Electronic navigational chart (ENC) datasets are georeferenced vector datasets for the visualization and analysis of hydrographic and maritime information. This SDK supports ENC datasets conforming to the International Hydrographic Organization (IHO) standard S-57 (Transfer Standard for Digital Hydrographic Data) and encrypted ENC datasets conforming to the standard S-63 (IHO Data Protection Scheme).
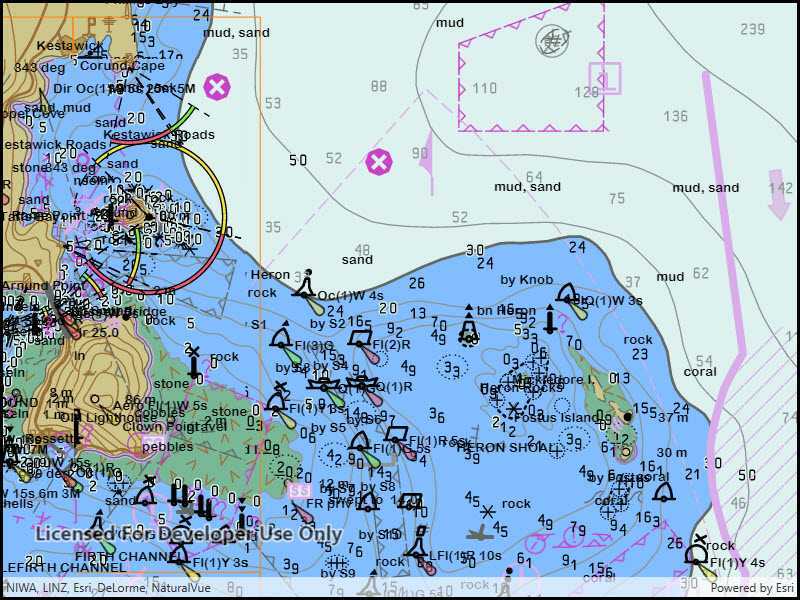
Use ENCs in your apps to:
-
Visualize S-57 data in compliance with S-52 standards and specifications for chart content display.
-
Use S-57 data as an additional data source for situational awareness and decision making.
-
Combine S-57 datasets with other sources of information for geospatial analysis.
-
Access ENCs encrypted conforming to the S-63 IHO Data Protection Scheme.
Get started
ENC functionality is accessed via the Esri.
NuGet package. The NuGet package will ensure that all binaries and resources for ENC functionality are deployed to your application’s output folder.
Understand ENCs
An ENC cell represents a navigational chart of a rectangular geographic area at a particular scale. Cells are bounded by meridians and parallels, though the actual area of coverage contained in the cell may be any shape. All the data in a single cell corresponds to a single navigational use, such as: overview, general, or coastal (a navigational use corresponds to a scale range suitable for a particular use).
Cells are stored in a single base dataset file and zero or more update dataset files. Each dataset file has a unique name. A base dataset file plus its update files (if any) when loaded together comprise the updated geographic data of one cell.
ENC data is distributed in exchange sets, which can contain many cells. On the file system, each exchange set resides in its own folder named ENC_ROOT. Each ENC_ROOT folder contains a collection of dataset files (*.000), update files (.001-.999), a catalog file containing metadata about the exchange set (CATALOG.031), and other optional files with data referenced by the exchange set, such as text and image files.
See S-57 Appendix B for additional details about S-57 ENC exchange sets and their content.
Display an ENC
Access an exchange set on the file system using an EncExchangeSet
object. An exchange set can be loaded using the path to the exchange set CATALOG.031 file, and optionally the paths to CATALOG.031 files for other exchange sets (in other directories) that contain additional update dataset files. If one of the datasets specified is an exchange set that contains only updates, then the exchange set with the corresponding base dataset files must be loaded in the same API call. See the Work with updates section for more information.
EncDataset
objects represent datasets. Each EncDataset
contains the base data for a cell and any updates that were also loaded when the exchange set was loaded, and provides access to metadata about the cell. Retrieve a collection of EncDataset
objects contained in an EncExchangeSet
using the Datasets
property.
ENC cells (individual charts) are represented by EncCell
objects. You can construct these objects in two ways:
- From a dataset represented by an
EncDataset
object. This approach will include the base dataset file and all corresponding update dataset files. This is the preferred approach. - From a base dataset file. This approach will only include the base dataset file, and not any corresponding update dataset files. This is not a typical use case.
Display an ENC cell by constructing an EncLayer
object from an EncCell
object. EncLayer
is derived from Layer
, so you add an EncLayer
to a map like you do other layers.
You can add all the charts in the exchange set by iterating through the exchange set's datasets, creating an EncCell
for each, creating an EncLayer
for each EncCell
, and adding each EncLayer
to the map.
EncExchangeSet encExchangeSet = new EncExchangeSet(encPath);
await encExchangeSet.LoadAsync();
List<Envelope> dataSetExtents = new List<Envelope>();
foreach (EncDataset encDataset in encExchangeSet.Datasets)
{
EncLayer encLayer = new EncLayer(new EncCell(encDataset));
await encLayer.LoadAsync();
dataSetExtents.Add(encLayer.FullExtent);
MyMapView.Map.OperationalLayers.Add(encLayer);
}
// Set the map viewpoint with the full extent of the ENC Exchange Set.
Envelope fullExtent = GeometryEngine.CombineExtents(dataSetExtents);
await MyMapView.SetViewpointAsync(new Viewpoint(fullExtent));
Work with ENC updates
ENC charts are often distributed as base cells (.000 files) with one or more update cells (.001, .002, etc. files). An exchange set can consist exclusively of update cells; in order to load an update exchange set, the path to the base exchange set must be provided along with the path to the update exchange set.
EncExchangeSet exchangeSet = new EncExchangeSet(new string[] { @"path\to\update", @"path\to\original" });
When loading an ENC cell, it is important to use the dataset-based constructor. If updates for a cell are part of an exchange set, they will be found only when the dataset-based constructor is used. Loading the cell from a path will not load any associated updates.
Set ENC environment settings
ENC layers are displayed in accordance with the IHO standard S-52 (Specifications for Chart Content and Display Aspects of ECDIS). You can define the display properties of your ENC layers by using the static EncEnvironmentSettings
class. These settings apply to all ENC layers in all maps. Settings fall under three categories: mariner settings, text group visibility settings, and viewing group settings. Text group settings control the display of labels for features, mariner settings control the symbolization and presentation of ENC features, and viewing group settings allow for quickly applying settings to logical groups of feature types.
// Enables display of seabed information for all ENC layers
EncEnvironmentSettings.Default.DisplaySettings.TextGroupVisibilitySettings.NatureOfSeabed = true;
ENC content is accessed via compiled SENC (System Electronic Navigational Chart) files. After an ENC cell has been loaded, all future loads of that cell will reference the underlying SENC file directly. You can use the environment settings to find or set the location where SENC files are stored.
Identify and select ENC features
ENC layers support identify through the common geoview feature identification mechanism, which takes a screen point and tolerance in pixels. Identify will return a collection of result objects, one per matching layer. For ENC layers, the results will have a Geo
property, which provides a collection of EncFeature
objects.
Once a feature has been identified, you can call Select
on the layer that contains the feature to select it.
// Perform the identify operation
IReadOnlyList<IdentifyLayerResult> results = await MyMapView.IdentifyLayersAsync(e.Position, 5, false);
// Return if there are no results
if (results.Count < 1) { return; }
// Take action on each identified ENC feature
foreach (IdentifyLayerResult result in results)
{
foreach (EncFeature feature in result.GeoElements)
{
// Get the layer associated with this set of results
EncLayer containingLayer = (EncLayer)result.LayerContent;
// Select the feature
containingLayer.SelectFeature(feature);
}
}
Access encrypted ENCs
You can use encrypted ENC datasets conforming to the S-63 standard (IHO Data Protection Scheme) on all platforms supported by this SDK: Windows, Android, iOS, Mac Catalyst, UWP, and Windows on ARM64. You are responsible for creating applications that are consistent with the S-63 specification and in compliance with the requirements set by the International Hydrographic Organization (IHO).
When working with encrypted ENC datasets:
- Your organization must enter an OEM (Original Equipment Manufacturer) agreement with the IHO in order to create an application that displays, processes, and operates on protected ENC datasets and information.
- Your organization is responsible for assigning unique hardware IDs to devices that use encrypted ENC datasets.
- A cell permit provided by the S-63 data server allows decryption of a specific cell on a specific device. A single file named PERMIT.TXT contains the cell permit. This file may multiple cell permits, one for each cell covered by the exchange set.
- The API may raise errors and warnings when operating on encrypted ENC datasets. You are responsible for checking for errors and warnings raised, and for handling the error or displaying to the user if required.
To access and work with encrypted ENC datasets:
- Ensure your app is licensed at the appropriate level. See the License levels and capabilities topic for more information about licensing requirements for working with ENC data.
- Set the hardware ID for the device.
- Set the certificate path.
- Ensure the SERIAL.ENC file (provided by the data server) is located in the same directory that contains the ENC_ROOT directory.
- Manage cell permits.
- Display ENC datasets in the map.
- Handle S-63 errors and warnings.
Set the hardware identifier
You are responsible for assigning unique hardware identifiers (HW_ID) to devices that will access encrypted ENC datasets. Hardware identifiers are unique values assigned by an OEM to each implementation of their system on a specific device or machine. Use the S63
method to apply the hardware identifier, that the API will use to decrypt S-63 ENCs. The hardware identifier must be set before attempting to access S-63 content.
S63EnvironmentSettings.SetHardwareId("FFFFF");
Set the certificate path
To authenticate and decrypt encrypted ENC datasets, you need the IHO Scheme Administrator (SA) Certificate. This SA certificate contains the public S-63 encryption key used by data servers to sign their S-63 data. Use the S63
method to define the path that contains the required digital certificate(s). The certificate path must be set before attempting to access S-63 content.
S63EnvironmentSettings.CertificatePath = @"..\..\IHO.CRT";
Manage cell permits
Cell permits are required for opening encrypted ENC datasets and are provided by the Data Server in a file called PERMIT.TXT. The API will validate that ENC cells are permitted for use by the specific device (according to the hardware ID set). The PERMIT.TXT file must be placed in the same directory that contains the CATALOG.031 file (typically the ENC_ROOT directory). There is no API provided for setting the location of the PERMIT.TXT file.
When opening encrypted ENC datasets there are permit-related warnings and errors that may be raised. See Handling S-63 warnings and errors for more information.
Handle S-63 errors and warnings
The API will check for various error conditions when opening encrypted ENC datasets. It is your responsibility to check for any errors and warnings and to handle those according to the S-63 specification. You can check for S-63 load warnings after attempting to load a cell or exchange set.
// Load the ENC exchange set
EncExchangeSet exchangeSet = new EncExchangeSet(encPath);
await exchangeSet.LoadAsync();
// Check the load warnings
foreach(var warning in exchangeSet.LoadWarnings)
{
// TODO - check for errors in table below and handle per S-63 spec
Debug.WriteLine($"S-63 error: {warning}");
}
The following table lists S-63 errors that may be reported by the API:
Runtime Code | SSE code | Description |
---|---|---|
11001 | SSE01 | Self Signed Key is invalid. |
11002 | SSE02 | Format of Self Signed Key file is incorrect. |
11003 | SSE03 | SA Signed Data Server Certificate is invalid. |
11004 | SSE04 | Format of SA Signed DS Certificate is incorrect. |
11005 | SSE05 | SA Digital Certificate (X509) file is not available. A valid certificate can be obtained from the IHO website or your data supplier. |
11006 | SSE06 | The SA Signed Data Server Certificate is invalid. The SA may have issued a new public key or the ENC may originate from another service. A new SA public key can be obtained from the IHO website or from your data supplier. |
11007 | SSE07 | SA signed DS Certificate file is not available. A valid certificate can be obtained from the IHO website or your data supplier. |
11008 | SSE08 | SA Digital Certificate (X509) file incorrect format. A valid certificate can be obtained from the IHO website or your data supplier. |
11009 | SSE09 | ENC Signature is invalid. |
11010 | SSE10 | Permits not available for this Data Server. Contact your data supplier to obtain the correct permits. |
11011 | SSE11 | Cell Permit not found. Load the permit file provided by the data supplier. |
11012 | SSE12 | Cell Permit format is incorrect. Contact your data supplier and obtain a new permit file. |
11013 | SSE13 | Cell Permit is invalid (checksum is incorrect) or the Cell Permit is for a different system. Contact your data supplier and obtain a new or valid permit file. |
11014 | SSE14 | Incorrect system date, check that the computer clock (if accessible) is set correctly or contact your system supplier. |
11015 | SSE15 | Subscription service has expired. Please contact your data supplier to renew the subscription license. |
11016 | SSE16 | ENC CRC value is incorrect. Contact your data supplier as ENC(s) may be corrupted or missing data. |
11017 | SSE17 | Userpermit is invalid (checksum is incorrect). Check that the correct hardware device (dongle) is connected or contact your system supplier to obtain a valid userpermit. |
11018 | SSE18 | HW_ID is incorrect format. |
11019 | SSE19 | Permits are not valid for this system. Contact your data supplier to obtain the correct permits. |
11020 | SSE20 | Subscription service will expire in less than 30 days. Please contact your data supplier to renew the subscription license. |
11021 | SSE21 | Decryption failed no valid cell permit found. Permits may be for another system or new permits may be required, please contact your supplier to obtain a new license. |
11022 | SSE22 | SA Digital Certificate (X509) has expired. A new SA public key can be obtained from the IHO website or from your data supplier. |
11023 | SSE23 | Non sequential update, previous update(s) missing - try reloading from the base media. If the problem persists contact your data supplier. |
11024 | SSE24 | ENC Signature format incorrect, contact your data supplier. |
11025 | SSE25 | The permit for ENC<cell name> has expired. This cell may be out of date and MUST NOT be used for Primary NAVIGATION. |
11026 | SSE26 | This ENC is not authenticated by the IHO acting as the Scheme Administrator. |
Performance considerations
ENC content is accesed via internal SENC files. When an ENC cell is loaded, an SENC representation is generated. Subsequent loads only read the generated SENC files. When developing apps for working with ENC content, understand that:
-
The SENC data path must be set before attempting to read ENC content.
- On .NET platforms, this is set to a temporary directory by default. The default directory may not be suitable for production use in the context of implementing an Electronic Chart Display and Information System (ECDIS).
-
SENC files are device and version specific. These files should not be exposed to users, backed up, or shared between devices. The SENC format is internal and may change between versions of ArcGIS Maps SDK for .NET. Therefore, SENC files created by an app built with one version of the SDK are not guaranteed to work with apps built with another version of the SDK, even on the same device.
- On iOS, consider setting the SENC path to a subdirectory of the documents directory that is excluded from iCloud and iTunes backup. See Apple Technical QA QA1719 for more information about excluding iOS directories from backup.
- See the Android Developers topic for more information about excluding directories from backup on Android.
-
SENC files take time to generate, this will delay the loading of new ENC cells. It may take a long time to load large ENC exchange sets consisting of many cells (potentially hours). Never block the UI when loading these files.
-
Because the initial load of an ENC cell (before the SENC files have been generated) can take some time to complete, consider pre-loading them to ensure availability before depending on them for navigation.
-
Do not attempt to read or manipulate SENC files. Changes to generated SENC files invalidate them. This requires the files to be re-generated the next time the corresponding cells are loaded.