Using the routing service, your own route services, or a route dataset stored on the device, you can find routes, directions, and perform advanced analyses on street networks. You can solve network problems such as finding the closest emergency vehicle or facility, identifying a service area around a location, or servicing a set of orders with a fleet of vehicles.
Your routing app can do things like:
- Calculate point-to-point and multi-stop routes
- Optimize the results to find the shortest or the fastest route
- Find the best sequence between stops
- Create routes based on the travel mode (driving, cycling, walking, and so on)
- Avoid restricted areas and maneuvers
- Specify time windows of arrival and departure for each stop
- Generate driving directions in multiple languages
What is routing?
Routing is the process of finding the best path between two or more stops in a street network. A common use case is to find a route between two stops, from an origin to a destination. Routing takes into consideration many different attributes in the street network such as speed limit, number of lanes, and time of day. Routing can also incorporate real-time data such as road conditions, accidents, street closures, and other restrictions to travel.
In addition to the routing described above, functionality is provided to analyze street networks. This includes the ability to create service areas (to find customers within a certain drive time, for example) or locate a closest facility (nearest hospital to an accident, for example).
Directions
If requested, directions are returned for each route in the results. Directions provide specific instructions for traveling the route. These are often referred to as "driving directions", but depending on the travel mode, could describe walking or cycling to the destination. Depending on the services configuration, directions can be returned in more than one language. Supported languages for a service are available in the service metadata.
Directions are composed of maneuvers. Each maneuver contains properties such as the direction text, which contains instructions that describe travel through a section of a route. A direction maneuver is further broken down into maneuver messages that give details about a specific maneuver.
If supported by the service, direction maneuver’s can include from level and to level values to define things like floor levels inside a building. For example, a user may want to see just one level of a walking route that spans multiple floors.
Navigation
The navigation API allows you to further enhance the routing experience using the current device location to track progress and provide navigation instructions (maneuvers) as the user travels the route. You can integrate driving directions with your device's text-to-speech capability and automatically recalculate a new route when the user leaves the current one.
The route tracker object provides the following functionality using the current device location and an appropriate route result:
- Progress information relative to the next stop, to the next maneuver, or along the entire route
- Guidance for navigation as it's needed (when approaching a maneuver, for example)
- Automatic recalculation of a route if the device location goes off route
How routing works
Online and local routing both rely on a transportation network to model travel. These networks are created from features (lines and points) that represent roads, bridges, tunnels, bike paths, train tracks, and other entities in the network. The geometric intersections of features help to define connectivity between the network entities they represent. The connectivity of the transportation network, along with resistance to travel (speed limit, for example) and other network properties, is analyzed to solve routing problems.
Network Analyst services are hosted in ArcGIS or can be published on your own ArcGIS servers. These services provide a REST API for clients such as mobile and Web applications. For more information about publishing routing services with ArcGIS Enterprise, see Publish routing services.
You can create a transportation network using ArcGIS Pro and deploy it to your device using either a mobile map package file (.mmpk
), a mobile scene package file (.mspk
), or a mobile geodatabase file. The code to work with a local transportation network (stored directly on the device) is identical to the code for using a routing service.
If you'd like a ready-to-use and regularly updated network dataset (and locator) for your area of interest, you can license StreetMap Premium data (in mobile map package format). For details, see Add StreetMap Premium data.
Route task
A route task is a network analysis task that is executed asynchronously. It returns results with details about a route that visits two or more stops (locations) within a transportation network. This operation is sometimes referred to as solving a route.
The RouteTask
refers to the local transportation network dataset or online service. If solves a Route
using the configured RouteParameters
and reports the RouteResult
.
// Create a RouteTask from an online route service
var routeTask = await RouteTask.CreateAsync(new Uri(
"https://route-api.arcgis.com/arcgis/rest/services/World/Route/NAServer/Route_World"));
Route parameters
Route task parameters specify how a route should be found. There are many parameters that let you customize how the route is determined. Parameters include stops and barriers. Other parameters include travel mode, whether stops map be reordered to find an optimized route, the units to be used in the turn-by-turn directions (miles or kilometers, for example), and so on.
To initially create RouteParameters
, call RouteTask.makeDefaultParameters()
to retrieve the default routing parameters defined for the service. You can then change individual route parameters as needed before executing the RouteTask
. The service's default parameters typically support the most common use case anticipated for that service. Different services can have different defaults.
// Get the route services default parameters
var routeParameters = await routeTask.CreateDefaultParametersAsync();
// Create a list of stops using the graphic's geometry (points)
var stops = new[] {
new Stop((MapPoint)originGraphic.Geometry),
new Stop((MapPoint)intermediateStopGraphic.Geometry),
new Stop((MapPoint)destinationGraphic.Geometry) };
// Add the stops to the route parameters
routeParameters.SetStops(stops);
// Return driving directions in Spanish
routeParameters.ReturnDirections = true;
routeParameters.DirectionsLanguage = "es";
Route results
The results returned from finding a route contain a collection of routes. Each route is represented by a polyline geometry and has information such as its total length and travel time. Depending on how you configured your route parameters, the results may also contain barriers, stops, and driving directions. Typically, the route, stops, and barriers are displayed as graphics in the map. Directions can be shown in a list or other UI element in the app.
// Solve the route using the route parameters
var routeResult = await routeTask.SolveRouteAsync(routeParameters);
// If a result is returned, display the route
if (routeResult?.Routes?.FirstOrDefault() is Route route)
{
// Show the route as a graphic in the map view
var routeGraphic = new Graphic(route.RouteGeometry);
_routeOverlay.Graphics.Add(routeGraphic);
// Get the driving directions
var directions = string.Join("\n", route.DirectionManeuvers.Select(maneuver => maneuver.DirectionText));
DirectionsTextBlock.Text = directions;
}
Examples
Find a route and directions
Use the routing service to find a route between a set of stops. The result will account for current traffic conditions and provide driving directions in Spanish.
To find a route, you need to define at least two stops to visit. The default travel mode is driving, but you can use walk or trucking travel modes as well. It is always good to provide the start time to get the best results (to consider the appropriate traffic conditions, for example). In this example, the start time is set with the current time which indicates to the service that the route should use the current traffic conditions. The directions language is set to "es" to generate driving directions in Spanish.
The result contains a set of ordered stops to visit, the route segments, and turn-by-turn text directions.
// Create a RouteTask from an online route service
var routeTask = await RouteTask.CreateAsync(new Uri(
"https://route-api.arcgis.com/arcgis/rest/services/World/Route/NAServer/Route_World"));
// Get the route services default parameters
var routeParameters = await routeTask.CreateDefaultParametersAsync();
// Create a list of stops using the graphic's geometry (points)
var stops = new[] {
new Stop((MapPoint)originGraphic.Geometry),
new Stop((MapPoint)intermediateStopGraphic.Geometry),
new Stop((MapPoint)destinationGraphic.Geometry) };
// Add the stops to the route parameters
routeParameters.SetStops(stops);
// Return driving directions in Spanish
routeParameters.ReturnDirections = true;
routeParameters.DirectionsLanguage = "es";
// Solve the route using the route parameters
var routeResult = await routeTask.SolveRouteAsync(routeParameters);
// If a result is returned, display the route
if (routeResult?.Routes?.FirstOrDefault() is Route route)
{
// Show the route as a graphic in the map view
var routeGraphic = new Graphic(route.RouteGeometry);
_routeOverlay.Graphics.Add(routeGraphic);
// Get the driving directions
var directions = string.Join("\n", route.DirectionManeuvers.Select(maneuver => maneuver.DirectionText));
DirectionsTextBlock.Text = directions;
}
Use API keys
An API Key access token can be used to authorize access to ArcGIS Online services and resources from your app, as well as to monitor access to those services. You can use a single access token for all requests made by your app, or assign individual access tokens for any classes that implements APIKeyResourceInterface
Tutorials
Samples
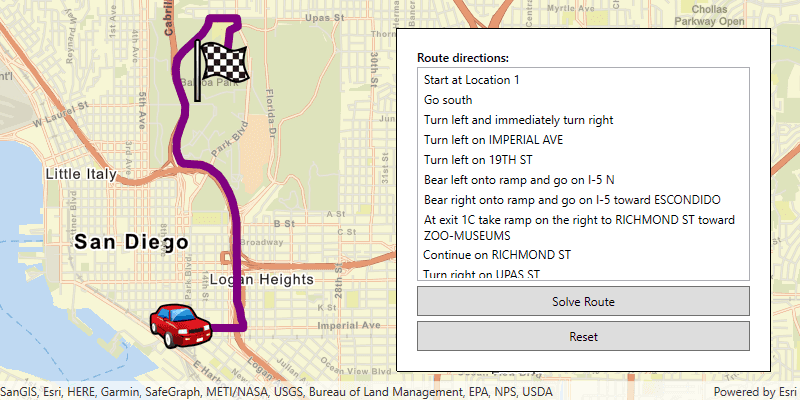
Find route
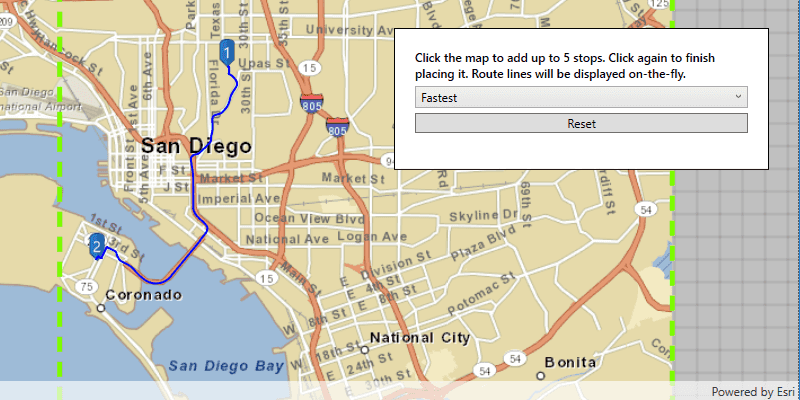
Offline routing
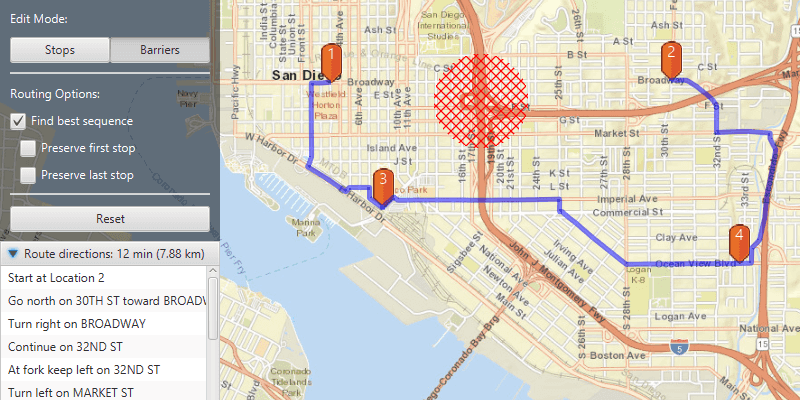
Route around barriers
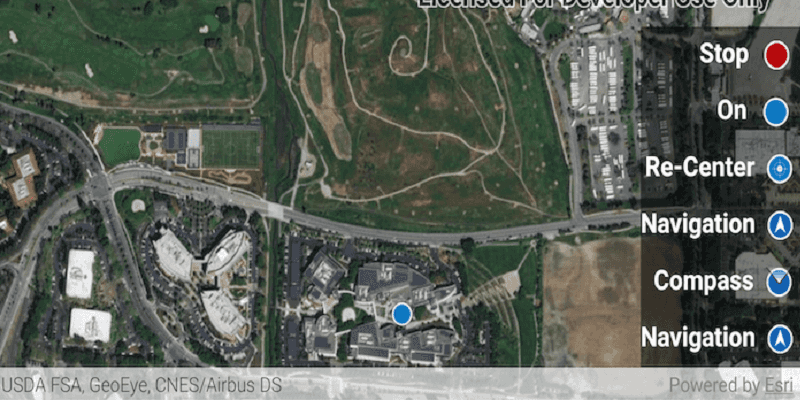
Display device location with autopan modes
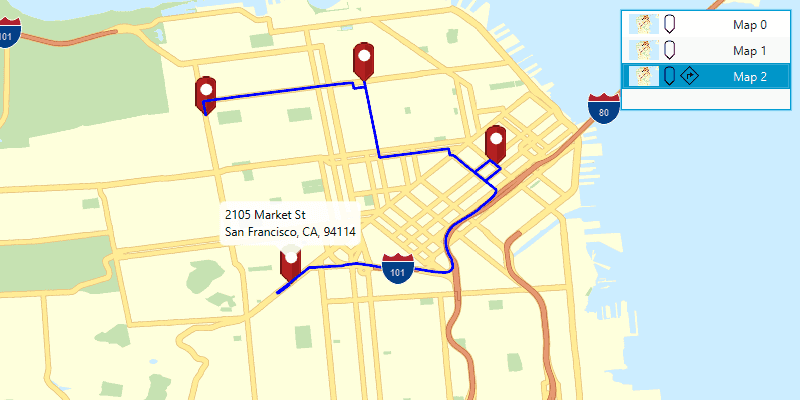
Mobile map (search and route)
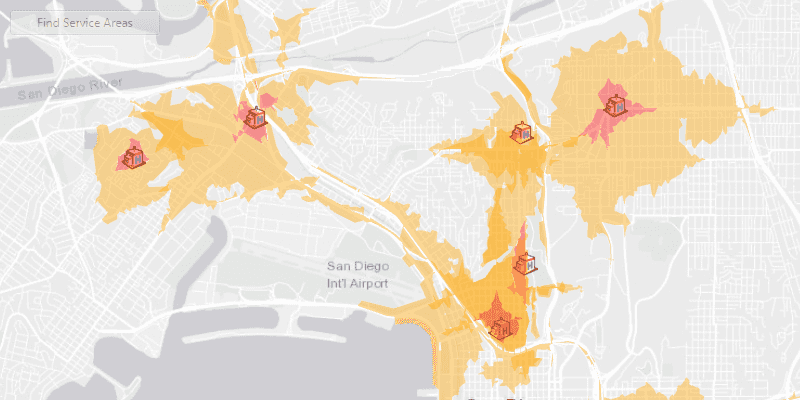
Find service area
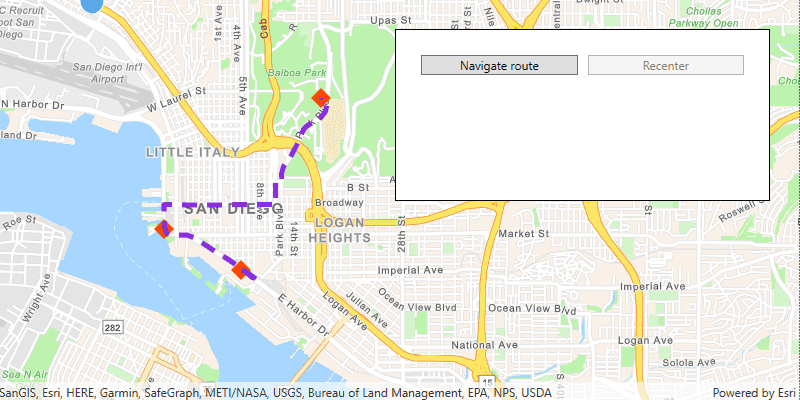