Maps and scenes use a collection of layers to display geographic data from a variety of sources and formats. These can include several basemap layers and data layers from online or local sources.
Each layer in a map references geographic data, either from an online service or from a local dataset. There are a variety of layers that can be added to a map, each designed to display a particular type of data. Some layers display images, such as satellite photos or aerial photography, others are composed of a collection of features to represent real-world entities using point, line, or polygon geometries. In addition to geometry, features have attributes that provide details about the entity it represents.
Basemap layers
A basemap layer is a layer that provides the overall visual context for a map or scene. It typically contains geographic features such as continents, lakes, administrative boundaries, streets, cities, and place names. These features are represented with different styles provided by the basemap styles service such as streets, topographic, and imagery. The style you choose depends on the type of application and the visualization you would like to create.
A basemap layer provides the visual foundation for a mapping application. It typically contains data with global coverage and is the first layer added to a map or scene. The main data source for a basemap layer is the basemap styles service. When a view displays a map, a basemap layer is the first layer to draw, followed by data layers, and then graphics.
The basemap styles service provides a number of vector tile and image tile basemap layers that you can use to build different types of mapping applications. Each of the basemap styles is defined in an enumeration. For example, you can use the Navigation style to create an application that supports driving directions, or you can use Imagery to create an application that displays a real-world view of the earth.
// Provide an access token for your app (usually when the app starts).
//Esri.ArcGISRuntime.ArcGISRuntimeEnvironment.ApiKey = "YOUR_ACCESS_TOKEN";
var map = new Map(BasemapStyle.ArcGISNavigation);
// Use any of the available BasemapStyle values ...
// var map = new Map(BasemapStyle.ArcGISTopographic);
// var map = new Map(BasemapStyle.ArcGISLightGray);
// var map = new Map(BasemapStyle.OSMLightGray);
// ... several others.
// If preferred, you can provide a key directly for the basemap.
//map.Basemap.ApiKey = "YOUR_ACCESS_TOKEN";
// Provide an access token for your app (usually when the app starts).
//Esri.ArcGISRuntime.ArcGISRuntimeEnvironment.ApiKey = "YOUR_ACCESS_TOKEN";
var map = new Map(BasemapStyle.ArcGISImagery);
// Use any of the available BasemapStyle values ...
// var map = new Map(BasemapStyle.ArcGISHillshadeLight);
// var map = new Map(BasemapStyle.ArcGISHillshadeDark);
// ... several others.
// If preferred, you can provide a key directly for the basemap.
//map.Basemap.ApiKey = "YOUR_ACCESS_TOKEN";
Data layers
A data layer, also known as an operational layer, is a layer that can access geographic data from a data source. You use a data layer to display geographic data on top of a basemap layer in a map or scene. The data source for the layer can be a data service or a file such as a shapefile or GeoPackage.
A data layer provides access to geographic data that is displayed in a map or scene. Each layer references a file or service data source. The data source contains either as vector data (points, lines, polygons and attributes) or raster data (images). Different types of layers can access and display different types of data.
The data for a data layer is typically stored in ArcGIS as a data service. You can use feature services, map tile services, and vector tile services to host your data. Learn more in Data hosting.
A data layer can also be created from a dataset stored on the device. For example, data stored in a shapefile, mobile map package, or geodatabase. Learn more about using offline data in Offline maps, scenes, and data.
To add a data layer to a map or scene, you typically add imagery or tile layers first, and then polygons, lines, and points layers last. A map or scene controls the order of the layers, and the map or scene view combines the layers to create the final display.
// Provide an access token for your app (usually when the app starts).
//Esri.ArcGISRuntime.ArcGISRuntimeEnvironment.ApiKey = "YOUR_ACCESS_TOKEN";
var map = new Map(BasemapStyle.ArcGISTopographic);
// Create tables for feature data hosted in ArcGIS Online.
var trailheadsTable = new ServiceFeatureTable(new Uri("https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Trailheads_Styled/FeatureServer/0"));
var trailsTable = new ServiceFeatureTable(new Uri("https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Trails_Styled/FeatureServer/0"));
var openSpacesTable = new ServiceFeatureTable(new Uri("https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Parks_and_Open_Space_Styled/FeatureServer/0"));
// Create feature layers to show the tables, add them to the map's collection of data layers.
map.OperationalLayers.Add(new FeatureLayer(trailheadsTable));
map.OperationalLayers.Add(new FeatureLayer(trailsTable));
map.OperationalLayers.Add(new FeatureLayer(openSpacesTable));
MainView.Map = map;
Use API keys
An API Key access token can be used to authorize access to ArcGIS Online services and resources from your app, as well as to monitor access to those services. You can use a single access token for all requests made by your app, or assign individual access tokens for any classes that implements APIKeyResourceInterface
Tutorials
Samples
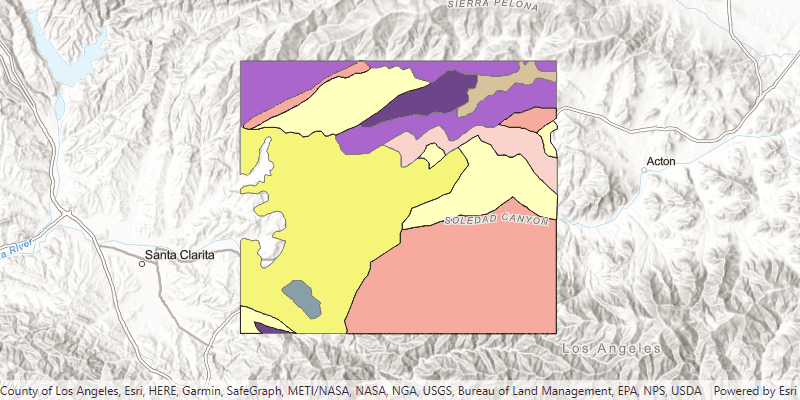
Feature layer (feature service)
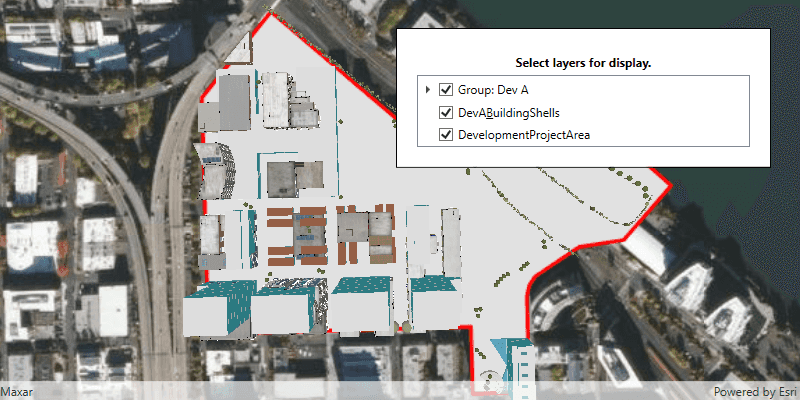
Group layers
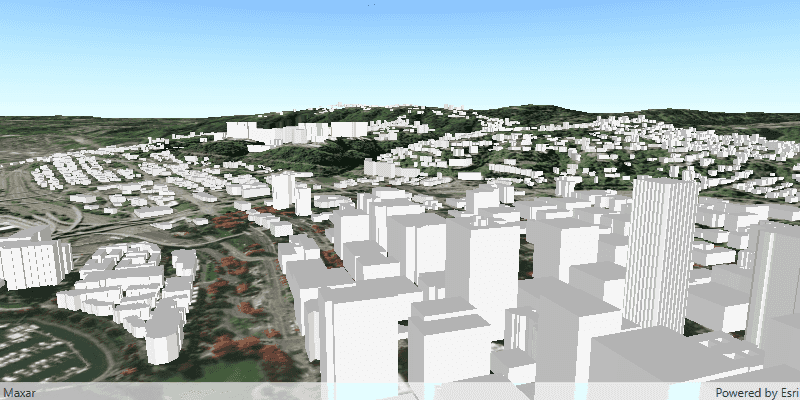
Scene layer
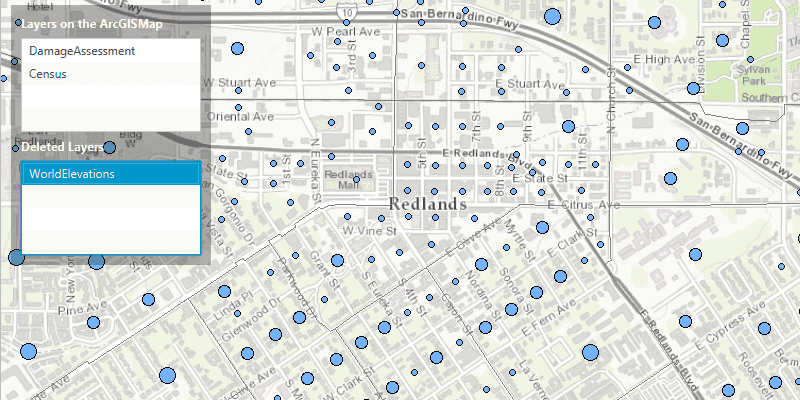
Manage operational layers
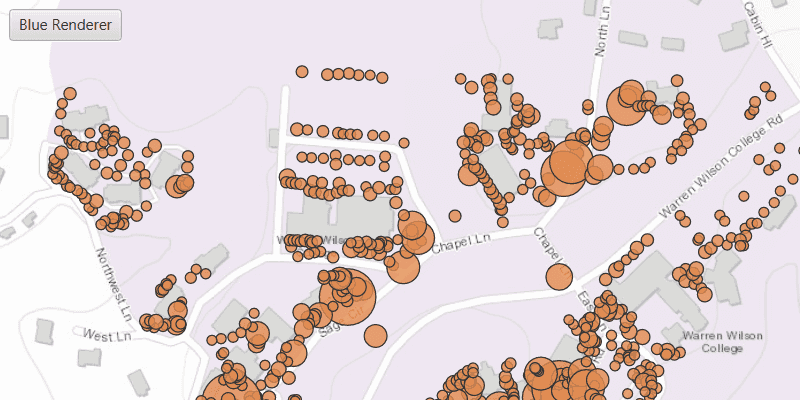
Change feature layer renderer
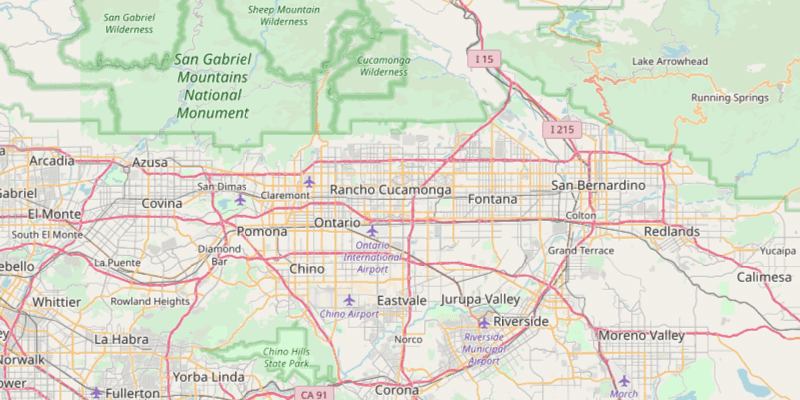