Learn how to find a route and directions with the route service.
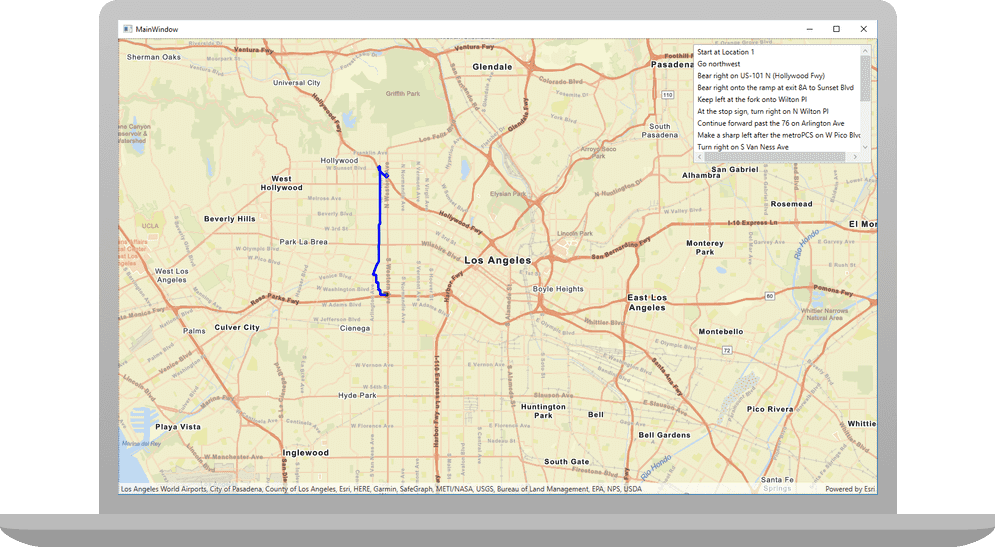
Routing is the process of finding the path from an origin to a destination in a street network. You can use the Routing service to find routes, get driving directions, calculate drive times, and solve complicated, multiple vehicle routing problems. To create a route, you typically define a set of stops (origin and one or more destinations) and use the service to find a route with directions. You can also use a number of additional parameters such as barriers and mode of travel to refine the results.
In this tutorial, you define an origin and destination by clicking on the map. These values are used to get a route and directions from the route service. The directions are also displayed on the map.
Prerequisites
Before starting this tutorial:
-
You need an ArcGIS Location Platform or ArcGIS Online account.
-
Ensure your development environment meets the system requirements.
Optionally, you may want to install the ArcGIS Maps SDK for .NET to get access to project templates in Visual Studio (Windows only) and offline copies of the NuGet packages.
Steps
Get an access token
You need an access token to use the location services used in this tutorial.
-
Go to the Create an API key tutorial to obtain an access token.
-
Ensure that the following privileges are enabled: Location services > Basemaps > Basemap styles service and Location services > Routing.
-
Copy the access token as it will be used in the next step.
To learn more about other ways to get an access token, go to Types of authentication.
Open a Visual Studio solution
-
To start the tutorial, complete the Display a map tutorial or download and unzip the solution.
-
Open the
.sln
file in Visual Studio. -
In the Solution Explorer, click App.xaml.cs.
-
In the App class, add an override for the
O
function to set then Startup() Api
property onKey ArcGISRuntimeEnvironment
.App.xaml.csUse dark colors for code blocks public partial class App : Application { protected override void OnStartup(StartupEventArgs e) { base.OnStartup(e); Esri.ArcGISRuntime.ArcGISRuntimeEnvironment.ApiKey = "YOUR_ACCESS_TOKEN"; } } }
If you are developing with Visual Studio for Windows, ArcGIS Maps SDK for .NET provides a set of project templates for each supported .NET platform. These templates follow the Model-View-ViewModel (MVVM) design pattern. Install the ArcGIS Maps SDK for .NET Visual Studio Extension to add the templates to Visual Studio (Windows only). See Install and set up for details.
Update the tutorial name used in the project (optional)
The Visual Studio solution, project, and the namespace for all classes currently use the name Display
. Follow the steps below if you prefer the name to reflect the current tutorial. These steps are not required, your code will still work if you keep the original name.
The tutorial instructions and code use the name Find
for the solution, project, and namespace. You can choose any name you like, but it should be the same for each of these.
-
Update the name for the solution and the project.
- In Visual Studio, in the Solution Explorer, right-click the solution name and choose Rename. Provide the new name for your solution.
- In the Solution Explorer, right-click the project name and choose Rename. Provide the new name for your project.
-
Rename the namespace used by classes in the project.
- In the Solution Explorer, expand the project node.
- Double-click MapViewModel.cs in the Solution Explorer to open the file.
- In the
Map
class, double-click the namespace name (View Model Display
) to select it, and then right-click and choose Rename....AMap - Provide the new name for the namespace.
- Click Apply in the Rename: DisplayAMap window that appears in the upper-right of the code window. This will rename the namespace throughout your project.
-
Build the project.
- Choose Build > Build solution (or press <F6>).
Update the basemap and initial extent
For displaying routes and driving directions, a streets basemap usually works best. You will update the Map
to use the ArcGISStreets
basemap and center the display on Los Angeles, California.
-
In the Visual Studio > Solution Explorer, double-click MainWindow.xaml.cs to open the file.
A view defined with XAML, such as
Main
, is composed of two files in a Visual Studio project. The visual elements (such as map views, buttons, text boxes, and so on) are defined with XAML markup in aWindow .xaml
file. This file is often referred to as the "page". The code for the XAML elements is stored in an associated.xaml.cs
file that is known as the "code-behind", since it stores the code behind the controls. Keeping with the MVVM design pattern, user interface events (such as the code that responds to a button click) are handled in the code-behind for the view. -
Update the
Viewpoint
that theMapView
initially shows when the app initializes. This viewpoint will show an area around Los Angeles, California.MainWindow.xaml.csUse dark colors for code blocks public MainWindow() { InitializeComponent(); MapPoint mapCenterPoint = new MapPoint(-118.24532, 34.05398, SpatialReferences.Wgs84); MainMapView.SetViewpoint(new Viewpoint(mapCenterPoint, 144_447.638572)); }
-
In the Visual Studio > Solution Explorer, double-click MapViewModel.cs to open the file.
The project uses the Model-View-ViewModel (MVVM) design pattern to separate the application logic (view model) from the user interface (view).
Map
contains the view model class for the application, calledView Model.cs Map
. See the Microsoft documentation for more information about the Model-View-ViewModel pattern.View Model -
In the
Setup
function, update theMap() Map
to use theBasemap
basemap.Style.ArcGISStreets MapViewModel.csUse dark colors for code blocks private void SetupMap() { Map = new Map(BasemapStyle.ArcGISStreets);
-
Click Debug > Start Debugging (or press <F5> on the keyboard) to run the app.
You should see a streets map centered on an area of Los Angeles.
Define member variables
You will need member variables in the Map
class to store the status of user-defined route stops and several Graphic
objects for displaying route results.
-
Add additional required
using
statements to the top of theMap
class.View Model Classes in the
Esri.ArcGISRuntime.Tasks.NetworkAnalysis
namespace provide network analysis functionality, such as solving routes, finding closest facilities, or defining service areas on a street network.Esri.ArcGISRuntime.UI
contains theGraphic
andGraphicsOverlay
classes.Esri.ArcGISRuntime.Symbology
contains the classes that define the symbols and renderers used to display features and graphics.MapViewModel.csUse dark colors for code blocks using System; using System.Collections.Generic; using System.ComponentModel; using System.Runtime.CompilerServices; using System.Text; using Esri.ArcGISRuntime.Geometry; using Esri.ArcGISRuntime.Mapping; using System.Linq; using System.Threading.Tasks; using Esri.ArcGISRuntime.Symbology; using Esri.ArcGISRuntime.Tasks.NetworkAnalysis; using Esri.ArcGISRuntime.UI;
-
Create the enum with values that represent the three possible contexts for clicks on the map. Add a private variable to track the current click state.
The user will click the map to define the origin and destination locations for the route. If neither stop has been defined, a map click will be used for the origin ("from") location. If an origin is defined, the click will be used to define the destination ("to") location. If both are defined, you may choose to start over and use the click to define a new origin location. This enum has values that represent the context of these clicks so the code can respond appropriately.
MapViewModel.csUse dark colors for code blocks namespace FindARouteAndDirections { class MapViewModel : INotifyPropertyChanged { enum RouteBuilderStatus { NotStarted, // No locations have been defined. SelectedStart, // Origin point exists. SelectedStartAndEnd // Origin and destination exist. } private RouteBuilderStatus _currentState = RouteBuilderStatus.NotStarted;
-
Create additional member variables for the route result graphics. These graphics will show the stops (origin and destination locations) and the route result polyline that connects them.
MapViewModel.csUse dark colors for code blocks enum RouteBuilderStatus { NotStarted, // No locations have been defined. SelectedStart, // Origin point exists. SelectedStartAndEnd // Origin and destination exist. } private RouteBuilderStatus _currentState = RouteBuilderStatus.NotStarted; private Graphic _startGraphic = new(); private Graphic _endGraphic = new(); private Graphic _routeGraphic = new();
Add properties for graphics overlays and driving directions
A graphics overlay is a container for graphics that are always displayed on top of all the other layers in the map. You will use graphics overlays to display graphics for a Route
and its stops.
A Route
provides turn-by-turn directions with its DirectionManeuvers
property. You will expose the directions with a property in Map
so they can be bound to a control in the app's UI.
-
In the view model, create a new property named
Graphics
. This will be a collection ofOverlays GraphicsOverlay
that will store point, line, and polygon graphics.MapViewModel.csUse dark colors for code blocks private Map? _map; public Map? Map { get { return _map; } set { _map = value; OnPropertyChanged(); } } private GraphicsOverlayCollection? _graphicsOverlayCollection; public GraphicsOverlayCollection? GraphicsOverlays { get { return _graphicsOverlayCollection; } set { _graphicsOverlayCollection = value; OnPropertyChanged(); } }
-
Create a property in
Map
that exposes a list of driving directions for aView Model Route
.MapViewModel.csUse dark colors for code blocks private GraphicsOverlayCollection? _graphicsOverlayCollection; public GraphicsOverlayCollection? GraphicsOverlays { get { return _graphicsOverlayCollection; } set { _graphicsOverlayCollection = value; OnPropertyChanged(); } } private List<string>? _directions; public List<string>? Directions { get { return _directions; } set { _directions = value; OnPropertyChanged(); } }
Create route graphics
You will define the symbology for each Route
result Graphic
and add them to a GraphicsOverlay
.
-
Create a new
GraphicsOverlay
to contain graphics for theRoute
result and stops. Create aGraphicsOverlayCollection
that contains the overlay and use it to set theMap
property.View Model.Graphics Overlays MapViewModel.csUse dark colors for code blocks private void SetupMap() { Map = new Map(BasemapStyle.ArcGISStreets); GraphicsOverlay routeAndStopsOverlay = new GraphicsOverlay(); this.GraphicsOverlays = new GraphicsOverlayCollection { routeAndStopsOverlay };
-
Create each result
Graphic
and define itsSymbol
.The
Geometry
for each graphic is initiallynull
and will not display until a valid geometry is assigned (for example, when the user clicks to define a stop location).MapViewModel.csUse dark colors for code blocks GraphicsOverlay routeAndStopsOverlay = new GraphicsOverlay(); this.GraphicsOverlays = new GraphicsOverlayCollection { routeAndStopsOverlay }; var startOutlineSymbol = new SimpleLineSymbol(SimpleLineSymbolStyle.Solid, System.Drawing.Color.Blue, 2); _startGraphic = new Graphic(null, new SimpleMarkerSymbol { Style = SimpleMarkerSymbolStyle.Diamond, Color = System.Drawing.Color.Orange, Size = 8, Outline = startOutlineSymbol } ); var endOutlineSymbol = new SimpleLineSymbol(style: SimpleLineSymbolStyle.Solid, color: System.Drawing.Color.Red, width: 2); _endGraphic = new Graphic(null, new SimpleMarkerSymbol { Style = SimpleMarkerSymbolStyle.Square, Color = System.Drawing.Color.Green, Size = 8, Outline = endOutlineSymbol } ); _routeGraphic = new Graphic(null, new SimpleLineSymbol( style: SimpleLineSymbolStyle.Solid, color: System.Drawing.Color.Blue, width: 4 ));
-
Add the graphics to the
GraphicsOverlay
.MapViewModel.csUse dark colors for code blocks _routeGraphic = new Graphic(null, new SimpleLineSymbol( style: SimpleLineSymbolStyle.Solid, color: System.Drawing.Color.Blue, width: 4 )); routeAndStopsOverlay.Graphics.AddRange(new [] { _startGraphic, _endGraphic, _routeGraphic });
-
Create a function to reset the current route creation state. The function will set the
Geometry
for each resultGraphic
tonull
, clear driving directions text from theMap
property, and set the status variable toView Model.Directions Route
.Builder Status.Not Started MapViewModel.csUse dark colors for code blocks routeAndStopsOverlay.Graphics.AddRange(new [] { _startGraphic, _endGraphic, _routeGraphic }); } private void ResetState() { _startGraphic.Geometry = null; _endGraphic.Geometry = null; _routeGraphic.Geometry = null; Directions = null; _currentState = RouteBuilderStatus.NotStarted; }
Find a route between two stops
You will create a function that uses the route service to find the best route between two stops.
-
Create a new asynchronous function called
Find
.Route() When calling methods asynchronously inside a function (using the
await
keyword), theasync
keyword is required in the signature.Asynchronous functions that don't return a value should have a return type of
Task
. Although avoid
return type would continue to work, this is not considered best practice. Exceptions thrown by anasync void
method cannot be caught outside of that method, are difficult to test, and can cause serious side effects if the caller is not expecting them to be asynchronous. The only circumstance whereasync void
is acceptable is when using an event handler, such as a button click.See the Microsoft documentation for more information about Asynchronous programming with async and await.
MapViewModel.csUse dark colors for code blocks private void ResetState() { _startGraphic.Geometry = null; _endGraphic.Geometry = null; _routeGraphic.Geometry = null; Directions = null; _currentState = RouteBuilderStatus.NotStarted; } public async Task FindRoute() { }
-
Create route
Stop
objects using the start and end graphics' geometry.MapViewModel.csUse dark colors for code blocks public async Task FindRoute() { if(_startGraphic.Geometry == null || _endGraphic.Geometry == null) return; var stops = new [] { _startGraphic, _endGraphic }.Select(graphic => { var geometry = graphic.Geometry as MapPoint; return new Stop(geometry!); });
-
Create a new
RouteTask
that uses the route service. CreateRouteParameters
,SetStops
and request toReturnRoutes
andReturnDirections
with the result.MapViewModel.csUse dark colors for code blocks if(_startGraphic.Geometry == null || _endGraphic.Geometry == null) return; var stops = new [] { _startGraphic, _endGraphic }.Select(graphic => { var geometry = graphic.Geometry as MapPoint; return new Stop(geometry!); }); var routeTask = await RouteTask.CreateAsync(new Uri("https://route-api.arcgis.com/arcgis/rest/services/World/Route/NAServer/Route_World")); RouteParameters parameters = await routeTask.CreateDefaultParametersAsync(); parameters.SetStops(stops); parameters.ReturnDirections = true; parameters.ReturnRoutes = true;
-
Use the
RouteTask
to solve the route. If theRouteResult
contains aRoute
, set the route graphic geometry with the result geometry, set theMap
property with theView Model.Directions DirectionManeuvers
, and reset the current state. If no route was found, reset the state and throw an exception to alert the user.MapViewModel.csUse dark colors for code blocks var routeTask = await RouteTask.CreateAsync(new Uri("https://route-api.arcgis.com/arcgis/rest/services/World/Route/NAServer/Route_World")); RouteParameters parameters = await routeTask.CreateDefaultParametersAsync(); parameters.SetStops(stops); parameters.ReturnDirections = true; parameters.ReturnRoutes = true; var result = await routeTask.SolveRouteAsync(parameters); if (result?.Routes?.FirstOrDefault() is Route routeResult) { _routeGraphic.Geometry = routeResult.RouteGeometry; Directions = routeResult.DirectionManeuvers.Select(maneuver => maneuver.DirectionText).ToList(); _currentState = RouteBuilderStatus.NotStarted; } else { ResetState(); throw new Exception("Route not found"); }
Handle user taps on the map
The user will tap (or click) locations on the map to define start and end points for the route. The MapView
control will accept the user input but the Map
class will contain the logic to handle tap locations and to define stops for solving the route.
-
Create a function in
Map
to handleView Model MapPoint
input from the user. Depending on the currentRoute
, the point sets the geometry for the start or end location graphic. If the current point defines the end location, a call toBuilder Status Find
solves the route.Route() MapViewModel.csUse dark colors for code blocks if (result?.Routes?.FirstOrDefault() is Route routeResult) { _routeGraphic.Geometry = routeResult.RouteGeometry; Directions = routeResult.DirectionManeuvers.Select(maneuver => maneuver.DirectionText).ToList(); _currentState = RouteBuilderStatus.NotStarted; } else { ResetState(); throw new Exception("Route not found"); } } public async Task HandleTap(MapPoint tappedPoint) { switch (_currentState) { case RouteBuilderStatus.NotStarted: ResetState(); _startGraphic.Geometry = tappedPoint; _currentState = RouteBuilderStatus.SelectedStart; break; case RouteBuilderStatus.SelectedStart: _endGraphic.Geometry = tappedPoint; _currentState = RouteBuilderStatus.SelectedStartAndEnd; await FindRoute(); break; case RouteBuilderStatus.SelectedStartAndEnd: // Ignore map clicks while routing is in progress break; } }
-
In the Visual Studio > Solution Explorer, double-click MainWindow.xaml.cs to open the file.
-
Add a handler for the
GeoView.GeoViewTapped
event. The event will fire when the user taps (or clicks) theMapView
. The tapped location will be passed toMap
.View Model.Handle Tap() Asynchronous functions should return
Task
orTask<
. For event handlers, such as theT> Button.Click
handler, the function must returnvoid
to conform to the delegate signature. See the Microsoft documentation regarding Async return types.MainWindow.xaml.csUse dark colors for code blocks public MainWindow() { InitializeComponent(); MapPoint mapCenterPoint = new MapPoint(-118.24532, 34.05398, SpatialReferences.Wgs84); MainMapView.SetViewpoint(new Viewpoint(mapCenterPoint, 144_447.638572)); } public async void MainMapView_GeoViewTapped(object sender, GeoViewInputEventArgs e) { try { var viewmodel = Resources["MapViewModel"] as MapViewModel; if (viewmodel != null && e?.Location != null) { await viewmodel.HandleTap(e.Location); } } catch (Exception ex) { MessageBox.Show("Error", ex.Message); } }
Bind UI elements to the view model
Map
has properties for graphics overlays (Map
) and driving directions (Map
) that must be bound to UI elements to be presented to the user. The GeoView.GeoViewTapped
event must also be handled so the view model can accept map locations from the user.
-
In the Visual Studio > Solution Explorer, double-click MainWindow.xaml to open the file.
-
Use data binding to bind the
Graphics
property of theOverlays Map
to theView Model MapView
control. Handle theGeo
event with theView Tapped Main
function.M a p View_ Geo View Tapped Data binding and the Model-View-ViewModel (MVVM) design pattern allow you to separate the logic in your app (the view model) from the presentation layer (the view). Graphics can be added and removed from the graphics overlays in the view model, and those changes appear in the map view through data binding.
MainWindow.xamlUse dark colors for code blocks <Window.Resources> <local:MapViewModel x:Key="MapViewModel" /> </Window.Resources> <Grid> <esri:MapView x:Name="MainMapView" Map="{Binding Map, Source={StaticResource MapViewModel}}" GraphicsOverlays="{Binding GraphicsOverlays, Source={StaticResource MapViewModel}}" GeoViewTapped="MainMapView_GeoViewTapped" />
-
Add a
List
element and bind itsView Item
to theSource Map
property. When a route is successfully solved, the turn-by-turn directions will appear here.View Model.Directions MainWindow.xamlUse dark colors for code blocks <Border Background="White" BorderBrush="Black" Margin="10" HorizontalAlignment="Right" VerticalAlignment="Top" Width="300" Height="200"> <ListView ItemsSource="{Binding Directions, Mode=OneWay, Source={StaticResource MapViewModel}}" />
Run the app
-
Click Debug > Start Debugging (or press <F5> on the keyboard) to run the app.
Clicks on the map should alternate between creating an origin and destination point. When both points are defined, the route task uses the route service to find the best route and provide turn-by-turn directions.
What's next?
To explore more API features and ArcGIS location services, try the following tutorial: