You may need to include additional (non-code) files for your app. Such resources commonly include things like images, fonts, or other visual elements for styling the app. For an ArcGIS Maps SDK for .NET app, you might also need to provide things like mobile map/scene packages (.mmpk, .mspk), tile packages (.tpk, .vtpk), geodatabases (.geodatabase), locators (.loc/.loz), and so on.
Include resources for your .NET MAUI app
In your .NET MAUI project, you can include resources (files) in a centralized location for consumption on each platform your app supports. Files you want to include with an app are typically placed in the Resources folder of your .NET MAUI app project. The Resources folder contains subfolders for various types of files, like images, fonts, and styles. You should add raw assets like the data files mentioned above to the raw subfolder with a build action of MauiAsset.
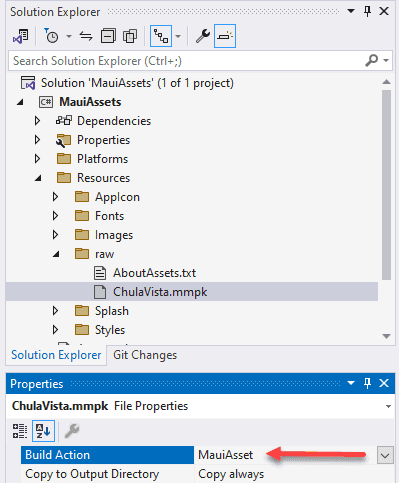
These files are deployed with your package and are accessed using code like this:
var stream = await FileSystem.OpenAppPackageFileAsync("ChulaVista.mmpk");
Accessing raw resources in the app package
How files are packaged varies a lot from platform to platform. For instance, files on Android are available as a stream that you can read rather than as a file on disk. For iOS, they are read-only files. A good technique when working with these files is to read a file from the package and write it to a local file in the app's data folder. Working with the file in the data folder avoids having to handle cross-platform package differences.
The following function reads a resource from the package and writes it to a file in the app's data folder (a folder created for your app when it is installed). The location of the app's data directory varies across platforms, but can consistently be referenced by the App
File system helper. The function checks if the file exists, and if it doesn't, the resource is written to a new file in the app's data directory.
private async Task<string> GetAppDataDirectoryFilePath(string resourceFilePath, string fileName)
{
// Check to see if the mobile map package is already present in the AppDataDirectory.
if (File.Exists(Path.Combine(FileSystem.Current.AppDataDirectory, fileName)))
{
// If the mobile map package is present in the AppDataDirectory, return the file path.
return Path.Combine(FileSystem.Current.AppDataDirectory, fileName);
}
// Open the source file.
using Stream inputStream = await FileSystem.OpenAppPackageFileAsync(resourceFilePath);
// Create an output filename
string targetFile = Path.Combine(FileSystem.Current.AppDataDirectory, fileName);
// Copy the file to the AppDataDirectory
using FileStream outputStream = File.Create(targetFile);
await inputStream.CopyToAsync(outputStream);
return targetFile;
}
The following code shows how to use this function to copy a mobile map package from the package to the app's data folder. The code then creates a MobileMapPackage
from the file in the app's data folder. In this example, the "ChulaVista.mmpk" file was added to the project's Resources/raw folder with a build action of MauiAsset.
// Get the path to the mobile map package.
string mmpkPath = await GetAppDataDirectoryFilePath("ChulaVista.mmpk", "ChulaVista.mmpk");
// Create the mobile map package.
MobileMapPackage mobileMapPackage = await MobileMapPackage.OpenAsync(mmpkPath);
Storing resources in a different folder
You may want to use a different folder in your project to store the raw resources used by your app. For example, you may want to create a new folder called "Assets" (at the same level as the "Resources" folder) and use it to store your app's resource files.
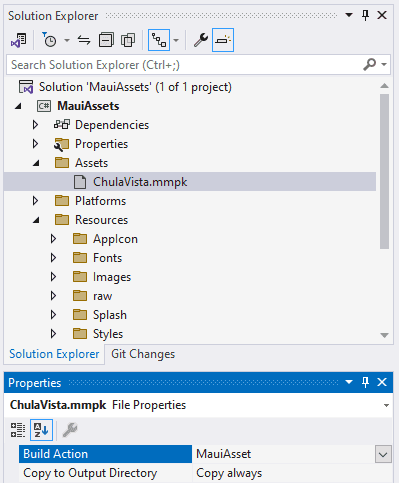
Your code will work nearly the same as described above as long as you set the Build Action to MauiAsset. The one difference will be how you reference the resource when using File
. In this case, you must prefix the resource name with the name of the folder. For example:
var inputStream = await FileSystem.OpenAppPackageFileAsync("Assets/ChulaVista.mmpk");