Learn how to download and display an offline map for a user-defined geographical area of a web map.
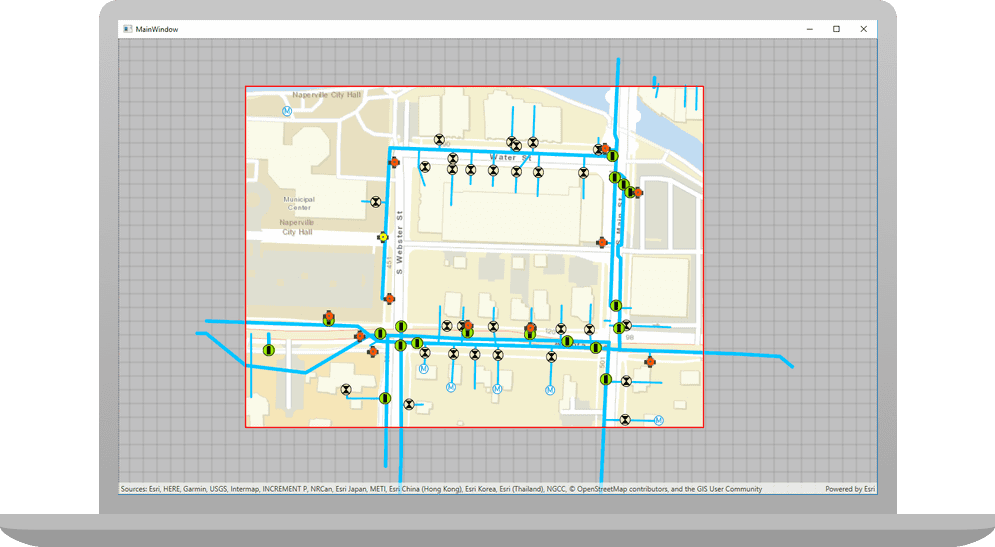
Offline maps allow users to continue working when network connectivity is poor or lost. If a web map is enabled for offline use, a user can request that ArcGIS generates an offline map for a specified geographic area of interest.
In this tutorial, you will download an offline map for an area of interest from the web map of the stormwater network within Naperville, IL, USA . You can then use this offline map without a network connection.
Before starting this tutorial:
-
You need an ArcGIS Location Platform or ArcGIS Online account.
-
Ensure your development environment meets the system requirements.
Optionally, you may want to install the ArcGIS Maps SDK for .NET to get access to project templates in Visual Studio (Windows only) and offline copies of the NuGet packages.
Steps
Open a Visual Studio solution
-
To start the tutorial, complete the Display a web map tutorial or download and unzip the solution.
-
Open the
.sln
file in Visual Studio. -
If you downloaded the solution, get an access token and set the API key.
An API Key gives your app access to secure resources used in this tutorial.
-
Go to the Create an API key tutorial to obtain a new API key access token. Ensure that the following privilege is enabled: Location services > Basemaps > Basemap styles service. Copy the access token as it will be used in the next step.
-
In Visual Studio, in the Solution Explorer, click App.xaml.cs.
-
In the App class, add an override for the
O
function to set then Startup() Api
property onKey ArcGISRuntimeEnvironment
.App.xaml.csUse dark colors for code blocks public partial class App : Application { protected override void OnStartup(StartupEventArgs e) { base.OnStartup(e); Esri.ArcGISRuntime.ArcGISRuntimeEnvironment.ApiKey = "YOUR_ACCESS_TOKEN"; } } }
-
If you are developing with Visual Studio for Windows, ArcGIS Maps SDK for .NET provides a set of project templates for each supported .NET platform. These templates follow the Model-View-ViewModel (MVVM) design pattern. Install the ArcGIS Maps SDK for .NET Visual Studio Extension to add the templates to Visual Studio (Windows only). See Install and set up for details.
Update the tutorial name used in the project (optional)
The Visual Studio solution, project, and the namespace for all classes currently use the name Display
. Follow the steps below if you prefer the name to reflect the current tutorial. These steps are not required, your code will still work if you keep the original name.
The tutorial instructions and code use the name Display
for the solution, project, and namespace. You can choose any name you like, but it should be the same for each of these.
-
Update the name for the solution and the project.
- In Visual Studio, in the Solution Explorer, right-click the solution name and choose Rename. Provide the new name for your solution.
- In the Solution Explorer, right-click the project name and choose Rename. Provide the new name for your project.
-
Rename the namespace used by classes in the project.
- In the Solution Explorer, expand the project node.
- Double-click MapViewModel.cs in the Solution Explorer to open the file.
- In the
Map
class, double-click the namespace name (View Model Display
) to select it, and then right-click and choose Rename....AWeb Map - Provide the new name for the namespace.
- Click Apply in the Rename: DisplayAWebMap window that appears in the upper-right of the code window. This will rename the namespace throughout your project.
-
Build the project.
- Choose Build > Build solution (or press <F6>).
Get the web map item ID
You can use ArcGIS tools to create and view web maps. Use the Map Viewer to identify the web map item ID. This item ID will be used later in the tutorial.
- Go to the Naperville water network in the Map Viewer in ArcGIS Online. This web map displays stormwater network within Naperville, IL, USA .
- Make a note of the item ID at the end of the browser's URL. The item ID should be:
acc027394bc84c2fb04d1ed317aac674
Display the web map
You can display a web map using the web map's item ID. Create a map from the web map portal item, and display it in your app.
-
In the Visual Studio > Solution Explorer, double-click MapViewModel.cs to open the file.
The project uses the Model-View-ViewModel (MVVM) design pattern to separate the application logic (view model) from the user interface (view).
Map
contains the view model class for the application, calledView Model.cs Map
. See the Microsoft documentation for more information about the Model-View-ViewModel pattern.View Model -
Add additional required
using
statements at the top of the class.MapViewModel.csUse dark colors for code blocks using System; using System.Collections.Generic; using System.Text; using Esri.ArcGISRuntime.Geometry; using Esri.ArcGISRuntime.Mapping; using System.ComponentModel; using System.Runtime.CompilerServices; using System.Threading.Tasks; using Esri.ArcGISRuntime.Portal; using Esri.ArcGISRuntime.Symbology; using Esri.ArcGISRuntime.Tasks.Offline; using Esri.ArcGISRuntime.UI; using System.Windows; using System.Diagnostics; using System.Drawing;
-
In MapViewModel.cs, modify the
Setup
function to update the web map's item ID to that of the Naperville water network.Map() The existing code creates a
PortalItem
using the item ID and anArcGISPortal
referencing ArcGIS Online. It sets theMap
property to a newView Model.Map Map
created using thePortalItem
.MapViewModel.csUse dark colors for code blocks private async Task SetupMap() { // Create a portal pointing to ArcGIS Online. ArcGISPortal portal = await ArcGISPortal.CreateAsync(); // Create a portal item for a specific web map id. string webMapId = "acc027394bc84c2fb04d1ed317aac674"; PortalItem mapItem = await PortalItem.CreateAsync(portal, webMapId); // Create the map from the item. Map map = new Map(mapItem); // Set the view model "Map" property. this.Map = map;
-
Click Debug > Start Debugging (or press <F5> on the keyboard) to run the app.
You should see a map of the stormwater network within Naperville, IL, USA . Use the mouse to drag, scroll, and double-click the map view to explore the map.
Specify an area of the web map to take offline
You can specify an area of the web map to take offline using either an Envelope
or a Polygon
. You can use a graphic to display the area on the map.
-
In the MapViewModel class, create a new property named
Graphics
. This will be a collection ofOverlays GraphicsOverlay
to display a graphic of the area to take offline.Use dark colors for code blocks private Map? _map; public Map? Map { get { return _map; } set { _map = value; OnPropertyChanged(); } } private GraphicsOverlayCollection? _graphicsOverlays; public GraphicsOverlayCollection? GraphicsOverlays { get { return _graphicsOverlays; } set { _graphicsOverlays = value; OnPropertyChanged(); } }
-
Modify the
Setup
function. Use anMap() EnvelopeBuilder
to create a newEnvelope
that defines an area to take offline.MapViewModel.csUse dark colors for code blocks // Set the view model "Map" property. this.Map = map; // Define area of interest (envelope) to take offline. EnvelopeBuilder envelopeBldr = new EnvelopeBuilder(SpatialReferences.Wgs84) { XMin = -88.1526, XMax = -88.1490, YMin = 41.7694, YMax = 41.7714 }; Envelope offlineArea = envelopeBldr.ToGeometry();
-
Display a graphic of the area to take offline.
Use a
SimpleLineSymbol
and aSimpleFillSymbol
to display a newGraphic
of theoffline
with a red outline. Add the graphic to a newArea GraphicsOverlay
and set theMap
property to display it in the map view.View Model.Graphics Overlays MapViewModel.csUse dark colors for code blocks Envelope offlineArea = envelopeBldr.ToGeometry(); // Create a graphic to display the area to take offline. SimpleLineSymbol lineSymbol = new SimpleLineSymbol(SimpleLineSymbolStyle.Solid, Color.Red, 2); SimpleFillSymbol fillSymbol = new SimpleFillSymbol(SimpleFillSymbolStyle.Solid, Color.Transparent, lineSymbol); Graphic offlineAreaGraphic = new Graphic(offlineArea, fillSymbol); // Create a graphics overlay and add the graphic. GraphicsOverlay areaOverlay = new GraphicsOverlay(); areaOverlay.Graphics.Add(offlineAreaGraphic); // Add the overlay to a new graphics overlay collection. GraphicsOverlayCollection overlays = new GraphicsOverlayCollection { areaOverlay }; // Set the view model's "GraphicsOverlays" property (will be consumed by the map view). this.GraphicsOverlays = overlays;
-
In the Visual Studio > Solution Explorer, double-click MainWindow.xaml to open the file.
-
Use data binding to bind the
Graphics
property of theOverlays Map
to theView Model MapView
control.Data binding and the Model-View-ViewModel (MVVM) design pattern allow you to separate the logic in your app (the view model) from the presentation layer (the view).
Use dark colors for code blocks <esri:MapView x:Name="MainMapView" Map="{Binding Map, Source={StaticResource MapViewModel}}" GraphicsOverlays="{Binding GraphicsOverlays, Source={StaticResource MapViewModel}}" />
-
Click Debug > Start Debugging (or press <F5> on the keyboard) to run the app.
You should see a red outline on the stormwater network within Naperville, IL, USA . This indicates the area of the web map that you are going to take offline.
Download and display the offline map
You can generate and download an offline map for a specified area of interest using an asynchronous task. When complete, it will provide the offline map for display in your map view.
-
Return to the MapViewModel.cs file and add code to the
Setup
function to create anMap OfflineMapTask
that references the online map by calling the staticOfflineMapTask.CreateAsync
method.MapViewModel.csUse dark colors for code blocks // Set the view model's "GraphicsOverlays" property (will be consumed by the map view). this.GraphicsOverlays = overlays; // Create an offline map task using the current map. OfflineMapTask offlineMapTask = await OfflineMapTask.CreateAsync(map);
-
Get default parameters to generate and download the offline map. Modify them to download a read-only offline map.
This tutorial does not involve editing and updating the contents of the offline map. When an offline map is editable, metadata is stored in ArcGIS to track and synchronize edits. Setting the
GenerateOfflineMapUpdateMode
toN
avoids the overhead of maintaining this metadata in ArcGIS.o Updates MapViewModel.csUse dark colors for code blocks // Create an offline map task using the current map. OfflineMapTask offlineMapTask = await OfflineMapTask.CreateAsync(map); // Create a default set of parameters for generating the offline map from the area of interest. GenerateOfflineMapParameters parameters = await offlineMapTask.CreateDefaultGenerateOfflineMapParametersAsync(offlineArea); parameters.UpdateMode = GenerateOfflineMapUpdateMode.NoUpdates;
-
Set a download location for the offline map.
MapViewModel.csUse dark colors for code blocks // Create a default set of parameters for generating the offline map from the area of interest. GenerateOfflineMapParameters parameters = await offlineMapTask.CreateDefaultGenerateOfflineMapParametersAsync(offlineArea); parameters.UpdateMode = GenerateOfflineMapUpdateMode.NoUpdates; // Build a folder path named with today's date/time in the "My Documents" folder. string documentsFolder = Environment.GetFolderPath(Environment.SpecialFolder.MyDocuments); string downloadDirectory = System.IO.Path.Combine(documentsFolder, "OfflineMap_" + DateTime.Now.ToFileTime().ToString());
This tutorial code creates a new unique folder in the documents folder using the current date and time.
-
Create a new
GenerateOfflineMapJob
using theparameters
anddownload
.Directory MapViewModel.csUse dark colors for code blocks // Build a folder path named with today's date/time in the "My Documents" folder. string documentsFolder = Environment.GetFolderPath(Environment.SpecialFolder.MyDocuments); string downloadDirectory = System.IO.Path.Combine(documentsFolder, "OfflineMap_" + DateTime.Now.ToFileTime().ToString()); GenerateOfflineMapJob generateJob = offlineMapTask.GenerateOfflineMap(parameters, downloadDirectory);
-
Create a function called
Generate
. This function will respond when the job completes or fails and will track the precent complete as the job runs. Add aJ o b_ Progress Changed try/catch
block to handle exceptions and start by getting a reference to theGenerateOfflineMapJob
, passed in as thesender
argument.MapViewModel.csUse dark colors for code blocks // Build a folder path named with today's date/time in the "My Documents" folder. string documentsFolder = Environment.GetFolderPath(Environment.SpecialFolder.MyDocuments); string downloadDirectory = System.IO.Path.Combine(documentsFolder, "OfflineMap_" + DateTime.Now.ToFileTime().ToString()); GenerateOfflineMapJob generateJob = offlineMapTask.GenerateOfflineMap(parameters, downloadDirectory); } private async void GenerateJob_ProgressChanged(object? sender, EventArgs e) { try { var generateJob = sender as GenerateOfflineMapJob; if(generateJob == null) { return; } } catch (Exception ex) { MessageBox.Show($"Error generating offline map: {ex.Message}"); } }
-
If the job succeeds, set the
Map
property with the offline map result. If it fails, notify the user. If the job is running, write the percent complete to the console.View Model.Map MapViewModel.csUse dark colors for code blocks try { var generateJob = sender as GenerateOfflineMapJob; if(generateJob == null) { return; } // If the job succeeds, show the offline map in the map view. if (generateJob.Status == Esri.ArcGISRuntime.Tasks.JobStatus.Succeeded) { var result = await generateJob.GetResultAsync(); this.Map = result.OfflineMap; Debug.WriteLine("Generate offline map: Complete"); } // If the job fails, notify the user. else if (generateJob.Status == Esri.ArcGISRuntime.Tasks.JobStatus.Failed) { MessageBox.Show($"Unable to generate a map for that area: {generateJob?.Error?.Message}"); } else { int percentComplete = generateJob.Progress; Debug.WriteLine($"Generate offline map: {percentComplete}%"); } } catch (Exception ex) { MessageBox.Show($"Error generating offline map: {ex.Message}"); }
-
Return to your code in
Setup
and assign theMap Generate
function to handle theJ o b_ Progress Changed Job.ProgressChanged()
event.MapViewModel.csUse dark colors for code blocks GenerateOfflineMapJob generateJob = offlineMapTask.GenerateOfflineMap(parameters, downloadDirectory); generateJob.ProgressChanged += GenerateJob_ProgressChanged;
-
Start the
generate
by calling itsJob Job.Start()
method.MapViewModel.csUse dark colors for code blocks GenerateOfflineMapJob generateJob = offlineMapTask.GenerateOfflineMap(parameters, downloadDirectory); generateJob.ProgressChanged += GenerateJob_ProgressChanged; generateJob.Start(); }
-
Click Debug > Start Debugging (or press <F5> on the keyboard) to run the app.
You should see an offline map for the specified area of the stormwater network within Naperville, IL, USA . Remove your network connection and you will still be able to use the mouse to drag, scroll, and double-click the map view to explore this offline map.
What's next?
Learn how to use additional API features, ArcGIS location services, and ArcGIS tools in these tutorials: