Using a place name or street address as input, you can find locations of interest and interact with them on the map. The process of matching locations on the map to an address is referred to as geocoding. An online or local locator contains the logic for matching addresses, place names, or categories to geographic locations.
What is geocoding?
Geocoding is the process of inputting text for an address or place name to return a geographic location and additional address information (the complete address). For example, you can geocode "1600 Pennsylvania Ave, DC" to return its location (-77.03654 longitude, 38.89767 latitude) and a more complete address ("1600 Pennsylvania Avenue NW, Washington, DC 20500").
You can use geocoding to:
- Find the location of an address or place.
- Convert incomplete address text to a complete address.
- Provide a list of candidates for an incomplete address or place.
Find places
Place search, also known as point of interest (POI) search, uses a name or category to find geographic entities (such as businesses, administrative boundaries, and other features). For example, you can search for restaurants near a location, counties in a state, or land features such as the Grand Canyon.
There are two types of searches you can perform: local and global. A local search uses a location to prioritize results that are nearby or a search extent to confine the search area and restrict results by a defined boundary. A global search is an open search that typically isn't confined to an extent.
You can use place search to:
- Find the locations of places around the world.
- Locate businesses near a location.
- Search for places by category such as restaurants, gas stations, or schools.
- Find and display places on a map.
Suggest
Suggest functionality allows character-by-character autocomplete suggestions to be generated from user input in your geocoding application. This capability facilitates the interactive search experience by reducing the number of characters that need to be entered before a suggested match is obtained.
You can pass most of the same parameters to generate suggestions as you would pass when geocoding, such as preferred search location and category. Once a suitable auto-complete suggestion is returned, the suggest result can be passed directly to the geocoding operation. To optimize quality and performance you should pass the same parameters to suggest and to geocode.
You can use suggest to:
- Generate more complete input when the user is unsure of an exact place name or address.
- Minimize the number of characters a user needs to type to get a good result.
- Optimize quality and performance by passing a suggest result to geocode with the same parameters.
Reverse geocoding
Reverse geocoding is the process of interpolating a street address or identifying a place name for an input point. To refine the search, you can specify a feature type to return a specific type of address such as only POIs or addresses. You can also specify whether to return a street address or rooftop location.
The geocoding service uses the location and all parameters to return a single address, place, or intersection that is the closest match. This reverse geocoding result may contain a number of attributes such as the place name, full address, city, region, and location.
Reverse geocoding will always return the closest result, and the result may be an address, place, or intersection. If the locator you're using was built with only POI data, the result will always be a POI. If your locator was built to support multiple feature types, you can pass a feature type parameter to ensure that the result returned is of the desired type.
You can use reverse geocoding to:
- Get the nearest address, place, or intersection to your current location.
- Show an address or place name when you tap on a map.
- Find the nearest address, place, or intersection for a geographic location.
How geocoding works
Geocoding is implemented using an online or local locator. Specify an address, output data fields, and optionally, additional parameters to refine the search. The more complete you can make the input address (including a postal code, for example), the more likely the locator will find an exact match. To refine the search, you can provide additional parameters such as a search location, category, country code, and other parameters.
The locator parses the address and uses all of the parameters to return a set of address candidates. Each candidate contains a full address, location, attributes, and a score (0-100) that indicates how well it was matched based on the feature type of the locator used to make the match. Candidates are always returned in order from most to least exact irrespective of score; you never need to sort the candidates that are returned. Because score is relative to feature type, a slightly lower score on a Point
match will still be a better candidate than a higher score on a postal match, for instance. By default, the matched address, score, and location are returned with the geocode results. To return additional available data fields, set the output fields parameter in the geocode parameters.
For a place search, instead of passing a single-line address value as input, you can provide a place name or category. The geocode parameters allow you to prefer candidates that are closer to a specified location, which can be useful if searching for facilities near the current position. Depending on the data used to build your locator, you can return information about the locations, such as addresses, phone numbers, and URLs for candidates, as well as the distance from a reference location.
You can use geocode parameters to refine or restrict search results when geocoding place names just as you do with an address search.
Locator task
Geocoding, reverse geocoding, or place searches are implemented using a locator task. This task is configured with an online or local locator that contains the logic for matching addresses, place names, or categories to geographic locations.
You create a LocatorTask
by passing a URI (for an online locator) or a path (for a local locator) to the static LocatorTask.CreateAsync()
method on the class. The following example creates a new LocatorTask
that uses the Geocoding service.
// Provide an access token for your app (usually when the app starts).
// Esri.ArcGISRuntime.ArcGISRuntimeEnvironment.ApiKey = "YOUR_ACCESS_TOKEN";
var locatorTask = new LocatorTask(new Uri("https://geocode-api.arcgis.com/arcgis/rest/services/World/GeocodeServer"));
// You can also set an access token directly on the Locator Task:
// locatorTask.ApiKey = "YOUR_ACCESS_TOKEN";
var results = await locatorTask.GeocodeAsync("1600 Pennsylvania Ave NW, DC");
if (results?.FirstOrDefault() is GeocodeResult firstResult)
{
Console.WriteLine($"Found {firstResult.Label} at {firstResult.DisplayLocation} with score {firstResult.Score}");
}
Geocode parameters
When geocoding an address or place, you can optionally provide preferences for the geocoding operation. The following list describes some of the properties you can control:
- Country—The code for the country to which geocoding should be restricted.
- Maximum results—A limit for the maximum number of address candidates to return.
- Output language—The language (culture) in which result attributes will be returned (supported output languages will vary depending on how the locator was built.)
- Output spatial reference—The spatial reference in which geographic locations for address candidates will be returned.
- Result attributes—A list of field names to include in the results (available fields can be queried from the locator.)
- Preferred search location—A geographic point that prioritizes nearby locations as results.
Specify geocoding preferences using a GeocodeParameters
object.
// search within Japan and return results in Japanese
var geocodeParams = new GeocodeParameters
{
CountryCode = "Japan",
OutputLanguage = new System.Globalization.CultureInfo("ja-JP")
};
geocodeParams.ResultAttributeNames.Add("City");
Geocode results
Depending on how specific and complete the input address is, you may get several candidates from a geocode operation. Results are ordered by quality of the match and scored based on the quality of the match relative to the feature type of the result. The first candidate should always be considered as the best match. Additional candidate information can be obtained by specifying supplemental output fields to include in the results. For a complete list of fields available for the Geocoding service, see Output fields in the ArcGIS services reference. If you built a custom locator, your locator will contain the output fields that you designated in the locator properties as well as any custom output fields you may have created.
Examples
Local place name search by category
This example searches for restaurants near a location by setting the Mexican food
category near a location in southern California.
To see a full list of categories, visit Category filtering in the ArcGIS services reference.
Steps
-
Reference the Geocoding service.
-
Provide a point to center the search on.
-
Define the category of places to find.
-
Add to the list of data fields to return: Score and Distance
-
Set the API key.
// Provide an access token for your app (usually when the app starts).
// Esri.ArcGISRuntime.ArcGISRuntimeEnvironment.ApiKey = "YOUR_ACCESS_TOKEN";
var locatorTask = new LocatorTask(new Uri("https://geocode-api.arcgis.com/arcgis/rest/services/World/GeocodeServer"));
// You can also set an access token directly on the Locator Task:
// locatorTask.ApiKey = "YOUR_ACCESS_TOKEN";
var parameters = new GeocodeParameters();
parameters.Categories.Add("Mexican food");
parameters.PreferredSearchLocation = new MapPoint(
x: -118.20,
y: 34.001,
spatialReference: SpatialReferences.Wgs84
);
parameters.ResultAttributeNames.Add("Score");
parameters.ResultAttributeNames.Add("Distance");
var results = await locatorTask.GeocodeAsync("", parameters);
if (results?.FirstOrDefault() is GeocodeResult result)
{
Console.WriteLine($"Found {result.Label} at {result.DisplayLocation} with score {result.Score}");
}
The result is a collection of candidates, sorted by score, that include the distance from the input location.
Use API keys
An API Key access token can be used to authorize access to ArcGIS Online services and resources from your app, as well as to monitor access to those services. You can use a single access token for all requests made by your app, or assign individual access tokens for any classes that implements APIKeyResourceInterface
Tutorials
Samples

Find address

Find place
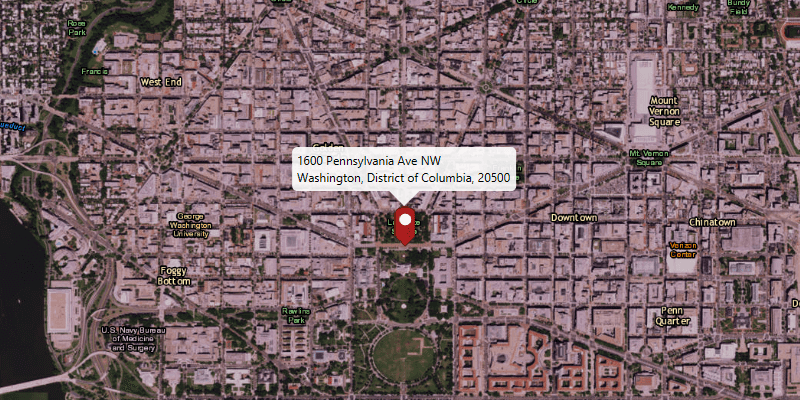
Reverse geocode
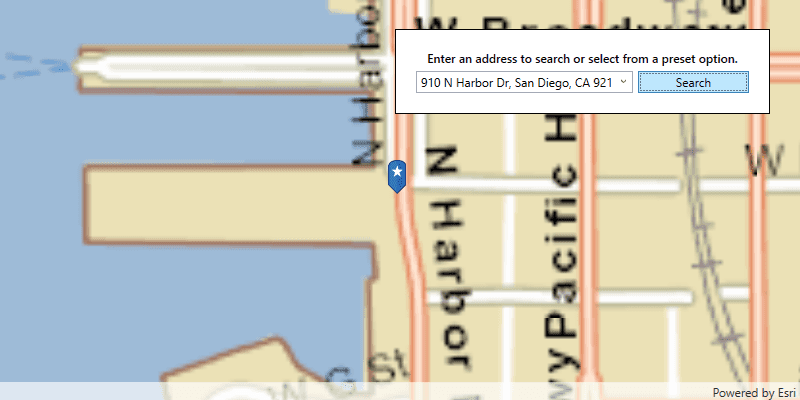
Offline geocode
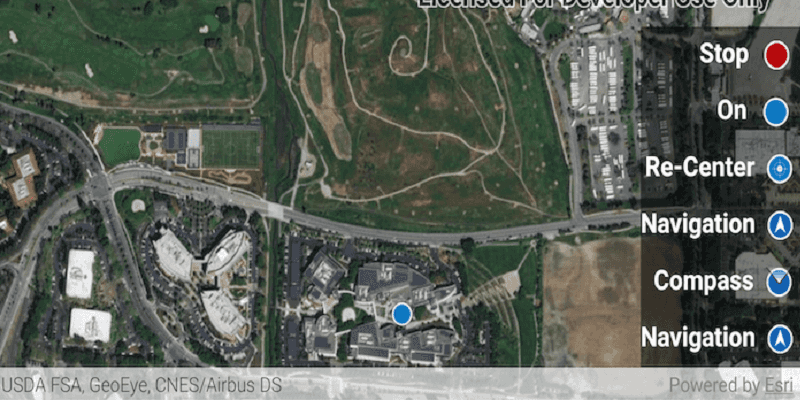
Display device location with autopan modes
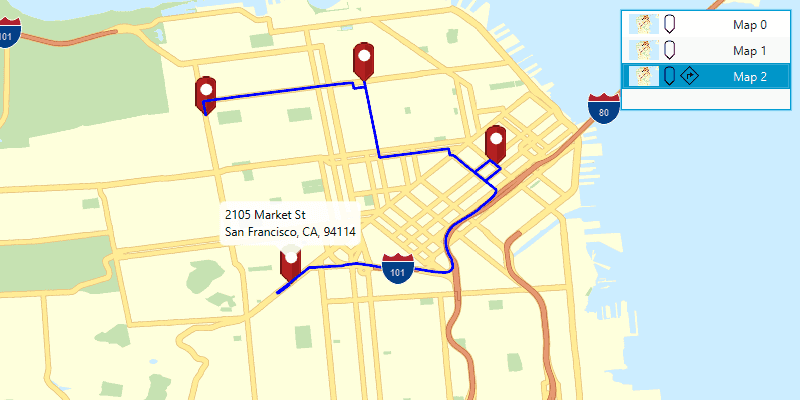