Learn how to apply renderers and label definitions to a feature layer based on attribute values.
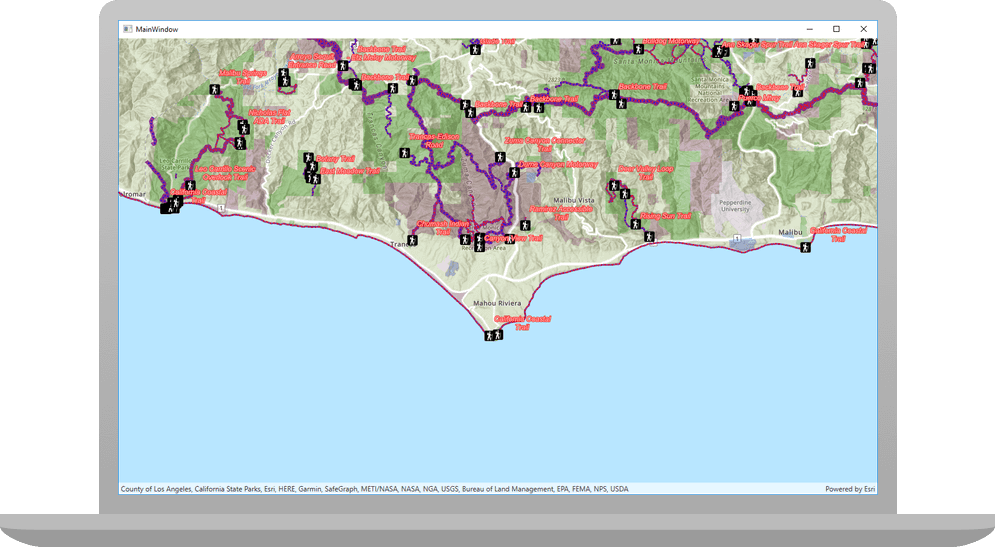
Applications can display feature layer data with different styles to enhance the visualization. The type of Renderer
you choose depends on your application. A SimpleRenderer
applies the same symbol to all features, a UniqueValueRenderer
applies a different symbol to each unique attribute value, and a ClassBreaksRenderer
applies a symbol to a range of numeric values. Renderers are responsible for accessing the data and applying the appropriate symbol to each feature when the layer draws. You can also use a LabelDefinition
to show attribute information for features. Visit the Styles and data visualization documentation to learn more about styling layers.
You can also author, style and save web maps, web scenes, and layers as portal items and then add them to the map in your application. Visit the following tutorials to learn more about adding portal items.
In this tutorial, you will apply different renderers to enhance the visualization of three feature layers with data for the Santa Monica Mountains: Trailheads with a single symbol, Trails based on elevation change and bike use, and Parks and Open Spaces based on the type of park.
Prerequisites
Before starting this tutorial:
-
You need an ArcGIS Location Platform or ArcGIS Online account.
-
Ensure your development environment meets the system requirements.
Optionally, you may want to install the ArcGIS Maps SDK for .NET to get access to project templates in Visual Studio (Windows only) and offline copies of the NuGet packages.
Steps
Open a Visual Studio solution
-
To start the tutorial, complete the Display a map tutorial or download and unzip the solution.
-
Open the
.sln
file in Visual Studio. -
If you downloaded the solution, get an access token and set the API key.
An API Key gives your app access to secure resources used in this tutorial.
-
Go to the Create an API key tutorial to obtain a new API key access token. Ensure that the following privilege is enabled: Location services > Basemaps > Basemap styles service. Copy the access token as it will be used in the next step.
-
In Visual Studio, in the Solution Explorer, click App.xaml.cs.
-
In the App class, add an override for the
O
function to set then Startup() Api
property onKey ArcGISRuntimeEnvironment
.App.xaml.csUse dark colors for code blocks public partial class App : Application { protected override void OnStartup(StartupEventArgs e) { base.OnStartup(e); Esri.ArcGISRuntime.ArcGISRuntimeEnvironment.ApiKey = "YOUR_ACCESS_TOKEN"; } } }
-
If you are developing with Visual Studio for Windows, ArcGIS Maps SDK for .NET provides a set of project templates for each supported .NET platform. These templates follow the Model-View-ViewModel (MVVM) design pattern. Install the ArcGIS Maps SDK for .NET Visual Studio Extension to add the templates to Visual Studio (Windows only). See Install and set up for details.
Update the tutorial name used in the project (optional)
The Visual Studio solution, project, and the namespace for all classes currently use the name Display
. Follow the steps below if you prefer the name to reflect the current tutorial. These steps are not required, your code will still work if you keep the original name.
The tutorial instructions and code use the name Style
for the solution, project, and namespace. You can choose any name you like, but it should be the same for each of these.
-
Update the name for the solution and the project.
- In Visual Studio, in the Solution Explorer, right-click the solution name and choose Rename. Provide the new name for your solution.
- In the Solution Explorer, right-click the project name and choose Rename. Provide the new name for your project.
-
Rename the namespace used by classes in the project.
- In the Solution Explorer, expand the project node.
- Double-click MapViewModel.cs in the Solution Explorer to open the file.
- In the
Map
class, double-click the namespace name (View Model Display
) to select it, and then right-click and choose Rename....AMap - Provide the new name for the namespace.
- Click Apply in the Rename: DisplayAMap window that appears in the upper-right of the code window. This will rename the namespace throughout your project.
-
Build the project.
- Choose Build > Build solution (or press <F6>).
Steps
Create a function to add a feature layer
A feature layer can be added from a feature service hosted in ArcGIS. Each feature layer contains features with a single geometry type (point, line, or polygon), and a set of attributes. Once added to the map, feature layers can be symbolized, styled, and labeled in a variety of ways.
You will define variables to store feature service URLs used by the app's layers and then create a helper method to add a layer to the map's collection of operational layers. You will use this code throughout the tutorial as you add and symbolize various layers.
-
In the Visual Studio > Solution Explorer, double-click MapViewModel.cs to open the file.
The project uses the Model-View-ViewModel (MVVM) design pattern to separate the application logic (view model) from the user interface (view).
Map
contains the view model class for the application, calledView Model.cs Map
. See the Microsoft documentation for more information about the Model-View-ViewModel pattern.View Model -
Add additional required
using
statements near the top of the .cs file. Using statements make your code more concise by allowing you to use classes from these namespaces without having to fully qualify them.MapViewModel.csUse dark colors for code blocks using System; using System.Collections.Generic; using System.Text; using Esri.ArcGISRuntime.Geometry; using Esri.ArcGISRuntime.Mapping; using System.ComponentModel; using System.Runtime.CompilerServices; using Esri.ArcGISRuntime.Data; using Esri.ArcGISRuntime.Mapping.Labeling; using Esri.ArcGISRuntime.Symbology; using System.Diagnostics; using System.Drawing;
-
Create four static
URI
s: three for accessing feature layers, and a fourth for accessing a static image for use in a picture marker symbol. You will use these resources in future steps.MapViewModel.csUse dark colors for code blocks class MapViewModel : INotifyPropertyChanged { // Application constants used to connect to data and resources. static Uri parksAndOpenSpaces = new Uri("https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Parks_and_Open_Space/FeatureServer/0"); static Uri trails = new Uri("https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Trails/FeatureServer/0"); static Uri trailheads = new Uri("https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Trailheads/FeatureServer/0"); static Uri trailheadImage = new Uri("https://static.arcgis.com/images/Symbols/NPS/npsPictograph_0231b.png");
-
Add a new method named
Add
that takes a feature service URI as an argument, creates a feature layer from it, and adds the layer to the map.Feature Layer() MapViewModel.csUse dark colors for code blocks private Map? _map; public Map? Map { get { return _map; } set { _map = value; OnPropertyChanged(); } } private FeatureLayer AddFeatureLayer(Uri featureServiceUri) { // Create a service feature table from a Uri. ServiceFeatureTable serviceFeatureTable = new ServiceFeatureTable(featureServiceUri); // Create a feature layer from the service feature table. FeatureLayer featureLayer = new FeatureLayer(serviceFeatureTable); // Add the feature layer to the maps operational layers collection. Map?.OperationalLayers.Add(featureLayer); // Return the feature layer. return featureLayer; }
Add a layer with a unique value renderer
Create a method to apply a different symbol for each type of park area to the Parks and Open Spaces feature layer.
-
Add a new method named
Add
just after the newly addedOpen Space Layer() Add
method.Feature Layer() UniqueValue
assigns a symbol to a value or values. A unique value renderer uses a collection of unique values to assign the appropriate symbol for each feature it renderers.For this example, the renderer uses a feature's
TYPE
attribute value to apply the correct symbol.MapViewModel.csUse dark colors for code blocks private void AddOpenSpaceLayer() { // Create a parks and open spaces feature layer and add it to the map view. FeatureLayer featureLayer = AddFeatureLayer(parksAndOpenSpaces); // Create fill symbols. SimpleFillSymbol purpleFillSymbol = new SimpleFillSymbol(SimpleFillSymbolStyle.Solid, Color.Purple, null); SimpleFillSymbol greenFillSymbol = new SimpleFillSymbol(SimpleFillSymbolStyle.Solid, Color.Green, null); SimpleFillSymbol blueFillSymbol = new SimpleFillSymbol(SimpleFillSymbolStyle.Solid, Color.Blue, null); SimpleFillSymbol redFillSymbol = new SimpleFillSymbol(SimpleFillSymbolStyle.Solid, Color.Red, null); // Create a unique value for natural areas, regional open spaces, local parks, and regional recreation parks. UniqueValue naturalAreas = new UniqueValue("Natural Areas", "Natural Areas", purpleFillSymbol, "Natural Areas"); UniqueValue regionalOpenSpace = new UniqueValue("Regional Open Space", "Regional Open Space", greenFillSymbol, "Regional Open Space"); UniqueValue localPark = new UniqueValue("Local Park", "Local Park", blueFillSymbol, "Local Park"); UniqueValue regionalRecreationPark = new UniqueValue("Regional Recreation Park", "Regional Recreation Park", redFillSymbol, "Regional Recreation Park"); // Create a unique value renderer to display these values. UniqueValueRenderer openSpacesUniqueValueRenderer = new UniqueValueRenderer (new List<string>() { "TYPE" }, new List<UniqueValue>() { naturalAreas, regionalOpenSpace, localPark, regionalRecreationPark }, "Open Spaces", null); // Assign the unique value renderer to the open spaces layer. featureLayer.Renderer = openSpacesUniqueValueRenderer; // Set the layer opacity to semi-transparent. featureLayer.Opacity = 0.2; }
-
Update
Setup
to call the newMap() Add
method.Open Space Layer() MapViewModel.csUse dark colors for code blocks private void SetupMap() { // Create a new map with a 'topographic vector' basemap. Map = new Map(BasemapStyle.ArcGISTopographic); AddOpenSpaceLayer(); }
-
Click Debug > Start Debugging (or press <F5> on the keyboard) to run the app.
When the app opens, Parks and Open Spaces feature layer is added to the map. The map displays the different types of parks and open spaces with four unique symbols.
Add a layer with a class breaks renderer
Create a method to apply a different symbol for each of the five ranges of elevation gain to the Trails feature layer.
-
Add a new method named
Add
just after theTrails Layer() Add
method you created above.Open Space Layer() A
ClassBreak
assigns a symbol to a range of values.For this example, the renderer uses each feature's
ELEV_
attribute value to classify it into a defined range (class break) and apply the corresponding symbol.GAIN MapViewModel.csUse dark colors for code blocks private void AddTrailsLayer() { // Create a trails feature layer and add it to the map view. FeatureLayer featureLayer = AddFeatureLayer(trails); // Create simple line symbols. SimpleLineSymbol firstClassSymbol = new SimpleLineSymbol(SimpleLineSymbolStyle.Solid, Color.Purple, 3.0); SimpleLineSymbol secondClassSymbol = new SimpleLineSymbol(SimpleLineSymbolStyle.Solid, Color.Purple, 4.0); SimpleLineSymbol thirdClassSymbol = new SimpleLineSymbol(SimpleLineSymbolStyle.Solid, Color.Purple, 5.0); SimpleLineSymbol fourthClassSymbol = new SimpleLineSymbol(SimpleLineSymbolStyle.Solid, Color.Purple, 6.0); SimpleLineSymbol fifthClassSymbol = new SimpleLineSymbol(SimpleLineSymbolStyle.Solid, Color.Purple, 7.0); // Create five class breaks. ClassBreak firstClassBreak = new ClassBreak("Under 500", "0 - 500", 0.0, 500.0, firstClassSymbol); ClassBreak secondClassBreak = new ClassBreak("501 to 1000", "501 - 1000", 501.0, 1000.0, secondClassSymbol); ClassBreak thirdClassBreak = new ClassBreak("1001 to 1500", "1001 - 1500", 1001.0, 1500.0, thirdClassSymbol); ClassBreak fourthClassBreak = new ClassBreak("1501 to 2000", "1501 - 2000", 1501.0, 2000.0, fourthClassSymbol); ClassBreak fifthClassBreak = new ClassBreak("2001 to 2300", "2001 to 2300", 2001.0, 2300.0, fifthClassSymbol); List<ClassBreak> elevationBreaks = new List<ClassBreak>() { firstClassBreak, secondClassBreak, thirdClassBreak, fourthClassBreak, fifthClassBreak }; // Create and assign a class breaks renderer to the feature layer. ClassBreaksRenderer elevationClassBreaksRenderer = new ClassBreaksRenderer("ELEV_GAIN", elevationBreaks); featureLayer.Renderer = elevationClassBreaksRenderer; // Set the layer opacity to semi-transparent. featureLayer.Opacity = 0.75; }
-
Update
Setup
to call the newMap() Add
method.Trails Layer() MapViewModel.csUse dark colors for code blocks private void SetupMap() { // Create a new map with a 'topographic vector' basemap. Map = new Map(BasemapStyle.ArcGISTopographic); AddOpenSpaceLayer(); AddTrailsLayer(); }
-
Click Debug > Start Debugging (or press <F5> on the keyboard) to run the app.
When the app opens, the Trails feature layer is added to the map. The map displays trails with different symbols depending on trail elevation.
Add layers with definition expressions
You can use a definition expression to define a subset of features to display. Features that do not meet the expression criteria are not displayed by the layer. In the following steps, you will create two methods that use a definition expression to apply a symbol to a subset of features in the Trails feature layer.
FeatureLayer.DefinitionExpression
uses a SQL expression to limit the features available for query and display. Your code will create two layers that each display a different subset of trails based on the value for the USE_
field. Trails that allow bikes will be symbolized with a blue symbol ("USE_
) and those that don't will be red ("USE_
). Another way to symbolize these features would be to create a UniqueValueRenderer
that applies a different symbol for these values.
-
Add a method named
Add
with a definition expression to filter for trails that permit bikes. Add this method just after the newly addedBike Only Trails Layer() Add
method.Trails Layer() MapViewModel.csUse dark colors for code blocks private void AddBikeOnlyTrailsLayer() { // Create a trails feature layer and add it to the map view. FeatureLayer featureLayer = AddFeatureLayer(trails); // Write a definition expression to filter for trails that permit the use of bikes. featureLayer.DefinitionExpression = "USE_BIKE = 'Yes'"; // Create and assign a simple renderer to the feature layer. SimpleLineSymbol bikeTrailSymbol = new SimpleLineSymbol(SimpleLineSymbolStyle.Dot, Color.Blue, 2.0); SimpleRenderer bikeTrailRenderer = new SimpleRenderer(bikeTrailSymbol); featureLayer.Renderer = bikeTrailRenderer; }
-
Add another method named
Add
with a definition expression to filter for trails that don't allow bikes. Add this method just after theNo Bike Trails Layer() Add
method.Bike Only Trails Layer() MapViewModel.csUse dark colors for code blocks private void AddNoBikeTrailsLayer() { // Create a trails feature layer and add it to the map view. FeatureLayer featureLayer = AddFeatureLayer(trails); // Write a definition expression to filter for trails that don't permit the use of bikes. featureLayer.DefinitionExpression = "USE_BIKE = 'No'"; // Create and assign a simple renderer to the feature layer. SimpleLineSymbol noBikeTrailSymbol = new SimpleLineSymbol(SimpleLineSymbolStyle.Dot, Color.Red, 2.0); SimpleRenderer noBikeTrailRenderer = new SimpleRenderer(noBikeTrailSymbol); featureLayer.Renderer = noBikeTrailRenderer; }
-
Update
Setup
to call the newMap() Add
andBike Only Trails Layer() Add
methods.No Bike Trails Layer() MapViewModel.csUse dark colors for code blocks private void SetupMap() { // Create a new map with a 'topographic vector' basemap. Map = new Map(BasemapStyle.ArcGISTopographic); AddOpenSpaceLayer(); AddTrailsLayer(); AddNoBikeTrailsLayer(); AddBikeOnlyTrailsLayer(); }
-
Click Debug > Start Debugging (or press <F5> on the keyboard) to run the app.
When the app opens, two Trails feature layers are added to the map. One shows where bikes are permitted and the other where they are prohibited.
Symbolize a layer with a picture symbol and label features with an attribute
Create a method to style trailheads with hiker images and labels for the Trailheads feature layer.
Feature layers, graphics overlays, and map image layer sublayers in your app can be labeled using a combination of attribute values, text strings, and values calculated with an expression. You can determine how labels are positioned and prioritized, and how conflicts between overlapping labels are automatically and dynamically resolved.
For feature layers, graphics overlays, and map image sublayers, labeling is implemented using a collection of LabelDefinition
objects to define what labels look like (font, size, color, angle, and so on), the scale at which they display, the text they contain, how they handle overlaps, and so on.
If you want to label everything in your layer or overlay to look identical, you can define a single label definition. If you want to use different label formatting for different attribute values, you can add as many label definitions as you need to define distinct sets of geoelements for labeling.
-
Create a helper method named
Make
to define a label definition based on the feature layer attribute that's passed in. The function will also define the label placement and text symbol. Add this method just after theLabel Definition() Add
method you created earlier.No Bike Trails Layer() MapViewModel.csUse dark colors for code blocks private LabelDefinition MakeLabelDefinition(string labelAttribute) { // Create a new text symbol. TextSymbol labelTextSymbol = new TextSymbol { Color = Color.White, Size = 12.0, HaloColor = Color.Red, HaloWidth = 1.0, FontFamily = "Arial", FontStyle = FontStyle.Italic, FontWeight = FontWeight.Normal }; // Create a new Arcade label expression based on the field name. ArcadeLabelExpression labelExpression = new ArcadeLabelExpression("$feature." + labelAttribute); // Create and return the label definition. return new LabelDefinition(labelExpression, labelTextSymbol ); }
-
Add a method named
Add
. Add this method just after the newTrailheads Layer() Make
method.Label Definition() Use a
PictureMarkerSymbol
to draw a trailhead hiker image. Use theLabelDefinition
to label each trailhead by its name.MapViewModel.csUse dark colors for code blocks private void AddTrailheadsLayer() { // Create a trailheads feature layer and add it to the map view. FeatureLayer featureLayer = AddFeatureLayer(trailheads); // Create a new picture marker symbol that uses the trailhead image. PictureMarkerSymbol pictureMarkerSymbol = new PictureMarkerSymbol(trailheadImage); pictureMarkerSymbol.Height = 18.0; pictureMarkerSymbol.Width = 18.0; // Create a new simple renderer based on the picture marker symbol. SimpleRenderer simpleRenderer = new SimpleRenderer(pictureMarkerSymbol); // Set the feature layer's renderer and enable labels. featureLayer.Renderer = simpleRenderer; featureLayer.LabelsEnabled = true; // Create the label definition and pass the "TRL_NAME" attribute to label features by trail name. LabelDefinition trailHeadsDefinition = MakeLabelDefinition("TRL_NAME"); // Add the label definition to the layer's label definition collection. featureLayer.LabelDefinitions.Add(trailHeadsDefinition); }
-
Update
Setup
to call the newMap() Add
method.Trailheads Layer() MapViewModel.csUse dark colors for code blocks private void SetupMap() { // Create a new map with a 'topographic vector' basemap. Map = new Map(BasemapStyle.ArcGISTopographic); AddOpenSpaceLayer(); AddTrailsLayer(); AddNoBikeTrailsLayer(); AddBikeOnlyTrailsLayer(); AddTrailheadsLayer(); }
-
Click Debug > Start Debugging (or press <F5> on the keyboard) to run the app.
When the app opens, all the layers you've created and symbolized are displayed on the map.
- Parks and open spaces are displayed with four unique symbols
- Trails use different symbols (line widths) depending on trail elevation
- Trails are blue where bikes are permitted and red where they are prohibited
- Trailheads are displayed with a hiker icon and labels display each trail's name
What's next?
Learn how to use additional API features, ArcGIS location services, and ArcGIS tools in these tutorials: