Learn how to use a URL to access and display a feature layer in a map.
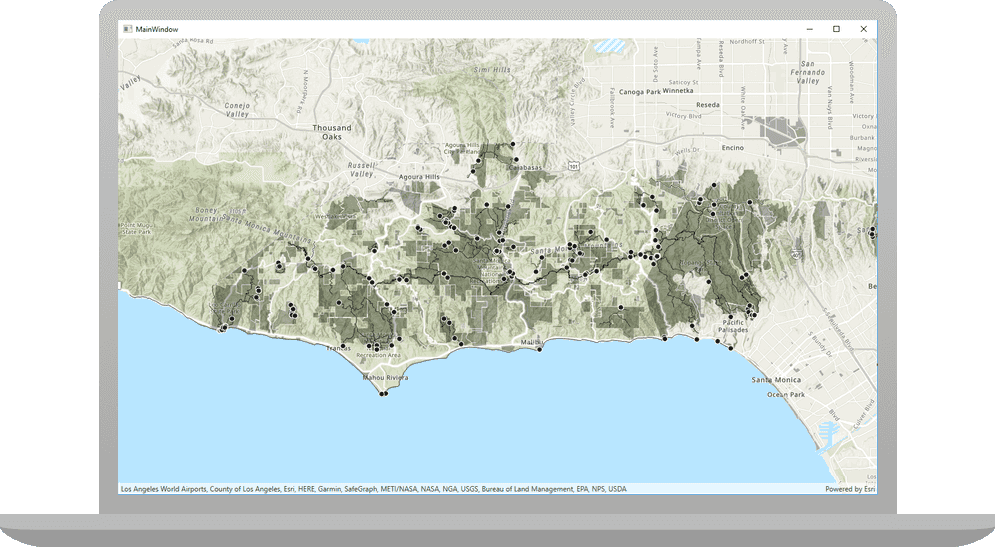
A map contains layers of geographic data. A map contains a basemap layer and, optionally, one or more data layers. This tutorial shows you how to access and display a feature layer in a map. You access feature layers with an item ID or URL. You will use URLs to access the Trailheads, Trails, and Parks and Open Spaces feature layers and display them in a map.
A feature layer is a dataset in a feature service hosted in ArcGIS. Each feature layer contains features with a single geometry type (point, line, or polygon), and a set of attributes. You can use feature layers to store, access, and manage large amounts of geographic data for your applications.
In this tutorial, you use URLs to access and display three different feature layers hosted in ArcGIS Online:
Prerequisites
Before starting this tutorial:
-
You need an ArcGIS Location Platform or ArcGIS Online account.
-
Ensure your development environment meets the system requirements.
Optionally, you may want to install the ArcGIS Maps SDK for .NET to get access to project templates in Visual Studio (Windows only) and offline copies of the NuGet packages.
Steps
Open a Visual Studio solution
-
To start the tutorial, complete the Display a map tutorial or download and unzip the solution.
-
Open the
.sln
file in Visual Studio. -
If you downloaded the solution, get an access token and set the API key.
An API Key gives your app access to secure resources used in this tutorial.
-
Go to the Create an API key tutorial to obtain a new API key access token. Ensure that the following privilege is enabled: Location services > Basemaps > Basemap styles service. Copy the access token as it will be used in the next step.
-
In Visual Studio, in the Solution Explorer, click App.xaml.cs.
-
In the App class, add an override for the
O
function to set then Startup() Api
property onKey ArcGISRuntimeEnvironment
.App.xaml.csUse dark colors for code blocks public partial class App : Application { protected override void OnStartup(StartupEventArgs e) { base.OnStartup(e); Esri.ArcGISRuntime.ArcGISRuntimeEnvironment.ApiKey = "YOUR_ACCESS_TOKEN"; } } }
-
If you are developing with Visual Studio for Windows, ArcGIS Maps SDK for .NET provides a set of project templates for each supported .NET platform. These templates follow the Model-View-ViewModel (MVVM) design pattern. Install the ArcGIS Maps SDK for .NET Visual Studio Extension to add the templates to Visual Studio (Windows only). See Install and set up for details.
Update the tutorial name used in the project (optional)
The Visual Studio solution, project, and the namespace for all classes currently use the name Display
. Follow the steps below if you prefer the name to reflect the current tutorial. This is not required, your code will still work if you keep the original name. The instructions will refer to Add
rather than Display
.
-
Update the name for the solution and the project.
- In the Visual Studio > Solution Explorer, right-click the solution name and choose Rename. Name the solution
Add
.AFeature Layer - In the Solution Explorer, right-click the project name and choose Rename. Name the project
Add
.AFeature Layer
- In the Visual Studio > Solution Explorer, right-click the solution name and choose Rename. Name the solution
-
Update the name of the namespace used by classes in the project.
- In the Solution Explorer, expand the project node.
- Double-click
Map
in the Solution Explorer to open the file.View Model.cs - In the
Map
class, double-click the namespace name (View Model Display
) to highlight it, right-click and choose Rename....AMap - Rename the namespace
Add
.AFeature Layer - Click Apply in the Rename: DisplayAMap window that appears in the upper-right of the code window. This will rename the namespace throughout your project.
-
Build the project.
- Choose Build > Build solution (or press <F6>).
Create URIs to reference feature service data
To display three new data layers (also known as operational layers) on top of the current basemap, you will create FeatureLayer
s using URIs to reference datasets hosted in ArcGIS Online.
-
Open a browser and navigate to the URL for Parks and Open Spaces to view metadata about the layer. To display the layer in your app, you only need the URL.
The service page provides information such as the geometry type, the geographic extent, the minimum and maximum scale at which features are visible, and the attributes (fields) it contains. You can preview the layer by clicking on ArcGIS.com Map in the "View In:" list at the top of the page.
-
In the Visual Studio > Solution Explorer, double-click MapViewModel.cs to open the file.
The project uses the Model-View-ViewModel (MVVM) design pattern to separate the application logic (view model) from the user interface (view).
Map
contains the view model class for the application, calledView Model.cs Map
. See the Microsoft documentation for more information about the Model-View-ViewModel pattern.View Model -
In the
Setup
function, add code that defines the URIs to the hosted layers. You will add: Trailheads (points), Trails (lines), and Parks and Open Spaces (polygons).Map() Use dark colors for code blocks private void SetupMap() { Map = new Map(BasemapStyle.ArcGISTopographic); var parksUri = new Uri( "https://services3.arcgis.com/GVgbJbqm8hXASVYi/ArcGIS/rest/services/Parks_and_Open_Space/FeatureServer/0" ); var trailsUri = new Uri( "https://services3.arcgis.com/GVgbJbqm8hXASVYi/ArcGIS/rest/services/Trails/FeatureServer/0" ); var trailheadsUri = new Uri( "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Trailheads/FeatureServer/0" );
Create feature layers to display the hosted data
You will create three new FeatureLayer
to display the hosted layer at each URI.
-
In the
Setup
function, create new feature layers and pass the appropriate URI to the constructor for each.Map() Use dark colors for code blocks var trailheadsUri = new Uri( "https://services3.arcgis.com/GVgbJbqm8hXASVYi/arcgis/rest/services/Trailheads/FeatureServer/0" ); var parksLayer = new FeatureLayer(parksUri); var trailsLayer = new FeatureLayer(trailsUri); var trailheadsLayer = new FeatureLayer(trailheadsUri);
-
Add the feature layers to the map. Data layers are displayed in the order in which they are added. Polygon layers should be added before layers with lines or points if there's a chance the polygon symbols will obscure features beneath.
Use dark colors for code blocks var parksLayer = new FeatureLayer(parksUri); var trailsLayer = new FeatureLayer(trailsUri); var trailheadsLayer = new FeatureLayer(trailheadsUri); Map.OperationalLayers.Add(parksLayer); Map.OperationalLayers.Add(trailsLayer); Map.OperationalLayers.Add(trailheadsLayer);
-
Click Debug > Start Debugging (or press <F5> on the keyboard) to run the app.
You should see point, line, and polygon features (representing trailheads, trails, and parks) draw on the map for an area in the Santa Monica Mountains.
What's next?
Learn how to use additional API features, ArcGIS location services, and ArcGIS tools in these tutorials: