Learn how to use an ArcGIS portal item to access and display a feature layer in a map.
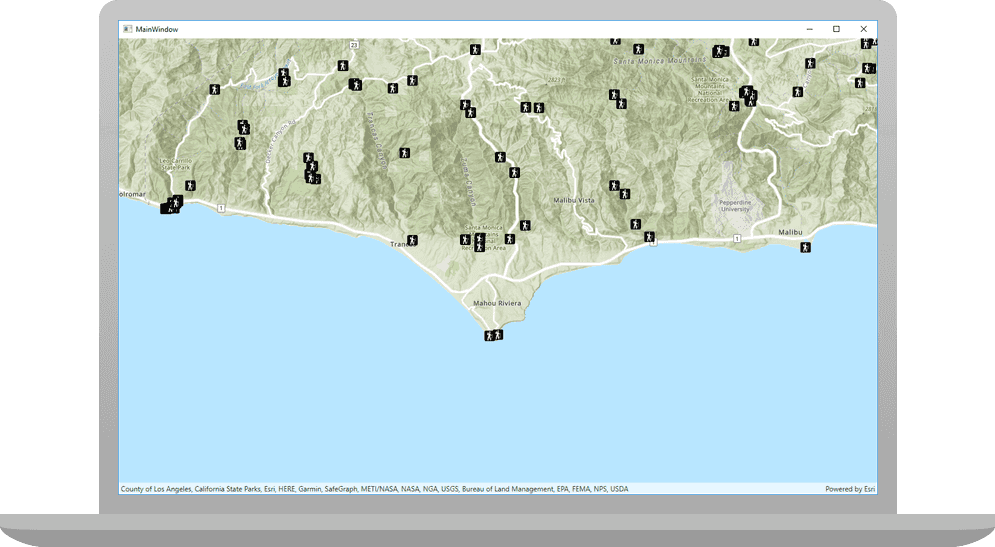
You can host a variety of geographic data and other resources using ArcGIS Online. These portal items can also define how the data is presented. A web map or web scene, for example, not only defines the layers for a map or scene, but also how layers are symbolized, the minimum and/or maximum scales at which they display, and several other properties. Likewise, a hosted feature layer contains the data for the layer and also defines the symbols and other display properties for how it is presented. When you add a map, scene, or layer from a portal item to your app, everything that has been saved with the item is applied in your app. Adding portal items to your app rather than creating them programmatically saves you from writing a lot of code, and can provide consistency across apps that use the same data.
In this tutorial, you will add a hosted feature layer to display trailheads in the Santa Monica Mountains of Southern California. The hosted layer defines the trailhead locations (points) as well as the symbols used to display them.
Prerequisites
Before starting this tutorial:
-
You need an ArcGIS Location Platform or ArcGIS Online account.
-
Ensure your development environment meets the system requirements.
Optionally, you may want to install the ArcGIS Maps SDK for .NET to get access to project templates in Visual Studio (Windows only) and offline copies of the NuGet packages.
Steps
Open the Visual Studio solution
-
To start the tutorial, complete the Display a map tutorial or download and unzip the solution.
-
Open the
.sln
file in Visual Studio. -
If you downloaded the solution, get an access token and set the API key.
An API Key gives your app access to secure resources used in this tutorial.
-
Go to the Create an API key tutorial to obtain a new API key access token. Ensure that the following privilege is enabled: Location services > Basemaps > Basemap styles service. Copy the access token as it will be used in the next step.
-
In Visual Studio, in the Solution Explorer, click App.xaml.cs.
-
In the App class, add an override for the
O
function to set then Startup() Api
property onKey ArcGISRuntimeEnvironment
.App.xaml.csUse dark colors for code blocks public partial class App : Application { protected override void OnStartup(StartupEventArgs e) { base.OnStartup(e); Esri.ArcGISRuntime.ArcGISRuntimeEnvironment.ApiKey = "YOUR_ACCESS_TOKEN"; } } }
-
If you are developing with Visual Studio for Windows, ArcGIS Maps SDK for .NET provides a set of project templates for each supported .NET platform. These templates follow the Model-View-ViewModel (MVVM) design pattern. Install the ArcGIS Maps SDK for .NET Visual Studio Extension to add the templates to Visual Studio (Windows only). See Install and set up for details.
Update the tutorial name used in the project (optional)
The Visual Studio solution, project, and the namespace for all classes currently use the name Display
. Follow the steps below if you prefer the name to reflect the current tutorial. These steps are not required, your code will still work if you keep the original name.
The tutorial instructions and code use the name Add
for the solution, project, and namespace. You can choose any name you like, but it should be the same for each of these.
-
Update the name for the solution and the project.
- In Visual Studio, in the Solution Explorer, right-click the solution name and choose Rename. Provide the new name for your solution.
- In the Solution Explorer, right-click the project name and choose Rename. Provide the new name for your project.
-
Rename the namespace used by classes in the project.
- In the Solution Explorer, expand the project node.
- Double-click MapViewModel.cs in the Solution Explorer to open the file.
- In the
Map
class, double-click the namespace name (View Model Display
) to select it, and then right-click and choose Rename....AMap - Provide the new name for the namespace.
- Click Apply in the Rename: DisplayAMap window that appears in the upper-right of the code window. This will rename the namespace throughout your project.
-
Build the project.
- Choose Build > Build solution (or press <F6>).
Display the ArcGIS portal item
You can reference an item (such as a web map or feature layer) hosted in a portal (such as ArcGIS Online) using its unique item ID. You will reference the Trailheads Styled feature layer stored in ArcGIS Online using its item ID of: 2e4b3df6ba4b44969a3bc9827de746b3
. You will then add that feature layer to your map's collection of data layers (operational layers).
-
In Visual Studio, in the Solution Explorer, double-click MapViewModel.cs to open the file.
-
Add additional required
using
statements near the top of the class file.MapViewModel.csUse dark colors for code blocks using System; using System.Collections.Generic; using System.Text; using Esri.ArcGISRuntime.Geometry; using Esri.ArcGISRuntime.Mapping; using System.ComponentModel; using System.Runtime.CompilerServices; using Esri.ArcGISRuntime.Portal; using System.Threading.Tasks;
-
Modify the signature of the
Setup
function to include theMap() async
keyword and to returnTask
rather thanvoid
.MapViewModel.csUse dark colors for code blocks private async Task SetupMap() { // Create a new map with a 'topographic vector' basemap. Map = new Map(BasemapStyle.ArcGISTopographic); }
When calling methods asynchronously inside a function (using the
await
keyword), theasync
keyword is required in the signature.Although a
void
return type would continue to work, this is not considered best practice. Exceptions thrown by anasync void
method cannot be caught outside of that method, are difficult to test, and can cause serious side effects if the caller is not expecting them to be asynchronous. The only circumstance whereasync void
is acceptable is when using an event handler, such as a button click.See the Microsoft documentation for more information about Asynchronous programming with async and await.
-
In the
Map
constructor, modify the call toView Model Setup
to avoid a compilation warning. After changingMap() Setup
to an asynchronous method, the following warning appears in the Visual Studio Error List.Map() Use dark colors for code blocks Copy Because this call is not awaited, execution of the current method continues before the call is completed. Consider applying the 'await' operator to the result of the call.
Because your code does not anticipate a return value from this call, the warning can be ignored. To be more specific about your intentions with this call and to address the warning, add the following code to store the return value in a discard.
MapViewModel.csUse dark colors for code blocks public MapViewModel() { _ = SetupMap(); }
From the Microsoft documentation:
"[Discards] are placeholder variables that are intentionally unused in application code. Discards are equivalent to unassigned variables; they don't have a value. A discard communicates intent to the compiler and others that read your code: You intended to ignore the result of an expression."
-
Add code to the
Setup
function to create aMap() PortalItem
object that references the feature layer portal item. To do this, provide the item ID and anArcGISPortal
object.MapViewModel.csUse dark colors for code blocks private async Task SetupMap() { // Create a new map with a 'topographic vector' basemap. Map = new Map(BasemapStyle.ArcGISTopographic); // Create an ArcGIS Portal object. ArcGISPortal portal = await ArcGISPortal.CreateAsync(); // Create a portal item from the ArcGIS Portal object using a portal item string. PortalItem portalItem = await PortalItem.CreateAsync(portal, "2e4b3df6ba4b44969a3bc9827de746b3"); }
-
Create a
FeatureLayer
using thePortalItem
which loads it asynchronously. Then add the feature layer to theMap
's operational layers collection.MapViewModel.csUse dark colors for code blocks private async Task SetupMap() { // Create a new map with a 'topographic vector' basemap. Map = new Map(BasemapStyle.ArcGISTopographic); // Create an ArcGIS Portal object. ArcGISPortal portal = await ArcGISPortal.CreateAsync(); // Create a portal item from the ArcGIS Portal object using a portal item string. PortalItem portalItem = await PortalItem.CreateAsync(portal, "2e4b3df6ba4b44969a3bc9827de746b3"); // Create a feature layer from the portal item and specify a numerical layer id (i.e. 0). FeatureLayer layer = new FeatureLayer(portalItem, 0); // Add the layer to the operational layer of the map. Map.OperationalLayers.Add(layer); }
-
Click Debug > Start Debugging (or press <F5> on the keyboard) to run the app.
You should see a map of trail heads in the Santa Monica mountains. Click, drag, and scroll the mouse wheel on the map view to explore the map.
What's next?
Learn how to use additional API features, ArcGIS location services, and ArcGIS tools in these tutorials: