Learn how to create and display a scene with a basemap layer and an elevation layer. Set properties of the scene's camera to control the 3D perspective.
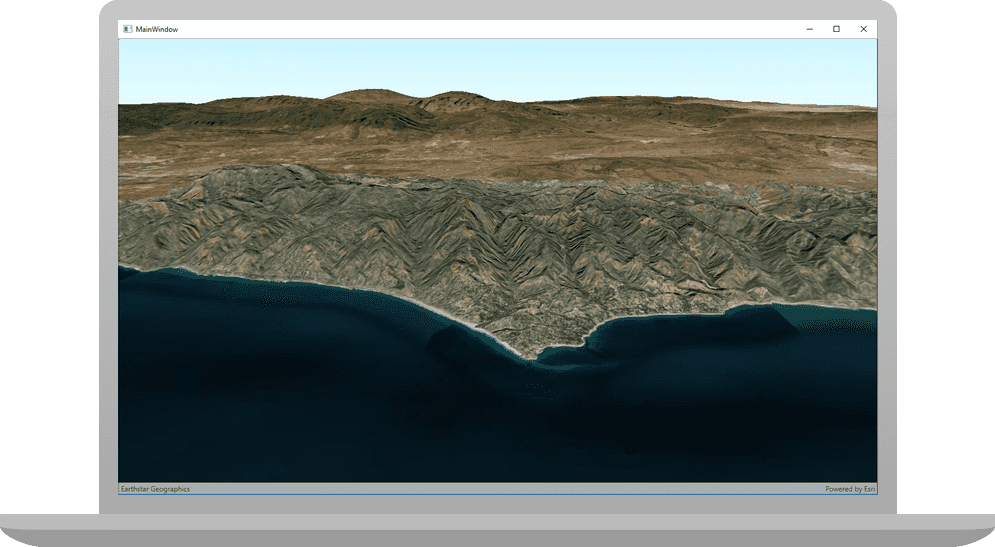
Like a map, a scene contains layers of geographic data. It contains a basemap layer and, optionally, one or more data layers. To provide a realistic view of the terrain, you can also add elevation layers to define the height of the surface across the scene. The 3D perspective of the scene is controlled by the scene's camera, which defines the position of the scene observer in 3D space.
In this tutorial, you create and display a scene using the imagery basemap layer. The surface of the scene is defined with an elevation layer and the camera is positioned to display an area of the Santa Monica Mountains in the scene view.
The scene and code will be used as the starting point for other 3D tutorials.
Prerequisites
Before starting this tutorial:
-
You need an ArcGIS Location Platform or ArcGIS Online account.
-
Ensure your development environment meets the system requirements.
Optionally, you may want to install the ArcGIS Maps SDK for .NET to get access to project templates in Visual Studio (Windows only) and offline copies of the NuGet packages.
Steps
Create a new Visual Studio Project
ArcGIS Maps SDK for .NET supports apps for Windows Presentation Framework (WPF), Universal Windows Platform (UWP), Windows UI Library (WinUI), and .NET MAUI. The instructions for this tutorial are specific to creating a WPF .NET project using Visual Studio for Windows.
-
Start Visual Studio and create a new project.
- In the Visual Studio start screen, click Create a new project.
- Choose the WPF App (.NET) template for C#, then click Next.
- Provide required values in the Configure your new project panel:
- Project name:
Display
AScene - Location:
choose a folder
- Project name:
- Click Create to create the project.
If you are developing with Visual Studio for Windows, ArcGIS Maps SDK for .NET provides a set of project templates for each supported .NET platform. These templates follow the Model-View-ViewModel (MVVM) design pattern. Install the ArcGIS Maps SDK for .NET Visual Studio Extension to add the templates to Visual Studio (Windows only). See Install and set up for details.
Add a reference to the API
-
Add a reference to the API by installing a NuGet package.
- In Solution Explorer, right-click Dependencies and choose Manage NuGet Packages.
- In the NuGet Package Manager window, ensure the selected Package source is
nuget.org
(upper-right). - Select the Browse tab and search for ArcGIS Maps SDK.
- In the search results, select the appropriate package for your platform. For this tutorial project, choose the Esri.ArcGISRuntime.WPF NuGet package.
- Confirm the Latest stable version of the package is selected in the Version dropdown.
- Click Install.
- The Preview Changes dialog confirms any package(s) dependencies or conflicts. Review the changes and click OK to continue installing the packages.
- Review the license information on the License Acceptance dialog and click I Accept to add the package(s) to your project.
- In the Visual Studio Output window, ensure the packages were successfully installed. If you see an error about the target Windows version, you will fix that in the next step.
- Close the NuGet Package Manager window.
-
You may see an error like this in the Visual Studio Error List:
The 'Esri.ArcGISRuntime.WPF' nuget package cannot be used to target 'net8.0-windows'. Target 'net8.0-windows10.0.19041.0' or later instead.
. If so, follow these steps to address it.- In Solution Explorer, right-click the DisplayAMap project entry in the tree view and choose Edit Project File.
- Update the
<
element withTarget Framework> net8.0-windows10.0.19041.0
(or higher).
Use dark colors for code blocks <PropertyGroup> <OutputType>WinExe</OutputType> <TargetFramework>net8.0-windows10.0.19041.0</TargetFramework> <UseWPF>true</UseWPF> </PropertyGroup>
- Save the project file (
Display
) and close it.AMap
Get an access token
You need an access token to use the location services used in this tutorial.
-
Go to the Create an API key tutorial to obtain an access token.
-
Ensure that the following privilege is enabled: Location services > Basemaps > Basemap styles service.
-
Copy the access token as it will be used in the next step.
To learn more about other ways to get an access token, go to Types of authentication.
Set your API key
-
In the Solution Explorer, expand the node for App.xaml, and double-click App.xaml.cs to open it.
-
In the App class, add an override for the
O
function to set then Startup() Api
property onKey ArcGISRuntimeEnvironment
.App.xaml.csUse dark colors for code blocks public partial class App : Application { protected override void OnStartup(StartupEventArgs e) { base.OnStartup(e); Esri.ArcGISRuntime.ArcGISRuntimeEnvironment.ApiKey = "YOUR_ACCESS_TOKEN"; } } }
-
Save and close the
App.xaml.cs
file.
Create a view model to store app logic
Since this app builds the foundation to be used in several following tutorials, it's good to build it with a solid design.
The Model-View-ViewModel (MVVM) design pattern provides an architecture that separates user interface elements, and related code, from the underlying app logic. In this pattern,model
represents the data consumed in an app, view
represents the user interface, and view model
contains the logic that binds model
and view
together. The framework required for such a pattern might initially seem like a lot of work for a small project, but as your project's complexity increases, a solid design foundation will make your code more flexible and easier to maintain.
In an ArcGIS app designed with MVVM, the map view or scene view usually provides the main view
component. Many of the classes fill the role of model
(representing data as maps, scenes, layers, graphics, features, and others). Much of the code you write will be for the view model
component, where you will add logic to work with ArcGIS objects and provide data for display in the view
.
-
Add a new class that defines a
view model
for the project.- Click Project > Add Class ....
- Name the new class
Scene
.View Model.cs - Click Add to create the new class and add it to the project.
- The new class will open in Visual Studio.
-
Add required
using
statements to the view model.SceneViewModel.csUse dark colors for code blocks using System; using System.Collections.Generic; using System.Text; using Esri.ArcGISRuntime.Geometry; using Esri.ArcGISRuntime.Mapping; using System.ComponentModel; using System.Runtime.CompilerServices;
-
Implement the
INotify
interface in theProperty Changed Scene
class.View Model This interface defines a
Property
event that is used to notify clients (views) that a property of the view model has changed.Changed SceneViewModel.csUse dark colors for code blocks namespace DisplayAScene { class SceneViewModel : INotifyPropertyChanged {
-
Inside the
Scene
class, add code to implement theView Model Property
event.Changed When a property of the view model changes, a call to
O
will raise this event.n Property Changed SceneViewModel.csUse dark colors for code blocks class SceneViewModel : INotifyPropertyChanged { public event PropertyChangedEventHandler? PropertyChanged; protected void OnPropertyChanged([CallerMemberName] string propertyName = "") { PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName)); } }
-
Define a new property on the view model called
Scene
that exposes anScene
object. When the property is set, callO
.n Property Changed SceneViewModel.csUse dark colors for code blocks class SceneViewModel : INotifyPropertyChanged { public event PropertyChangedEventHandler? PropertyChanged; protected void OnPropertyChanged([CallerMemberName] string propertyName = "") { PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName)); } private Scene? _scene; public Scene? Scene { get { return _scene; } set { _scene = value; OnPropertyChanged(); } } }
-
Add a function to the
Scene
class calledView Model Setup
. Start the function by creating a newScene Scene
using theArcGISImagery
field of theStandard Basemap
enum.Style SceneViewModel.csUse dark colors for code blocks private void SetupScene() { // Create a new scene with an imagery basemap. Scene scene = new Scene(BasemapStyle.ArcGISImageryStandard); }
-
Create an
ElevationSource
to define the base surface for the scene.An elevation source can define a surface with 3D terrain in a scene. Without an elevation source, the default globe surface is used to display the scene.
SceneViewModel.csUse dark colors for code blocks // Create a new scene with an imagery basemap. Scene scene = new Scene(BasemapStyle.ArcGISImageryStandard); // Create an elevation source to show relief in the scene. string elevationServiceUrl = "http://elevation3d.arcgis.com/arcgis/rest/services/WorldElevation3D/Terrain3D/ImageServer"; ArcGISTiledElevationSource elevationSource = new ArcGISTiledElevationSource(new Uri(elevationServiceUrl)); // Create a Surface with the elevation data. Surface elevationSurface = new Surface(); elevationSurface.ElevationSources.Add(elevationSource); // Add an exaggeration factor to increase the 3D effect of the elevation. elevationSurface.ElevationExaggeration = 2.5; // Apply the surface to the scene. scene.BaseSurface = elevationSurface;
-
Define the initial viewpoint for the scene using a
Camera
and a point in the scene.The position you view the scene from is defined by a
Camera
. The following properties of the camera are used to define an observation point in the scene:- 3D location: Latitude, longitude, and altitude
- Heading: Azimuth of the camera's direction
- Pitch: Up and down angle
- Roll: Side-to-side angle
SceneViewModel.csUse dark colors for code blocks // Apply the surface to the scene. scene.BaseSurface = elevationSurface; // Create a point that defines the observer's (camera) initial location in the scene. // The point defines a longitude, latitude, and altitude of the initial camera location. MapPoint cameraLocation = new MapPoint(-118.804, 33.909, 5330.0, SpatialReferences.Wgs84); // Create a Camera using the point, the direction the camera should face (heading), and its pitch and roll (rotation and tilt). Camera sceneCamera = new Camera(locationPoint: cameraLocation, heading: 355.0, pitch: 72.0, roll: 0.0); // Create the initial point to center the camera on (the Santa Monica mountains in Southern California). // Longitude=118.805 degrees West, Latitude=34.027 degrees North MapPoint sceneCenterPoint = new MapPoint(-118.805, 34.027, SpatialReferences.Wgs84); // Set an initial viewpoint for the scene using the camera and observation point. Viewpoint initialViewpoint = new Viewpoint(sceneCenterPoint, sceneCamera); scene.InitialViewpoint = initialViewpoint;
-
Set the
Scene
property with theView Model.Scene scene
you've created.SceneViewModel.csUse dark colors for code blocks // Set an initial viewpoint for the scene using the camera and observation point. Viewpoint initialViewpoint = new Viewpoint(sceneCenterPoint, sceneCamera); scene.InitialViewpoint = initialViewpoint; // Set the view model "Scene" property. this.Scene = scene;
-
Add a constructor to the class that calls
Setup
when a newScene Scene
is created.View Model When code like
Scene
runs, the class constructor also runs. This is a good place to add code that needs to run whenever the class is initialized.View Model new Scene VM = new Scene View Model(); SceneViewModel.csUse dark colors for code blocks namespace DisplayAScene { class SceneViewModel : INotifyPropertyChanged { public SceneViewModel() { SetupScene(); }
Your Scene
is complete!
An advantage to using the MVVM design pattern is that you can reuse code from a view model
. Because this API has a nearly-standard API surface across platforms, a view model
written for one app typically works on all supported .NET platforms.
Next, set up a view
in your project to consume the view model
.
Add a scene view
A SceneView
control is used to display a Scene
. You will add a scene view to your project UI and wire it up to consume the scene that is defined on Scene
.
-
Add required XML namespace and resource declarations.
- Open
Main
and switch to the XAML viewWindow.xaml - Inside the existing namespace declarations, add an
esri
XML namespace for the ArcGIS controls - Add XAML that defines a
Scene
instance as a static resourceView Model
MainWindow.xamlUse dark colors for code blocks <Window x:Class="DisplayAScene.MainWindow" xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:d="http://schemas.microsoft.com/expression/blend/2008" xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006" xmlns:local="clr-namespace:DisplayAScene" xmlns:esri="http://schemas.esri.com/arcgis/runtime/2013" mc:Ignorable="d" Title="MainWindow" Height="450" Width="800"> <Window.Resources> <local:SceneViewModel x:Key="SceneViewModel" /> </Window.Resources>
- Open
-
Add a
SceneView
control toMain
and bind it to theWindow.xaml Scene
.View Model - Add XAML that defines a
Scene
control namedView Main
Scene View - Use data binding to set the
Scene
property of the control using theScene
resourceView Model
MainWindow.xamlUse dark colors for code blocks <Grid> <esri:SceneView x:Name="MainSceneView" Scene="{Binding Scene, Source={StaticResource SceneViewModel}}" /> </Grid>
- Add XAML that defines a
Run the app
Click Debug > Start Debugging (or press <F5> on the keyboard) to run the app.
You should see a scene with the topographic basemap layer centered on the Santa Monica Mountains in California. Double-click, drag, and scroll the mouse wheel over the scene view to explore the scene.
What's next?
Learn how to use additional API features, ArcGIS location services, and ArcGIS tools in these tutorials: