay overlay->graphics()->append(point_graphic); // Create a line PolylineBuilder* polyline_builder = new PolylineBuilder(SpatialReference::wgs84(), this); polyline_builder->addPoint(-118.8215, 34.0140); polyline_builder->addPoint(-118.8149, 34.0081); polyline_builder->addPoint(-118.8089, 34.0017); // Create a symbol for the line SimpleLineSymbol* line_symbol = new SimpleLineSymbol(SimpleLineSymbolStyle::Solid, QColor(Qt::blue), 3, this); // Create a graphic to display the line with its symbology Graphic* polyline_graphic = new Graphic(polyline_builder->toGeometry(), line_symbol, this); // Add line graphic to the graphics overlay overlay->graphics()->append(polyline_graphic);
-
Press Ctrl + R to run the app.
You should see a point and a line graphic along Westward Beach.
Add a polygon graphic
A polygon graphic is created using a polygon and a fill symbol. A polygon is defined as a sequence of points that describe a closed boundary.
Polygons have one or more distinct parts. Each part is a sequence of points describing a closed boundary. For a single area with no holes, you can use Polygon
to create a polygon with just one part. To create a polygon with more than one part, use PolygonBuilder
.
Polygon graphics support a number of symbol types such as SimpleFillSymbol
, PictureFillSymbol
, SimpleMarkerSymbol
, and TextSymbol
. See Symbol
in the API documentation to learn more about symbols.
-
Create a
Polygon
and aSimpleFillSymbol
. To create thePolygon
, create a newPointCollection
with aSpatialReference
and usePolygonBuilder
to add newPoint
objects to it. Add the highlighted code.Display_a_map.cppUse dark colors for code blocks Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. Add line. // Create a graphic to display the line with its symbology Graphic* polyline_graphic = new Graphic(polyline_builder->toGeometry(), line_symbol, this); // Add line graphic to the graphics overlay overlay->graphics()->append(polyline_graphic); // Create a list of points to make up the polygon const QList<Point> points = { Point(-118.8190, 34.0138), Point(-118.8068, 34.0216), Point(-118.7914, 34.0164), Point(-118.7960, 34.0086), Point(-118.8086, 34.0035), }; // Create a polygon using the list of points above PolygonBuilder* polygon_builder = new PolygonBuilder(SpatialReference::wgs84(), this); polygon_builder->addPoints(points); // Create symbols for the polygon SimpleLineSymbol* polygon_line_symbol = new SimpleLineSymbol(SimpleLineSymbolStyle::Solid, QColor(Qt::blue), 3, this); SimpleFillSymbol* fill_symbol = new SimpleFillSymbol(SimpleFillSymbolStyle::Solid, QColor(Qt::yellow), polygon_line_symbol, this); // Create a graphic to display the polygon with its symbology Graphic* polygon_graphic = new Graphic(polygon_builder->toGeometry(), fill_symbol, this); // Add polygon graphic to the graphics overlay overlay->graphics()->append(polygon_graphic);
-
Press Ctrl + R to run the app.
You should see a point, line, and polygon graphic around Mahou Riviera in the Santa Monica Mountains.
What's next?
Learn how to use additional API features, ArcGIS location services, and ArcGIS tools in these tutorials: