Learn how to display a map from a mobile map package (MMPK).
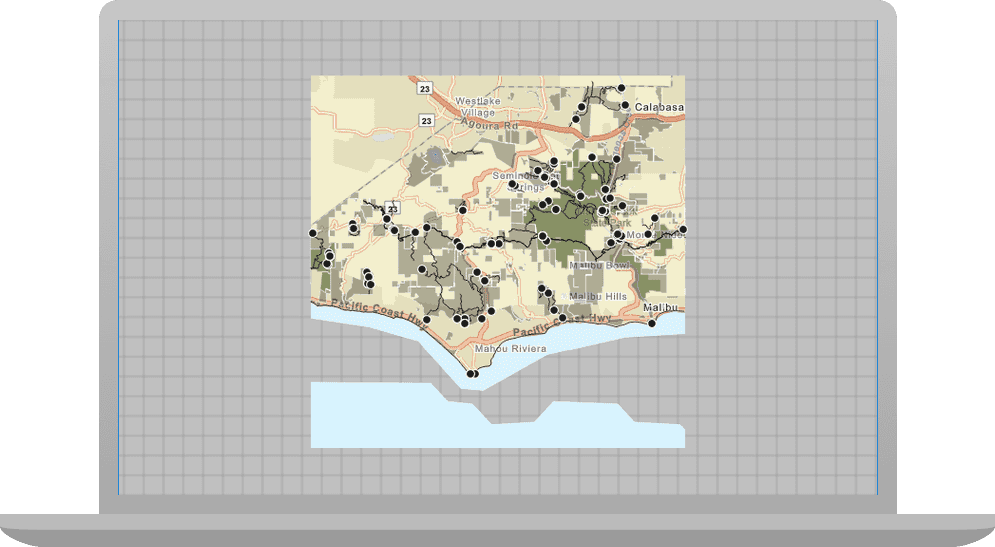
In this tutorial you will display a fully interactive map from a mobile map package (MMPK). The map contains a basemap layer and data layers and does not require a network connection.
Prerequisites
Before starting this tutorial:
-
You need an ArcGIS Location Platform or ArcGIS Online account.
-
Ensure your development environment meets the system requirements.
Optionally, you may want to install the ArcGIS Maps SDK for .NET to get access to project templates in Visual Studio (Windows only) and offline copies of the NuGet packages.
Steps
Open a Visual Studio solution
- To start the tutorial, complete the Display a map tutorial or download and unzip the solution.
- Open the
.sln
file in Visual Studio.
If you are developing with Visual Studio for Windows, ArcGIS Maps SDK for .NET provides a set of project templates for each supported .NET platform. These templates follow the Model-View-ViewModel (MVVM) design pattern. Install the ArcGIS Maps SDK for .NET Visual Studio Extension to add the templates to Visual Studio (Windows only). See Install and set up for details.
Update the tutorial name used in the project (optional)
The Visual Studio solution, project, and the namespace for all classes currently use the name Display
. Follow the steps below if you prefer the name to reflect the current tutorial. These steps are not required, your code will still work if you keep the original name.
The tutorial instructions and code use the name Display
for the solution, project, and namespace. You can choose any name you like, but it should be the same for each of these.
-
Update the name for the solution and the project.
- In Visual Studio, in the Solution Explorer, right-click the solution name and choose Rename. Provide the new name for your solution.
- In the Solution Explorer, right-click the project name and choose Rename. Provide the new name for your project.
-
Rename the namespace used by classes in the project.
- In the Solution Explorer, expand the project node.
- Double-click MapViewModel.cs in the Solution Explorer to open the file.
- In the
Map
class, double-click the namespace name (View Model Display
) to select it, and then right-click and choose Rename....AMap - Provide the new name for the namespace.
- Click Apply in the Rename: DisplayAMap window that appears in the upper-right of the code window. This will rename the namespace throughout your project.
-
Build the project.
- Choose Build > Build solution (or press <F6>).
Add a mobile map package to the project
Add a mobile map package (.mmpk) for distribution with your app.
-
Create or download the
Mahou
mobile map package. Complete the Create a mobile map package tutorial to create the package yourself. Otherwise, download the solution file: MahouRivieraTrails.mmpk.Riviera Trails.mmpk -
From the Visual Studio Project menu, choose Add > Existing item ....
-
Navigate to the folder that contains your mobile map package. Change the file type in the dialog to
All files (*.*)
, chooseMahou
, then click Add to add it to the project.Riviera Trails.mmpk -
In the Visual Studio Solution Explorer, select
Mahou
. In the Properties window, set the following properties of the file.Riviera Trails.mmpk - Build Action:
Content
- Copy to Output Directory:
Copy always
- Build Action:
Open the mobile map package and display a map
Select a map from the maps contained in a mobile map package and display it in a map view. Use the MobileMapPackage
class to access the mobile map package and load it to read its contents.
-
In the Visual Studio > Solution Explorer, double-click MapViewModel.cs to open the file.
-
Add additional required
using
statements at the top of the class.MapViewModel.csUse dark colors for code blocks using System; using System.Collections.Generic; using System.Text; using Esri.ArcGISRuntime.Geometry; using Esri.ArcGISRuntime.Mapping; using System.ComponentModel; using System.Runtime.CompilerServices; using System.Linq; using System.Threading.Tasks;
-
In the MapViewModel class, remove all the existing code in the
Setup
function.Map() MapViewModel.csUse dark colors for code blocks private void SetupMap() { // Create a new map with a 'topographic vector' basemap. Map = new Map(BasemapStyle.ArcGISTopographic); }
-
Modify the signature of the
Setup
function to include theMap() async
keyword and to returnTask
rather thanvoid
.MapViewModel.csUse dark colors for code blocks private async Task SetupMap() { }
When calling methods asynchronously inside a function (using the
await
keyword), theasync
keyword is required in the signature.Although a
void
return type would continue to work, this is not considered best practice. Exceptions thrown by anasync void
method cannot be caught outside of that method, are difficult to test, and can cause serious side effects if the caller is not expecting them to be asynchronous. The only circumstance whereasync void
is acceptable is when using an event handler, such as a button click.See the Microsoft documentation for more information about Asynchronous programming with async and await.
-
(Optional) Modify the call to
Setup
(in theMap() Map
constructor) to avoid a compilation warning. After changingView Model Setup
to an asynchronous method, the following warning appears in the Visual Studio Error List.Map() Use dark colors for code blocks Copy Because this call is not awaited, execution of the current method continues before the call is completed. Consider applying the 'await' operator to the result of the call.
Because your code does not anticipate a return value from this call, the warning can be ignored. Your code will run despite the warning. But if you want to be more specific about your intentions with this call and to address the warning, add the following code to store the return value in a discard.
MapViewModel.csUse dark colors for code blocks public MapViewModel() { _ = SetupMap(); }
From the Microsoft documentation:
"[Discards] are placeholder variables that are intentionally unused in application code. Discards are equivalent to unassigned variables; they don't have a value. A discard communicates intent to the compiler and others that read your code: You intended to ignore the result of an expression."
-
Add the following code to the
Setup
function. This code defines the path to the mobile map package relative to the app, creates the mobile map package using theMap() MobileMapPackage
constructor, loads the mobile map package asynchronously, and adds the first map in the mobile map package to theMapView
.Use dark colors for code blocks // Define the path to the mobile map package. string pathToMobileMapPackage = System.IO.Path.Combine(Environment.CurrentDirectory, @"MahouRivieraTrails.mmpk"); // Instantiate a new mobile map package. MobileMapPackage mahouRivieraTrails_MobileMapPackage = new MobileMapPackage(pathToMobileMapPackage); // Load the mobile map package. await mahouRivieraTrails_MobileMapPackage.LoadAsync(); // Show the first map in the mobile map package. this.Map = mahouRivieraTrails_MobileMapPackage.Maps.FirstOrDefault();
A
MobileMapPackage
can contain many maps in aMaps
array.Loading the mobile map package is an asynchronous process. The file is read on a thread that does not block the UI.
-
Click Debug > Start Debugging (or press <F5> on the keyboard) to run the app.
You will see a map of trailheads, trails, and parks for the area south of the Santa Monica mountains. You can pinch (to zoom and rotate), drag, and double-tap the map view to explore the map.
What's next?
Learn how to use additional API features, ArcGIS location services, and ArcGIS tools in these tutorials: