Symbol files, or program database files (.pdb), link instructions in compiled applications and libraries to statements in the original source code. They enable you to access a more informative call stack if that application or library fails. Call stacks are valuable because they enable the ArcGIS Maps SDK for .NET team to:
- Identify if the issue you are experiencing matches an existing issue in our backlog.
- Determine if we have reproduced the same issue you have in your environment.
- Gain more insight on where to look for the underlying problem.
If your UWP, WinUI, or WPF application crashes and the call stack points to the ArcGIS Maps SDK for .NET libraries, you can use the two workflows outlined below to provide Esri with more specific information and help us to more swiftly identify the cause of issue.
When debugging and working with symbol files you will see a distinction made between managed and native libraries ("modules"). Managed modules contain code whose execution is managed by the .NET Common Language Runtime. Native modules contain code whose execution is controlled by the developer of that library and as such is often referred to as unmanaged code.
UWP, WinUI, and WPF applications that you develop with ArcGIS Maps SDK for .NET will reference multiple managed and native libraries:
Esri.ArcGISRuntime.UWP.dll
orEsri.ArcGISRuntime.Win
orUI.dll Esri.ArcGISRuntime.WPF.dll
: A managed library containing the API types you reference.Esri.ArcGISRuntime.dll
(UWP, WinUI, and WPF): A managed library containing the API types you reference.Runtime
orCore Net.UWP.dll Runtime
orCore Net.Win UI.dll Runtime
: A native library containing functional references to the ArcGIS Maps SDK RuntimeCore library.Core Net200_0.WPF.dll Runtime
(UWP and WinUI) orCore Net.dll Runtime
(WPF): A native library containing functional references to the ArcGIS Maps SDK RuntimeCore library.Core Net200_0.dll runtime
: A native library containing the implementation for all ArcGIS Maps SDK runtime core capabilities.Core.dll
Add a reference to the Esri symbol server
- In Visual Studio, open the Options dialog (Tools > Options).
- In the Options dialog, expand Debugging and choose Symbols.
- Click the + button to add a new symbol file (.pdb) location.
- Enter the Esri symbol server URL: https://downloads2.esri.com/support/symbols.
- Ensure the checkbox is checked next to the new URL in the list of Symbol file (.pdb) locations and click OK to close the Options dialog.
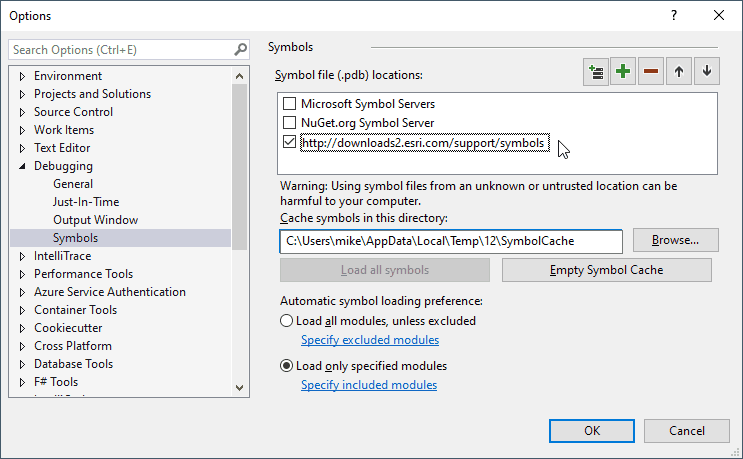
For more information see Specify symbol (.pdb) and source files in the Visual Studio debugger in the Microsoft documentation.
The following workflows provide an overview of the steps for debugging WPF applications. For UWP applications, follow the same workflow and load the equivalent UWP module reference where appropriate.
Workflow 1: Debug a WPF application
Follow these steps if you can reproduce the problem when running your application with the debugger attached.
- In Visual Studio, click Start Debugging (or tap F5).
- Open the Modules window by choosing Debug > Windows > Modules.
- Locate the module
Esri.ArcGISRuntime.dll
then right click and choose Load Symbols. - Ensure the Symbol Status for the module is Symbols loaded.
- Locate the module
Esri.ArcGISRuntime.WPF.dll
then right click and choose Load Symbols. - Ensure the Symbol Status for the module is Symbols loaded.
- Run through the workflow that reproduces the problem.
- Open the Threads window by choosing Debug > Windows > Threads.
- Locate and select the thread showing the current execution point (indicated by a yellow arrow).
- If the current execution thread shows no references to ArcGIS Maps SDK then locate the faulting thread.
- Inspect for references to ArcGIS Maps SDK for .NET.
- Select the thread of interest in the threads window.
- From the menu bar, navigate to Debug > Windows > Call Stack to view the call stack for the thread.
- In the Call Stack window, right click and choose Select All then copy the selected text.
- If you see one or more calls to
Esri.ArcGISRuntime.dll
with lines similar toruntimecore.dll!00007ffdeb571164
this indicates the call stack continues into the ArcGIS Runtime native libraries and you must enable native code debugging to get more information.
For more information see How to use the threads window in the Visual Studio documentation.
Enable native code debugging
- In Visual Studio, open the Project Properties dialog and select the Debug tab.
- Under the section Debugger engines, check the box to Enable native code debugging.
For more information see Enable mixed mode debugging in the Microsoft documentation.
Debug the application with native code debugging
-
In Visual Studio, click Start Debugging (or tap F5).
-
Click Debug > Windows > Modules to open the Modules window.
-
In the Modules window, right-click the entry for each of the following modules and choose Load Symbols:
Runtime
Core Net200_0.WPF.dll Runtime
Core Net200_0.dll runtimecore.dll
-
Ensure the Symbol Status for each module above is Symbols loaded.
-
Run through the workflow in your application that reproduces the problem.
-
From the menu bar, navigate to Debug > Windows > Threads to open the Threads window.
-
Locate and select the thread showing the current execution point (yellow arrow).
-
If the current execution thread shows no references to ArcGIS Runtime, locate the faulting thread.
-
Inspect for references to ArcGIS Runtime.
-
Select the thread of interest in the Threads window.
-
From the menu bar navigate to Debug > Windows > Call Stack to view the call stack for the thread.
-
In the Call Stack window right click, choose Select All and copy the selected text.
For more information see How to use the threads window in the Visual Studio documentation.
Workflow 2: Debug a dump file from a WPF application
Create dump file from your application process
- Run through the workflow in your application that reproduces the problem.
- When the application crashes open Windows Task Manager > select the Details tab > locate the process > Right click > choose Create dump file.
- On the Dumping Process dialog that appears, take note of the file location (select the path text and copy).
Debug the Dump File
-
In Visual Studio, navigate to File > Open > File.
-
In the open file dialog, browse for and choose the
.DMP
file (or paste the path you copied earlier). -
On the Minidump File Summary page look for the collapsible Actions section.
-
Choose Debug with Mixed.
-
For each of the following modules, locate the module > right click > choose Load Symbols:
Esri.ArcGISRuntime.dll
Esri.ArcGISRuntime.WPF.dll
Runtime
Core Net200_0.WPF.dll Runtime
Core Net200_0.dll runtimecore.dll
-
Ensure the Symbol Status for each module above is Symbols loaded.
-
From the menu bar, navigate to Debug > Windows > Threads to open the Threads window.
-
Locate and select the thread showing the current execution point (yellow arrow).
-
If the current execution thread shows no references to ArcGIS Runtime locate the faulting thread.
-
Inspect for references to ArcGIS Runtime.
-
Select the thread of interest in the threads window.
-
From the menu bar navigate to Debug > Windows > Call Stack to view the call stack for the thread.
-
In the Call Stack window right click, choose Select All and copy the selected text.
For more information see How to use the threads window in the Visual Studio documentation.
If you have copied the text for an ESRI-related call stack you can add this information to an Esri Community post or provide it to an Esri analyst when you contact Support to report your issue.
Example
The following example shows the type of call stack you might have in the event of a crash and shows how you might read a call stack to decipher potential causes of the problem and perhaps find a temporary workaround.
In July 2019 a developer posted on Esri Community to report a crash with 100.5 when their code called the asynchronous method Route
. They did the best thing you can do in this situation: they attached a simple standalone reproducer application to the post. It enabled the development team to repeat the exact workflow and get the call stack using the 100.5 symbol files. The call stack showed that code was failing within geometry spatial reference handling, which allowed us to recommend a straightforward workaround: specify the Spatial
when creating Map
geometries to represent Stops
. Since version 100.6, you too have access to the symbol files giving you a head start on identifying possible causes and temporary workarounds, and more specific information to share with us when reporting your issue. In this case, thanks to the excellent reproducer application from the user we were able to quickly diagnose and fix this bug for the 100.6 release.
Example call stack:
> runtimecore.dll!Esri_runtimecore::Geometry::Spatial_reference_impl::horizontal_equal_(class Esri_runtimecore::Geometry::Spatial_reference_impl const &) Unknown
runtimecore.dll!Esri_runtimecore::Geometry::Spatial_reference_impl::equals(class Esri_runtimecore::Geometry::Spatial_reference const &) Unknown
runtimecore.dll!Esri_runtimecore::Network_analyst::reproject(class std::shared_ptr const &,class std::shared_ptr const &,class std::shared_ptr const &) Unknown
runtimecore.dll!Esri_runtimecore::Network_analyst::NA_utils::reproject(class std::shared_ptr const &,class std::vector > &) Unknown
runtimecore.dll!Esri_runtimecore::Network_analyst::Local_route_task::initialize_stops_(class Esri_runtimecore::Network_analyst::Route_parameters const &,class std::vector > const &,class std::vector > &,class std::shared_ptr const &,class std::vector > &,class std::vector > &) Unknown
runtimecore.dll!Esri_runtimecore::Network_analyst::Local_route_task::solve(class Esri_runtimecore::Network_analyst::Route_parameters,class pplx::cancellation_token const &) Unknown
runtimecore.dll!Esri_runtimecore::Mapping::Local_solve_operation::solve_async(class std::shared_ptr const &,class std::shared_ptr const &,class Esri_runtimecore::Mapping::Task_completion_source >,class Esri_runtimecore::Mapping::Task_options) Unknown
runtimecore.dll!::operator()>() Unknown
runtimecore.dll!std::_Func_impl_no_alloc<,boost::any,pplx::task>::_Do_call() Unknown
runtimecore.dll!std::_Func_class > > >::operator()(class pplx::task > >) Unknown
runtimecore.dll!pplx::details::_PPLTaskHandle::_ContinuationTaskHandle,struct std::integral_constant,struct pplx::details::_TypeSelectorNoAsync>,struct pplx::details::_ContinuationTaskHandleBase>::invoke(void) Unknown
runtimecore.dll!pplx::details::_TaskProcHandle::_RunChoreBridge(void *) Unknown
runtimecore.dll!Esri_runtimecore::Common::Core_scheduler::bridge_proc_(void *) Unknown
runtimecore.dll!Esri_runtimecore::Common::Windows_Threadpool_scheduler::Scheduler_param::work_callback(struct _TP_CALLBACK_INSTANCE *,void *,struct _TP_WORK *) Unknown
ntdll.dll!77597dc4() Unknown
ntdll.dll![Frames below may be incorrect and/or missing, no symbols loaded for ntdll.dll] Unknown
[External Code]