Learn how to implement user authentication to access a secure ArcGIS service with OAuth credentials.
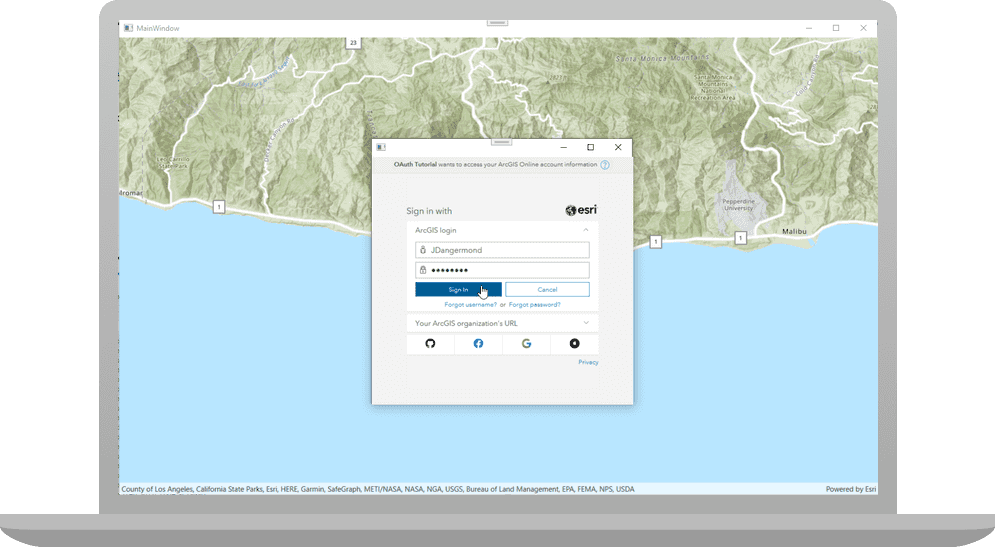
You can use different types of authentication to access secured ArcGIS services. To implement OAuth credentials for user authentication, you can use your ArcGIS account to register an app with your portal and get a Client ID, and then configure your app to redirect users to login with their credentials when the service or content is accessed. This is known as user authentication. If the app uses premium ArcGIS Online services that consume credits, for example, the app user's account will be charged.
In this tutorial, you will build an app that implements user authentication using OAuth credentials so users can sign in and be authenticated through ArcGIS Online to access the ArcGIS World Traffic service.
Prerequisites
Before starting this tutorial:
-
You need an ArcGIS Location Platform or ArcGIS Online account.
-
Ensure your development environment meets the system requirements.
Optionally, you may want to install the ArcGIS Maps SDK for .NET to get access to project templates in Visual Studio (Windows only) and offline copies of the NuGet packages.
Steps
Create OAuth credentials for user authentication
OAuth credentials are required to implement user authentication. These credentials are created as an Application item in your organization's portal.
-
Go to the Create OAuth credentials for user authentication tutorial and create OAuth credentials using your ArcGIS Location Platform or ArcGIS Online account.
-
Copy the
Client ID
andRedirect URL
as you will use them to implement user authentication in the next step. TheClient ID
is found on the Application item's Overview page, while theRedirect URL
is found on the Settings page.
The Client ID
uniquely identifies your app on the authenticating server. If the server cannot find an app with the provided Client ID, it will not proceed with authentication.
The Redirect URL
is used to identify a response from the authenticating server when the system returns control back to your app after an OAuth login. You can configure several redirect URLs in your application definition and can remove or edit them. It's important to make sure the redirect URL used in your app's code matches a redirect URL configured for the application.
Open a Visual Studio solution
-
To start the tutorial, complete the Display a map tutorial or download and unzip the solution.
-
Open the .sln file in Visual Studio.
-
Open the App.xaml.cs file and delete the code that sets your access token. Since your app uses OAuth, you will not need an access token.
App.xaml.csUse dark colors for code blocks 28 29 30 36 37 38Remove line Remove line Remove line Remove line Remove line public partial class App : Application { protected override void OnStartup(StartupEventArgs e) { base.OnStartup(e); Esri.ArcGISRuntime.ArcGISRuntimeEnvironment.ApiKey = "YOUR_ACCESS_TOKEN"; } } }
If you are developing with Visual Studio for Windows, ArcGIS Maps SDK for .NET provides a set of project templates for each supported .NET platform. These templates follow the Model-View-ViewModel (MVVM) design pattern. Install the ArcGIS Maps SDK for .NET Visual Studio Extension to add the templates to Visual Studio (Windows only). See Install and set up for details.
Update the tutorial name used in the project (optional)
The Visual Studio solution, project, and the namespace for all classes currently use the name Display
. Follow the steps below if you prefer the name to reflect the current tutorial. These steps are not required, your code will still work if you keep the original name.
The tutorial instructions and code use the name Access
for the solution, project, and namespace. You can choose any name you like, but it should be the same for each of these.
-
Update the name for the solution and the project.
- In Visual Studio, in the Solution Explorer, right-click the solution name and choose Rename. Provide the new name for your solution.
- In the Solution Explorer, right-click the project name and choose Rename. Provide the new name for your project.
-
Rename the namespace used by classes in the project.
- In the Solution Explorer, expand the project node.
- Double-click MapViewModel.cs in the Solution Explorer to open the file.
- In the
Map
class, double-click the namespace name (View Model Display
) to select it, and then right-click and choose Rename....A Map - Provide the new name for the namespace.
- Click Apply in the Rename: DisplayAMap window that appears in the upper-right of the code window. This will rename the namespace throughout your project.
-
Build the project.
- Choose Build > Build solution (or press <F6>).
Add a traffic layer
You will add a layer to display the ArcGIS World Traffic service, a dynamic map service that presents historical and near real-time traffic information for different regions in the world. This is a secure service and requires an ArcGIS Online organizational subscription.
ArcGIS World Traffic service data is updated every five minutes to provide traffic speed and traffic incident visualization and identification.
Traffic speeds are displayed as a percentage of free-flow speeds, which is frequently the speed limit or how fast cars tend to travel when unencumbered by other vehicles. The streets are color coded as follows:
- Green (fast): 85 - 100% of free flow speeds
- Yellow (moderate): 65 - 85%
- Orange (slow); 45 - 65%
- Red (stop and go): 0 - 45%
-
In the Visual Studio > Solution Explorer, double-click MapViewModel.cs to open the file.
The project uses the Model-View-ViewModel (MVVM) design pattern to separate the application logic (view model) from the user interface (view).
Map
contains the view model class for the application, calledView Model.cs Map
. See the Microsoft documentation for more information about the Model-View-ViewModel pattern.View Model -
In the
Setup
function, update the line that sets theMap() Map
property for the view model to instead store the map in a variable.MapViewModel.csUse dark colors for code blocks private void SetupMap() { // Create a new map with a 'topographic vector' basemap. var trafficMap = new Map(BasemapStyle.ArcGISTopographic); }
-
Create an
ArcGISMapImageLayer
to display the traffic service and add it to the map's collection of data layers (operational layers).MapViewModel.csUse dark colors for code blocks private void SetupMap() { // Create a new map with a 'topographic vector' basemap. var trafficMap = new Map(BasemapStyle.ArcGISTopographic); // Create a layer to display the ArcGIS World Traffic service. var trafficServiceUrl = "https://traffic.arcgis.com/arcgis/rest/services/World/Traffic/MapServer"; var trafficLayer = new ArcGISMapImageLayer(new Uri(trafficServiceUrl)); // Add the traffic layer to the map's data layer collection. trafficMap.OperationalLayers.Add(trafficLayer); }
-
Set the view model's
Map
property. Data binding handles displaying the map in the view.MapViewModel.csUse dark colors for code blocks private void SetupMap() { // Create a new map with a 'topographic vector' basemap. var trafficMap = new Map(BasemapStyle.ArcGISTopographic); // Create a layer to display the ArcGIS World Traffic service. var trafficServiceUrl = "https://traffic.arcgis.com/arcgis/rest/services/World/Traffic/MapServer"; var trafficLayer = new ArcGISMapImageLayer(new Uri(trafficServiceUrl)); // Add the traffic layer to the map's data layer collection. trafficMap.OperationalLayers.Add(trafficLayer); // Set the view model Map property with the new map. this.Map = trafficMap; }
-
Click Debug > Start Debugging (or press <F5> on the keyboard) to run the app.
If creating apps for Android or iOS, you will need the appropriate emulator, simulator, or device configured for testing (see System requirements for details)
You should see a map with the topographic basemap layer centered on the Santa Monica Mountains in California. The traffic layer doesn't appear because it requires authentication with ArcGIS Online.
Handle layer load errors
If a map attempts to load a layer based on a secured service without the required authentication, the layer is not added to the map. No exception is raised and unless you handle it in your code, the user may never know that the secured resource is missing from the map.
You will handle load status change events for the traffic layer and report an error message if the layer fails to load.
-
In the
Setup
function, after the line that creates the traffic layer, add the following line of code to handle the layer's load status changed event. You will create the code for this handler in another step.Map() MapViewModel.csUse dark colors for code blocks private void SetupMap() { // Create a new map with a 'topographic vector' basemap. var trafficMap = new Map(BasemapStyle.ArcGISTopographic); // Create a layer to display the ArcGIS World Traffic service. var trafficServiceUrl = "https://traffic.arcgis.com/arcgis/rest/services/World/Traffic/MapServer"; var trafficLayer = new ArcGISMapImageLayer(new Uri(trafficServiceUrl)); // Handle changes in the traffic layer's load status to check for errors. trafficLayer.LoadStatusChanged += TrafficLayer_LoadStatusChanged; // Add the traffic layer to the map's data layer collection. trafficMap.OperationalLayers.Add(trafficLayer); // Set the view model Map property with the new map. this.Map = trafficMap; }
-
Below the
Setup
function, create the function that handles load status change events for the layer. If the layer fails to load, display an error message to the user.Map() MapViewModel.csUse dark colors for code blocks private void TrafficLayer_LoadStatusChanged(object? sender, Esri.ArcGISRuntime.LoadStatusEventArgs e) { // Report the error message if the traffic layer fails to load. if (e.Status == Esri.ArcGISRuntime.LoadStatus.FailedToLoad) { var trafficLayer = sender as ArcGISMapImageLayer; System.Windows.MessageBox.Show(trafficLayer?.LoadError?.Message, "Traffic layer did not load"); } }
-
Click Debug > Start Debugging (or press <F5> on the keyboard) to run the app.
If creating apps for Android or iOS, you will need the appropriate emulator, simulator, or device configured for testing (see System requirements for details)
You should see the same map display, but this time a message box reports a "Token required" error when the traffic layer fails to load. An access token is an authorization string that provides secure access to content, data, and functionality in ArcGIS location services. To load the traffic service layer, you will use OAuth to get an access token based on ArcGIS Online credentials.
Implement user authentication using OAuth credentials
This API abstracts some of the details for user authentication using OAuth credentials in your app. You can use classes such as AuthenticationManager
to request, store, and manage credentials for secure resources.
You will add an authentication helper class that uses AuthenticationManager
and encapsulates additional authentication logic.
-
From the Visual Studio Project menu, choose Add class .... Name the class
Authentication
then click Add. The new class is added to your project and opens in Visual Studio.Helper.cs -
Select all the code in the class and delete it.
-
Copy all of the code below and paste it into the
Authentication
class in your project.Helper.cs AuthenticationHelper.csUse dark colors for code blocks Copy // Copyright 2022 Esri // Licensed under the Apache License, Version 2.0 (the "License"); // you may not use this file except in compliance with the License. // You may obtain a copy of the License at // // https://www.apache.org/licenses/LICENSE-2.0 // // Unless required by applicable law or agreed to in writing, software // distributed under the License is distributed on an "AS IS" BASIS, // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. // See the License for the specific language governing permissions and // limitations under the License. using Esri.ArcGISRuntime.Security; using System; using System.Collections.Generic; using System.Threading.Tasks; using System.Windows; using System.Windows.Controls; using System.Windows.Navigation; using System.Windows.Threading; namespace AccessServicesWithOAuth { // A helper class that manages authenticating with a server to access secure resources. internal static class AuthenticationHelper { // Specify the Client ID and Redirect URL to use for OAuth authentication. // Sign in with your ArcGIS Location Platform or ArcGIS Online account // (https://www.arcgis.com/home/signin.html) to create your OAuth Application item. private const string OAuthClientID = "YOUR_CLIENT_ID"; private const string OAuthRedirectUrl = "YOUR_REDIRECT_URL"; static AuthenticationHelper() { // Use the OAuthAuthorize class (defined below) to show the login UI. AuthenticationManager.Current.OAuthAuthorizeHandler = new OAuthAuthorize(); // Create a new ChallengeHandler that uses a method in this class to challenge for credentials. AuthenticationManager.Current.ChallengeHandler = new ChallengeHandler(PromptCredentialAsync); } // A function to register a secure server with the AuthenticationManager. // Pass in the URL of the server containing secure resources and, optionally, a client ID and // redirect URL (if not specified, the values defined above are used). public static void RegisterSecureServer(string url, string clientID = OAuthClientID, string redirectUrl = OAuthRedirectUrl) { // Define the server URL, authentication type, client ID, and redirect URL. ServerInfo portalServerInfo = new ServerInfo(new Uri(url)) { TokenAuthenticationType = TokenAuthenticationType.OAuthAuthorizationCode, OAuthClientInfo = new OAuthClientInfo(clientID, new Uri(redirectUrl)) }; // Register the server information with the AuthenticationManager. AuthenticationManager.Current.RegisterServer(portalServerInfo); } // A function that adds a credential to AuthenticationManager based on a temporary token. // This is useful for testing an app with secured services without having to log in. public static void ApplyTemporaryToken(string url, string token) { // Create a new OAuth credential for the specified URL with the token. OAuthTokenCredential tempToken = new OAuthTokenCredential(new Uri(url), token); // Add the credential to the AuthenticationManager. AuthenticationManager.Current.AddCredential(tempToken); } // The ChallengeHandler function that is called when access to a secured resource is attempted. public static async Task<Credential> PromptCredentialAsync(CredentialRequestInfo info) { Credential credential = null; try { // Get credentials for the specified server. The OAuthAuthorize class (defined below) // will get the user's credentials (show the login window and handle the response). credential = await AuthenticationManager.Current.GenerateCredentialAsync(info.ServiceUri); } catch (OperationCanceledException) { // Login was cancelled, no need to display an error to the user. } return credential; } #region OAuth handler // In a desktop (WPF) app, an IOAuthAuthorizeHandler component is used to handle some of // the OAuth details. Specifically, it implements AuthorizeAsync to show the login UI // (generated by the server that hosts secure content) in a web control. When the user // logs in successfully, cancels the login, or closes the window without continuing, the // IOAuthAuthorizeHandler is responsible for obtaining the authorization from the server // or raising an OperationCanceledException. public class OAuthAuthorize : IOAuthAuthorizeHandler { // Window to contain the login UI provided by the server. private Window _authWindow; // Use a TaskCompletionSource to track the completion of the authorization. private TaskCompletionSource<IDictionary<string, string>> _tcs; // URL for the authorization callback result (the redirect URI configured for your application). private string _callbackUrl; // URL that handles the authorization request for the server (provides the login UI). private string _authorizeUrl; // Function to initiate an authorization request. It takes the URIs for: the secured service, // the authorization endpoint, and the redirect URI. public Task<IDictionary<string, string>> AuthorizeAsync(Uri serviceUri, Uri authorizeUri, Uri callbackUri) { // Don't start an authorization request if one is still in progress. if (_tcs != null && !_tcs.Task.IsCompleted) { throw new Exception("Task in progress"); } // Create a new TaskCompletionSource to track progress. _tcs = new TaskCompletionSource<IDictionary<string, string>>(); // Store the authorization and redirect URLs. _authorizeUrl = authorizeUri.AbsoluteUri; _callbackUrl = callbackUri.AbsoluteUri; // Call a function to show the login page, make sure it runs on the UI thread for this app. Dispatcher dispatcher = Application.Current.Dispatcher; if (dispatcher == null || dispatcher.CheckAccess()) { // Currently on the UI thread, no need to dispatch. ShowLoginWindow(_authorizeUrl); } else { Action authorizeOnUIAction = () => ShowLoginWindow(_authorizeUrl); dispatcher.BeginInvoke(authorizeOnUIAction); } // Return the task associated with the TaskCompletionSource. return _tcs.Task; } // A function to show a login page hosted at the specified Url. private void ShowLoginWindow(string authorizeUri) { // Create a WebBrowser control to display the authorize page. WebBrowser webBrowser = new WebBrowser(); // Handle the navigation event for the browser to check for a response sent to the redirect URL. webBrowser.Navigating += WebBrowserOnNavigating; // Display the web browser in a new window. _authWindow = new Window { Content = webBrowser, Width = 450, Height = 450, WindowStartupLocation = WindowStartupLocation.CenterOwner }; // Set the app's window as the owner of the browser window // (if main window closes, so will the browser). if (Application.Current != null && Application.Current.MainWindow != null) { _authWindow.Owner = Application.Current.MainWindow; } // Handle the window closed event. _authWindow.Closed += OnWindowClosed; // Navigate the browser to the authorization url. webBrowser.Navigate(authorizeUri); // Display the window. _authWindow.ShowDialog(); } // Handle the browser window closing. private void OnWindowClosed(object sender, EventArgs e) { // If the browser window closes, return the focus to the main window. if (_authWindow != null && _authWindow.Owner != null) { _authWindow.Owner.Focus(); } // If the task wasn't completed, the user must have closed the window without logging in. if (!_tcs.Task.IsCompleted) { // Set the task completion source exception to indicate a canceled operation. _tcs.SetCanceled(); } _authWindow = null; } // Handle browser navigation (content changing). private void WebBrowserOnNavigating(object sender, NavigatingCancelEventArgs e) { // If no browser, uri, or an empty url, return. WebBrowser webBrowser = sender as WebBrowser; Uri uri = e.Uri; if (webBrowser == null || uri == null || string.IsNullOrEmpty(uri.AbsoluteUri)) return; // Check if browser was redirected to the callback URL (succesful authentication). if (uri.AbsoluteUri.StartsWith(_callbackUrl)) { e.Cancel = true; // Call a function to parse key/value pairs from the response URI. IDictionary<string, string> authResponse = DecodeParameters(uri); // Set the result for the task completion source with the dictionary. _tcs.SetResult(authResponse); // Close the window. if (_authWindow != null) { _authWindow.Close(); } } } // A function to parse key/value pairs from the provided URI. private static IDictionary<string, string> DecodeParameters(Uri uri) { // Get the values from the URI fragment or query string. string responseInfo = ""; if (!string.IsNullOrEmpty(uri.Fragment)) { responseInfo = uri.Fragment.Substring(1); } else { if (!string.IsNullOrEmpty(uri.Query)) { responseInfo = uri.Query.Substring(1); } } // Split the strings for each parameter (delimited with '&'). Dictionary<string, string> keyValueDictionary = new Dictionary<string, string>(); string[] keysAndValues = responseInfo.Split(new[] { '&' }, StringSplitOptions.RemoveEmptyEntries); // Iterate the parameter info and split into key/value pairs (delimited with '='). foreach (string kvString in keysAndValues) { string[] pair = kvString.Split('='); string key = pair[0]; string value = string.Empty; if (key.Length > 1) { value = Uri.UnescapeDataString(pair[1]); } // Add the key and corresponding value to the dictionary. keyValueDictionary.Add(key, value); } // Return the dictionary of string keys/values. return keyValueDictionary; } } #endregion OAuth handler } }
-
Add your values for
Client ID
(O
) andAuth Client ID Redirect URL
(O
). These were created with your OAuth Application item in an earlier step and are unique to your application.Auth Redirect Url AuthenticationHelper.csUse dark colors for code blocks // A helper class that manages authenticating with a server to access secure resources. internal static class AuthenticationHelper { // Specify the Client ID and Redirect URL to use for OAuth authentication. // Sign in with your ArcGIS Location Platform or ArcGIS Online account // (https://www.arcgis.com/home/signin.html) to create your OAuth Application item. private const string OAuthClientID = "YOUR_CLIENT_ID"; private const string OAuthRedirectUrl = "YOUR_REDIRECT_URL";
The Authentication
class is now set up to handle OAuth authentication in your app. This class is generic enough that you can use it in any app, as long as valid Client ID
and Redirect URL
values are provided.
The last step is to use Authentication
to register one or more server URLs with AuthenticationManager
to authenticate the user for secure resources from those servers.
Register ArcGIS Online for user authentication
Registering a portal with AuthenticationManager
ensures that the user will be authenticated for any secured services on that portal. The portal is registered using its URL and the type of authentication it requires (one of the token authentication types supported for ArcGIS). For OAuth authentication, you must also provide OAuth Application item details, such as the Client ID
and Redirect URL
.
-
In the Visual Studio > Solution Explorer, double-click MapViewModel.cs to open the file.
-
In the
Setup
function, add a line of code that usesMap() Authentication
to register ArcGIS Online for authentication.Helper MapViewModel.csUse dark colors for code blocks private void SetupMap() { // Add the ArcGIS Online URL to the authentication helper. AuthenticationHelper.RegisterSecureServer("https://www.arcgis.com/sharing/rest"); // Create a new map with a 'topographic vector' basemap. var trafficMap = new Map(BasemapStyle.ArcGISTopographic); // Create a layer to display the ArcGIS World Traffic service. var trafficServiceUrl = "https://traffic.arcgis.com/arcgis/rest/services/World/Traffic/MapServer"; var trafficLayer = new ArcGISMapImageLayer(new Uri(trafficServiceUrl)); // Handle changes in the traffic layer's load status to check for errors. trafficLayer.LoadStatusChanged += TrafficLayer_LoadStatusChanged; // Add the traffic layer to the map's data layer collection. trafficMap.OperationalLayers.Add(trafficLayer); // Set the view model Map property with the new map. this.Map = trafficMap; }
-
Click Debug > Start Debugging (or press <F5> on the keyboard) to run the app.
If creating apps for Android or iOS, you will need the appropriate emulator, simulator, or device configured for testing (see System requirements for details)
You should again see a map with the topographic basemap layer centered on the Santa Monica Mountains in California. You will be prompted for an ArcGIS Online username and password. Once you authenticate successfully with ArcGIS Online, the traffic layer will appear in the map.
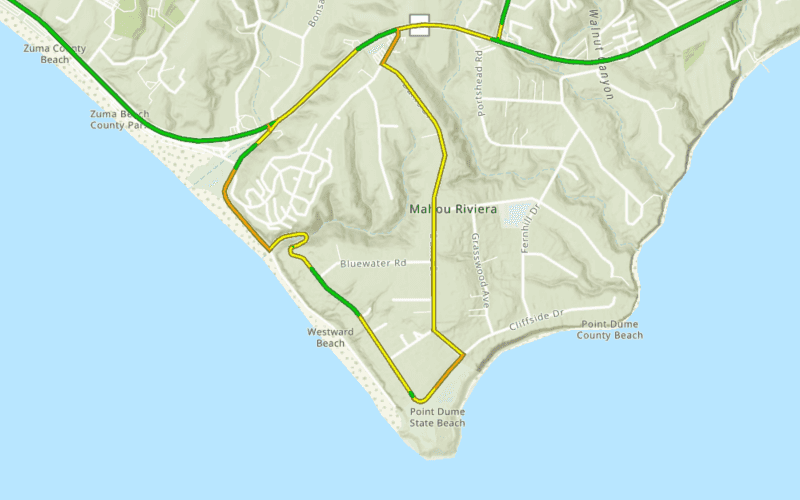
Testing apps that require authentication
When you are testing apps that require authentication for one or more services in the map, it can become monotonous to enter your OAuth credentials repeatedly. You can programmatically add authentication for a server using the temporary token
provided with your OAuth Application item details. As the name suggests, this token is valid for a limited time, but you can always get a fresh token from ArcGIS.com using your ArcGIS account.
The Authentication
has the following function for applying a temporary token.
// A function that adds a credential to AuthenticationManager based on a temporary token.
// This is useful for testing an app with secured services without having to log in.
public static void ApplyTemporaryToken(string url, string token)
{
// Create a new OAuth credential for the specified URL with the token.
OAuthTokenCredential tempToken = new OAuthTokenCredential(new Uri(url), token);
// Add the credential to the AuthenticationManager.
AuthenticationManager.Current.AddCredential(tempToken);
}
You can call the function with code such as:
AuthenticationHelper.ApplyTemporaryToken("https://www.arcgis.com/sharing/rest", "YOUR_TEMP_TOKEN");
Until the token expires, you can run the app without being challenged to provide your credentials.
Additional resources
If you are implementing OAuth for another .NET platform, you might find some of the following resources helpful.
- ArcGIS Maps SDK for .NET OAuth sample: The WPF version of this sample uses code similar to code used in this tutorial. The sample is available for other .NET platforms (use the switcher control at the top of the page) to illustrate implementing OAuth for apps targeting WinUI, UWP, and .NET MAUI.
- Web authentication broker: Use this authentication broker to implement OAuth in your Universal Windows Platform (UWP) apps.