Graphics can represent dynamic objects in the scene. A service might provide near real-time locations for a fleet of vehicles, for example. Your device might read GPS information and display your current location as a graphic, or you might simply animate graphics on the scene view by reading a hard-coded list of geographic coordinates. For these use cases, there are two ways in which your user might want to visualize moving graphics in a scene view. They might want to view several graphics at once as they interactively explore the entire scene, or they might want to continuously point the camera at a specific element and track it as it moves through the scene. Your app might even allow the user to interact both ways, sometimes selecting a particular graphic to follow, and then switching to explore the entire scene.
Visualize dynamic graphics in a scene
A scene view uses the concept of a camera and a focal point to display data in three dimensions. The camera represents the location of the observer in three-dimensional space (x, y, z), and the camera's focal point is the location that intersects the camera's line of sight. By default, a scene view allows the user to interactively change the camera position to center on a focal point of their choice. However, you may need to set the camera's focal point relative to a specific graphic or location in the scene view. In the case of a moving graphics, you may want to continuously update the camera to maintain focus on a particular graphic as it moves through the scene.
A CameraController
is provided to simplify the code required to keep the camera in sync with a moving graphic.
Prepare your graphics
To ensure your graphics can be tracked and properly displayed using the method described in this topic, follow these guidelines:
- Graphics you want to follow should have attributes to store the current heading, pitch, and roll.
- Each graphics overlay containing graphics you want to follow should have a renderer assigned.
- The graphics overlay renderer should have heading, pitch, and roll expressions defined in the renderer scene properties. These expressions should use the corresponding graphic attributes.
- The graphics overlay that contains the graphic to follow must be added to the scene view.
You may need to adjust the heading, pitch, and roll properties on your symbol to make sure its initial appearance is correct.
You can create a graphics overlay with the appropriate scene properties, add a single point graphic to it, and then add it to a scene view. The graphic _plane
would then be ready to follow in the scene.
// create a new RendererSceneProperties
var sceneProps = new RendererSceneProperties
{
// set heading, pitch, and roll expressions that use the corresponding graphic attribute names
HeadingExpression = "[Heading]",
PitchExpression = "[Pitch]",
RollExpression = "[Roll]"
};
// create a new simple renderer and apply the scene properties
var graphicsRenderer = new SimpleRenderer();
graphicsRenderer.SceneProperties = sceneProps;
// create a new graphics overlay, define absolute placement of graphics, and apply the renderer
var followGraphicsOverlay = new GraphicsOverlay();
followGraphicsOverlay.SceneProperties.SurfacePlacement = SurfacePlacement.Absolute;
followGraphicsOverlay.Renderer = graphicsRenderer;
// create a point graphic to track
var startPoint = new MapPoint(-117.00, 31.50, 2000.0, SpatialReferences.Wgs84);
_planeGraphic = new Graphic(startPoint, planeSymbol);
_planeGraphic.Attributes["Heading"] = 270.0;
_planeGraphic.Attributes["Pitch"] = 25.0;
_planeGraphic.Attributes["Roll"] = 12.0;
// add the graphic to the overlay
followGraphicsOverlay.Graphics.Add(_planeGraphic);
// add the graphics overlay to the scene view
MySceneView.GraphicsOverlays.Add(followGraphicsOverlay);
Update graphic position
When using a camera controller to follow a graphic, update the position of the graphic and the camera updates its location accordingly. Provide a new MapPoint
for the graphic's geometry, and update the heading, pitch, and roll attributes as needed. The graphics overlay renderer (and associated scene properties) controls updating the display of the graphic's heading, pitch, and roll.
_planeGraphic.Geometry = newMapPoint;
_planeGraphic.Attributes["Heading"] = newHeading;
_planeGraphic.Attributes["Pitch"] = newPitch;
_planeGraphic.Attributes["Roll"] = newRoll;
Camera controllers
A scene view has an associated controller that manages the camera for the scene. Each type of camera controller is designed to provide a specific user experience for interacting with the scene display. The camera controller and its properties can be changed at run time, so your app can provide the scene interaction experience best suited for the current context.
The following camera controllers are provided:
GlobeCameraController
(default) — Provides the default scene view camera behavior. Allows the user to freely move and focus the camera anywhere in the scene.OrbitGeoElementCameraController
— Locks the scene view's camera to maintain focus relative to a (possibly moving) graphic. The camera can only move relative to the target graphic.OrbitLocationCameraController
— Locks the scene view's camera to orbit a fixed location (map point). The camera can only move relative to the target map point.
When any camera controller other than GlobeCameraController
is active, the scene view's viewpoint cannot be assigned. Attempts to do so do not raise an exception, but they are ignored.
Use a camera controller to follow a graphic
The OrbitGeoElementCameraController
updates the camera focal point relative to a specified (stationary or moving) graphic. As the user interacts with the scene view, the scene view's camera follows a focal point specified by the target graphic. Using this controller, the camera moves in sync with the target.
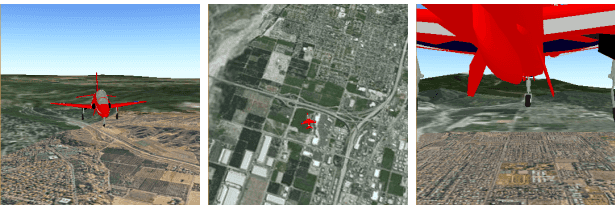
You could create a new OrbitGeoElementCameraController
and assign it to the SceneView
. The Graphic
to follow is passed to the object's constructor, along with the initial distance from the target to the camera. By default, the camera is positioned with a heading of 0 and a pitch of 45 degrees. See Adjust the display for information about modifying camera settings.
// create an OrbitGeoElementCameraController, pass in the target graphic and initial camera distance
var orbitGraphicController = new OrbitGeoElementCameraController(_planeGraphic, 1000);
MySceneView.CameraController = orbitGraphicController;
The graphic associated with an OrbitGeoElementCameraController
cannot be changed after the controller is initialized. To follow another graphic, you must create a new OrbitGeoElementCameraController
for the other graphic and set it as the scene view's camera controller.
Adjust the display
Although the OrbitGeoElementCameraController
locks the camera to a target graphic, the exact location of the camera in relation to the target can be adjusted using offset values. Other aspects of the scene display can also be modified, such as whether the camera should automatically adjust its heading, pitch, and roll when those values change in the tracked graphic. Adjustments to the camera display, as well as how to limit user interaction with the scene view, can be changed dynamically at run time.
Camera target offsets
The position of the camera focal point relative to the target graphic can be adjusted using x, y, and z target offsets. This allows you to make precise adjustments to the display of the scene, for example, placing the camera inside the cockpit of an airplane graphic. These offsets can be assigned individually or applied at once using an animation.
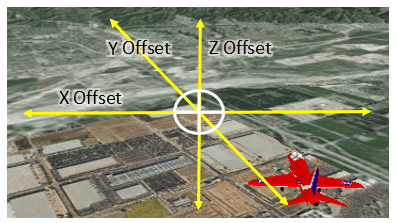
You can apply offsets for the OrbitGeoElementCameraController
.
// set the x, y, and z camera target offsets (animate change over 2 seconds)
await orbitGraphicController.SetTargetOffsetsAsync(43.0, 0.0, -55.0, 2.0);
You can also use OrbitGeoElementCameraController.TargetVerticalScreenFactor
to adjust the vertical position of the target in the display. Acceptable values range from 0 (bottom of the display) to 1 (top of the display). The default is 0.5, which places the target in the center of the display.
Auto heading, pitch, and roll
Auto heading, when enabled for the camera controller, means that the camera will rotate to follow changes in the graphic's heading and therefore provide a consistent view of the graphic. When auto heading is disabled, the camera remains at a constant heading and the graphic may appear to rotate as its heading changes.
Auto pitch and auto roll have similar effects. When enabled, the camera rotates with the target's pitch or roll and the surface appears to rotate around the graphic, as shown on the left in the following image. When disabled, the camera is not affected by changes in the graphic's pitch or roll.
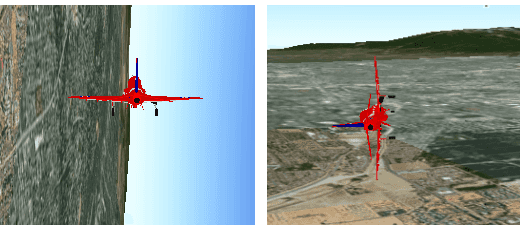
These settings are true
by default:
-
OrbitGeoElementCameraController.IsAutoHeadingEnabled
-
OrbitGeoElementCameraController.IsAutoPitchEnabled
-
OrbitGeoElementCameraController.IsAutoRollEnabled
Move the camera
While the OrbitGeoElementCameraController
is associated with the scene view, the camera can be moved relative to the target graphic (either programmatically or interactively).
- Camera distance — The distance in meters between the camera and its target. The camera position is derived from this distance plus the camera offsets.
- Camera heading offset — The clockwise angle measured in the target element symbol's horizontal plane starting directly behind the symbol (default is 0). Any angle in degrees is valid but will be normalized between the minimum and maximum camera heading offsets. If after normalization the value is outside of the allowed range, the nearest value within the range will be used.
- Camera pitch offset — The counterclockwise angle from the positive z-axis of the target element's symbol relative to the symbol's horizontal plane, as illustrated in the following image (default is 45). If the value specified is outside of the range defined by the minimum and maximum camera pitch offsets, the nearest value within the range will be used.
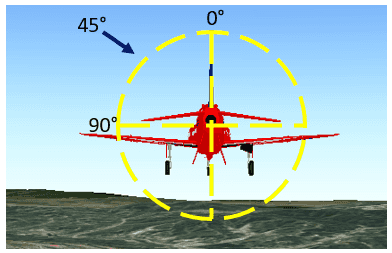
You can move the camera using the OrbitGeoElementCameraController
.
// update camera position: delta values for distance, heading, and pitch (animate change over 2 seconds)
await orbitGraphicController.MoveCameraAsync(300.0, 0.0, 45.0, 2.0);
Limit user interaction
When using an OrbitGeoElementCameraController
or OrbitLocationCameraController
, you can define minimum and maximum values for the camera distance, heading, pitch, and roll properties if you want to further restrict user interaction with the scene view. User interactions and scene view animations obey these limits.
You could limit the distance and pitch for the camera, but not the heading.
// limit the camera distance to between 1,000 and 10,000 meters
orbitLocationController.MinCameraDistance = 1000;
orbitLocationController.MaxCameraDistance = 10000;
// limit the camera pitch to between 25° and 75°
orbitLocationController.MinCameraPitchOffset = 25;
orbitLocationController.MaxCameraPitchOffset = 75;
Types of scene view interaction can also be completely disabled if needed. You may want the user to interact with the scene view by changing the camera heading and pitch, but not the camera distance, for example.
You could disable the ability for the user to modify the camera distance when interacting with the scene view.
// restrict the ability to interactively change the camera distance
orbitLocationController.IsCameraDistanceInteractive = false;
Stop following a graphic
To stop following a graphic, reset the scene view's camera controller to the default, GlobeCameraController
.
In general, the only time you need to assign this camera controller to the scene view is to return to the default behavior after applying one of the non-default controllers.
You can apply a new GlobeCameraController
to the SceneView
.
// apply the (default) globe camera controller
MySceneView.CameraController = new GlobeCameraController();
The globe camera controller allows the user to interactively change the camera position, focal point, and any of the camera properties. Use this controller to let your user freely explore the scene. See Navigate a scene view for more information about interacting with a scene view using the globe camera controller.