Learn how to implement user authentication to access a secure ArcGIS service with OAuth credentials in a cross-platform app.
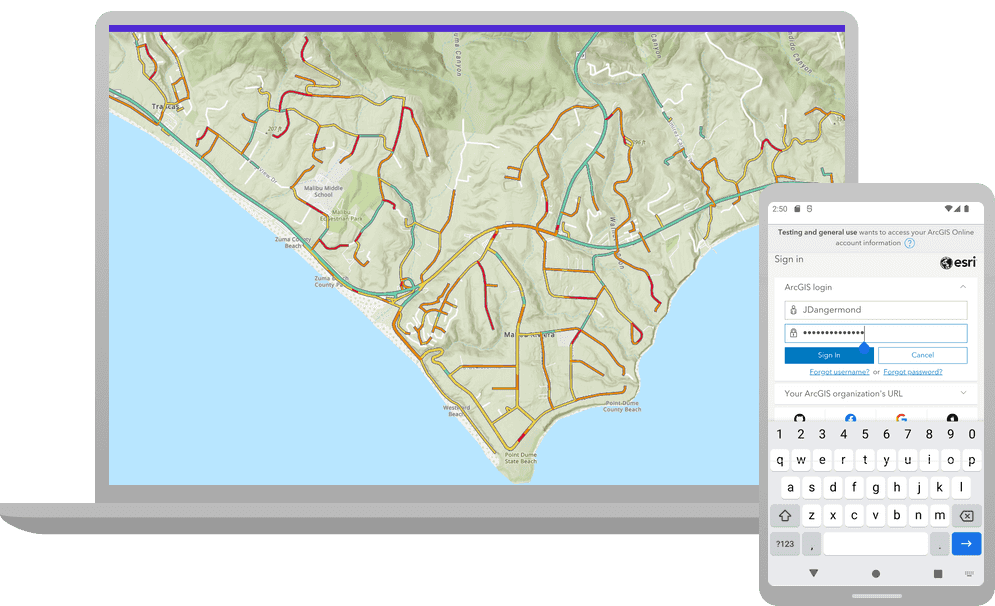
You can use different types of authentication to access secured ArcGIS services. To implement OAuth credentials for user authentication, you can use your ArcGIS account to register an app with your portal and get a Client ID, and then configure your app to redirect users to login with their credentials when the service or content is accessed. This is known as user authentication. If the app uses premium ArcGIS Online services that consume credits, for example, the app user's account will be charged.
In this tutorial, you will build an app that implements user authentication using OAuth credentials so users can sign in and be authenticated through ArcGIS Online to access the ArcGIS World Traffic service.
Prerequisites
Before starting this tutorial:
-
You need an ArcGIS Location Platform or ArcGIS Online account.
-
Ensure your development environment meets the system requirements.
Optionally, you may want to install the ArcGIS Maps SDK for .NET to get access to project templates in Visual Studio (Windows only) and offline copies of the NuGet packages.
Steps
Create OAuth credentials for user authentication
OAuth credentials are required to implement user authentication. These credentials are created as an Application item in your organization's portal.
-
Go to the Create OAuth credentials for user authentication tutorial and create OAuth credentials using your ArcGIS Location Platform or ArcGIS Online account.
-
Copy the
Client ID
andRedirect URL
as you will use them to implement user authentication in the next step. TheClient ID
is found on the Application item's Overview page, while theRedirect URL
is found on the Settings page.
The Client ID
uniquely identifies your app on the authenticating server. If the server cannot find an app with the provided Client ID, it will not proceed with authentication.
The Redirect URL
is used to identify a response from the authenticating server when the system returns control back to your app after an OAuth login. You can configure several redirect URLs in your application definition and can remove or edit them. It's important to make sure the redirect URL used in your app's code matches a redirect URL configured for the application.
Open a Visual Studio solution
-
To start the tutorial, complete the Display a map (.NET MAUI) tutorial or download and unzip the solution.
-
Open the .sln file in Visual Studio.
-
Open the MauiProgram.cs file and delete the code that sets your access token. Since your app uses OAuth, you will not need an access token.
MauiProgram.csUse dark colors for code blocks 21 22 23 24 25 26 27 28 29 30 31 32 33 35 36 37 38 39 40 41 42Remove line public static class MauiProgram { public static MauiApp CreateMauiApp() { var builder = MauiApp.CreateBuilder(); builder .UseMauiApp<App>() .ConfigureFonts(fonts => { fonts.AddFont("OpenSans-Regular.ttf", "OpenSansRegular"); fonts.AddFont("OpenSans-Semibold.ttf", "OpenSansSemibold"); }); builder.UseArcGISRuntime(config => config.UseApiKey("YOUR_ACCESS_TOKEN")); #if DEBUG builder.Logging.AddDebug(); #endif return builder.Build(); } }
If you are developing with Visual Studio for Windows, ArcGIS Maps SDK for .NET provides a set of project templates for each supported .NET platform. These templates provide all of the code needed for a basic Model-View-ViewModel (MVVM) app. Install the ArcGIS Maps SDK for .NET Visual Studio Extension to add the templates to Visual Studio (Windows only). See Install and set up for details.
Update the tutorial name used in the project (optional)
The Visual Studio solution, project, and the namespace for all classes currently use the name Display
. Follow the steps below if you prefer the name to reflect the current tutorial. These steps are not required, your code will still work if you keep the original name.
The tutorial instructions and code use the name Access
for the solution, project, and namespace. You can choose any name you like, but it should be the same for each of these.
-
Update the name for the solution and the project.
- In Visual Studio, in the Solution Explorer, right-click the solution name and choose Rename. Provide the new name for your solution.
- In the Solution Explorer, right-click the project name and choose Rename. Provide the new name for your project.
-
Rename the namespace used by classes in the project.
-
Open the Quick Find tool (Ctrl + f on the keyboard).
-
Toggle to replace mode by clicking the down arrow (⌄) to the left of the "Find..." entry.
-
Enter the current namespace in the top ("Find...") textbox.
-
Enter the new name for the namespace in the bottom ("Replace...") textbox.
-
Ensure Entire solution is selected in the dropdown below the text entries.
-
Click the Replace all button to the far right of the "Replace..." text box (or press Alt + a on the keyboard).
-
-
Rename the app title and ID.
-
In the Solution Explorer, right-click the project name and choose Properties.
-
In the project properties dialog that appears, navigate to MAUI Shared > General.
-
Rename the Application Title to match your new namespace.
-
Following the reverse domain name and lowercase lettering conventions, modify the Application ID to reflect your new namespace and title.
-
-
Build the project.
- Choose Build > Build solution (or press <F6>).
Add a reference to WinUIEx
-
In the Visual Studio > Solution Explorer, right-click the project node and choose Edit project file.
-
Include the following package reference in the AccessServicesWithOAuth.csproj file.
AccessServicesWithOAuth.csprojUse dark colors for code blocks <!-- WinUIEx is used to workaround the lack of a WebAuthenticationBroker for WinUI. https://github.com/microsoft/WindowsAppSDK/issues/441 --> <ItemGroup Condition="'$(TargetFramework)' == 'net8.0-windows10.0.19041.0'"> <PackageReference Include="WinUIEx" Version="2.3.4" /> </ItemGroup>
-
Save and close the project file.
Platform-specific setup for WebAuthenticator functionality
The .NET MAUI WebAuthenticator helps you implement browser-based authentication flows, which listen for a callback to a specific URL registered to the app. To use the .NET MAUI Web
some platform-specific setup is required.
Windows
For WinUI 3, the callback URI protocol needs to be included in the Package.appxmanifest file. The Protocol Name
must match the URI scheme of the redirect URL
on your ArcGIS Location Platform account.
-
In the Visual Studio > Solution Explorer, navigate to the Platforms\Windows folder.
-
Open the Package.appxmanifest file in an XML (text) editor and add the following lines.
Package.appxmanifestUse dark colors for code blocks <Applications> <Application Id="App" Executable="$targetnametoken$.exe" EntryPoint="$targetentrypoint$"> <uap:VisualElements DisplayName="$placeholder$" Description="$placeholder$" Square150x150Logo="$placeholder$.png" Square44x44Logo="$placeholder$.png" BackgroundColor="transparent"> <uap:DefaultTile Square71x71Logo="$placeholder$.png" Wide310x150Logo="$placeholder$.png" Square310x310Logo="$placeholder$.png" /> <uap:SplashScreen Image="$placeholder$.png" /> </uap:VisualElements> <Extensions> <uap:Extension Category="windows.protocol"> <uap:Protocol Name="my-app"/> </uap:Extension> </Extensions> </Application> </Applications>
iOS and Mac Catalyst
The iOS and Mac Catalyst platforms require the inclusion of your app's callback URI pattern in their Info.plist files. The CF
value must match the URI scheme of the redirect URL
on your ArcGIS Location Platform account.
-
In the Visual Studio > Solution Explorer, navigate to the Platforms\iOS folder.
-
Open the Info.plist file in an XML (text) editor and add the following lines. Make the same edits in the Platforms\MacCatalyst\Info.plist file.
Info.plistUse dark colors for code blocks <key>XSAppIconAssets</key> <string>Assets.xcassets/appicon.appiconset</string> <key>CFBundleURLTypes</key> <array> <dict> <key>CFBundleURLName</key> <string>My App</string> <key>CFBundleURLSchemes</key> <array> <string>my-app</string> </array> <key>CFBundleTypeRole</key> <string>Editor</string> </dict> </array>
Android
For Android, the AndroidManifest.xml file must be updated to include the Custom
, which is required by the Web
.
-
In the Visual Studio > Solution Explorer, navigate to the Platforms\Android folder.
-
Open the AndroidManifest.xml file in an XML (text) editor and add the following lines.
AndroidManifest.xmlUse dark colors for code blocks <!--https://github.com/dotnet/maui/issues/3760#issuecomment-1046241094--> <queries> <intent> <action android:name="android.support.customtabs.action.CustomTabsService" /> </intent> </queries>
Android also requires the implementation of WebAuthenticatorCallbackActivity class. This is included in the AuthenticationHelper.cs class covered in more detail later in this tutorial.
Use dark colors for code blocks Copy [Activity(NoHistory = true, Exported = true, LaunchMode = LaunchMode.SingleTop)] [IntentFilter(new[] { Intent.ActionView }, Categories = new[] { Intent.CategoryDefault, Intent.CategoryBrowsable }, DataScheme = "my-app", DataHost = "auth")] public class WebAuthenticationCallbackActivity : WebAuthenticatorCallbackActivity { }
The Microsoft documentation covers getting started with the .NET MAUI
Web
in more detail.Authenticator
Add a traffic layer
You will add a layer to display the ArcGIS World Traffic service, a dynamic map service that presents historical and near real-time traffic information for different regions in the world. This is a secure service and requires an ArcGIS Online organizational subscription.
ArcGIS World Traffic service data is updated every five minutes to provide traffic speed and traffic incident visualization and identification.
Traffic speeds are displayed as a percentage of free-flow speeds, which is frequently the speed limit or how fast cars tend to travel when unencumbered by other vehicles. The streets are color coded as follows:
- Green (fast): 85 - 100% of free flow speeds
- Yellow (moderate): 65 - 85%
- Orange (slow); 45 - 65%
- Red (stop and go): 0 - 45%
-
In the Visual Studio > Solution Explorer, double-click MapViewModel.cs to open the file.
The project uses the Model-View-ViewModel (MVVM) design pattern to separate the application logic (view model) from the user interface (view).
Map
contains the view model class for the application, calledView Model.cs Map
. See the Microsoft documentation for more information about the Model-View-ViewModel pattern.View Model -
In the
Setup
function, update the line that sets theMap() Map
property for the view model to instead store the map in a variable. Also change the line that sets the map's initial extent to use the variable.MapViewModel.csUse dark colors for code blocks private void SetupMap() { // Create a new map with a 'topographic vector' basemap. var trafficMap = new Map(BasemapStyle.ArcGISTopographic); var mapCenterPoint = new MapPoint(-118.805, 34.027, SpatialReferences.Wgs84); trafficMap.InitialViewpoint = new Viewpoint(mapCenterPoint, 100000); }
-
Create an
ArcGISMapImageLayer
to display the traffic service and add it to the map's collection of data layers (operational layers).MapViewModel.csUse dark colors for code blocks private void SetupMap() { // Create a new map with a 'topographic vector' basemap. var trafficMap = new Map(BasemapStyle.ArcGISTopographic); var mapCenterPoint = new MapPoint(-118.805, 34.027, SpatialReferences.Wgs84); trafficMap.InitialViewpoint = new Viewpoint(mapCenterPoint, 100000); // Create a layer to display the ArcGIS World Traffic service. var trafficServiceUrl = "https://traffic.arcgis.com/arcgis/rest/services/World/Traffic/MapServer"; var trafficLayer = new ArcGISMapImageLayer(new Uri(trafficServiceUrl)); // Add the traffic layer to the map's data layer collection. trafficMap.OperationalLayers.Add(trafficLayer); }
-
Set the view model's
Map
property. Data binding handles displaying the map in the view.MapViewModel.csUse dark colors for code blocks private void SetupMap() { // Create a new map with a 'topographic vector' basemap. var trafficMap = new Map(BasemapStyle.ArcGISTopographic); var mapCenterPoint = new MapPoint(-118.805, 34.027, SpatialReferences.Wgs84); trafficMap.InitialViewpoint = new Viewpoint(mapCenterPoint, 100000); // Create a layer to display the ArcGIS World Traffic service. var trafficServiceUrl = "https://traffic.arcgis.com/arcgis/rest/services/World/Traffic/MapServer"; var trafficLayer = new ArcGISMapImageLayer(new Uri(trafficServiceUrl)); // Add the traffic layer to the map's data layer collection. trafficMap.OperationalLayers.Add(trafficLayer); // Set the view model Map property with the new map. this.Map = trafficMap; }
-
Click Debug > Start Debugging (or press <F5> on the keyboard) to run the app.
If creating apps for Android or iOS, you will need the appropriate emulator, simulator, or device configured for testing (see System requirements for details)
You should see a map with the topographic basemap layer centered on the Santa Monica Mountains in California. The traffic layer doesn't appear because it requires authentication with ArcGIS Online.
Handle layer load errors
If a map attempts to load a layer based on a secured service without the required authentication, the layer is not added to the map. No exception is raised and unless you handle it in your code, the user may never know that the secured resource is missing from the map.
You will handle load status change events for the traffic layer and report an error message if the layer fails to load.
-
In the
Setup
function, after the line that creates the traffic layer, add the following line of code to handle the layer's load status changed event. You will create the code for this handler in another step.Map() MapViewModel.csUse dark colors for code blocks private void SetupMap() { // Create a new map with a 'topographic vector' basemap. var trafficMap = new Map(BasemapStyle.ArcGISTopographic); var mapCenterPoint = new MapPoint(-118.805, 34.027, SpatialReferences.Wgs84); trafficMap.InitialViewpoint = new Viewpoint(mapCenterPoint, 100000); // Create a layer to display the ArcGIS World Traffic service. var trafficServiceUrl = "https://traffic.arcgis.com/arcgis/rest/services/World/Traffic/MapServer"; var trafficLayer = new ArcGISMapImageLayer(new Uri(trafficServiceUrl)); // Handle changes in the traffic layer's load status to check for errors. trafficLayer.LoadStatusChanged += TrafficLayer_LoadStatusChanged; // Add the traffic layer to the map's data layer collection. trafficMap.OperationalLayers.Add(trafficLayer); // Set the view model Map property with the new map. this.Map = trafficMap; }
-
Below the
Setup
function, create the function that handles load status change events for the layer. If the layer fails to load, display an error message to the user.Map() MapViewModel.csUse dark colors for code blocks private async void TrafficLayer_LoadStatusChanged(object sender, Esri.ArcGISRuntime.LoadStatusEventArgs e) { // Report the error message if the traffic layer fails to load. if (e.Status == Esri.ArcGISRuntime.LoadStatus.FailedToLoad) { var trafficLayer = sender as ArcGISMapImageLayer; await MainThread.InvokeOnMainThreadAsync(() => { Application.Current.MainPage.DisplayAlert("Traffic layer did not load", trafficLayer?.LoadError?.Message, "Ok"); }); } }
-
Click Debug > Start Debugging (or press <F5> on the keyboard) to run the app.
If creating apps for Android or iOS, you will need the appropriate emulator, simulator, or device configured for testing (see System requirements for details)
You should see the same map display, but this time a message box reports a "Token required" error when the traffic layer fails to load. An access token is an authorization string that provides secure access to content, data, and functionality in ArcGIS location services. To load the traffic service layer, you will use OAuth to get an access token based on ArcGIS Online credentials.
Implement user authentication using OAuth credentials
This API abstracts some of the details for user authentication using OAuth credentials in your app. You can use classes such as AuthenticationManager
to request, store, and manage credentials for secure resources.
You will add an authentication helper class that uses AuthenticationManager
and encapsulates additional authentication logic.
-
From the Visual Studio Project menu, choose Add class .... Name the class AuthenticationHelper.cs then click Add. The new class is added to your project and opens in Visual Studio.
-
Select all the code in the class and delete it.
-
Copy all of the code below and paste it into the AuthenticationHelper.cs class in your project.
AuthenticationHelper.csUse dark colors for code blocks Copy // Copyright 2023 Esri // Licensed under the Apache License, Version 2.0 (the "License"); // you may not use this file except in compliance with the License. // You may obtain a copy of the License at // // https://www.apache.org/licenses/LICENSE-2.0 // // Unless required by applicable law or agreed to in writing, software // distributed under the License is distributed on an "AS IS" BASIS, // WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. // See the License for the specific language governing permissions and // limitations under the License. #if ANDROID using Android.App; using Application = Microsoft.Maui.Controls.Application; using Android.Content; using Android.Content.PM; #endif using Esri.ArcGISRuntime.Security; using System; using System.Collections.Generic; using System.Diagnostics; using System.Threading.Tasks; namespace AccessServicesWithOAuth { // A helper class that manages authenticating with a server to access secure resources. internal static class AuthenticationHelper { // Specify the Client ID and Redirect URL to use for OAuth authentication. // Sign in with your ArcGIS Location Platform or ArcGIS Online account // (https://www.arcgis.com/home/signin.html) to create your OAuth Application item. private const string OAuthClientID = "YOUR_CLIENT_ID"; private const string OAuthRedirectUrl = "YOUR_REDIRECT_URL"; static AuthenticationHelper() { // Use the OAuthAuthorize class (defined below) to show the login UI. AuthenticationManager.Current.OAuthAuthorizeHandler = new OAuthAuthorize(); // Create a new ChallengeHandler that uses a method in this class to challenge for credentials. AuthenticationManager.Current.ChallengeHandler = new ChallengeHandler(PromptCredentialAsync); } // A function to register a secure server with the AuthenticationManager. // Pass in the URL of the server containing secure resources and, optionally, a client ID and // redirect URL (if not specified, the values defined above are used). public static void RegisterSecureServer(string url, string clientID = OAuthClientID, string redirectUrl = OAuthRedirectUrl) { // Define the server URL, authentication type, client ID, and redirect URL. ServerInfo portalServerInfo = new ServerInfo(new Uri(url)) { TokenAuthenticationType = TokenAuthenticationType.OAuthAuthorizationCode, OAuthClientInfo = new OAuthClientInfo(clientID, new Uri(redirectUrl)) }; // Register the server information with the AuthenticationManager. AuthenticationManager.Current.RegisterServer(portalServerInfo); } // A function that adds a credential to AuthenticationManager based on a temporary token. // This is useful for testing an app with secured services without having to log in. public static void ApplyTemporaryToken(string url, string token) { // Create a new OAuth credential for the specified URL with the token. OAuthTokenCredential tempToken = new OAuthTokenCredential(new Uri(url), token); // Add the credential to the AuthenticationManager. AuthenticationManager.Current.AddCredential(tempToken); } // The ChallengeHandler function that is called when access to a secured resource is attempted. public static async Task<Credential> PromptCredentialAsync(CredentialRequestInfo info) { Credential credential = null; try { // Get credentials for the specified server. The OAuthAuthorize class (defined below) // will get the user's credentials (show the login window and handle the response). credential = await AuthenticationManager.Current.GenerateCredentialAsync(info.ServiceUri); } catch (OperationCanceledException) { // Login was cancelled, no need to display an error to the user. } return credential; } #region OAuth handler // In a .NET MAUI app, an IOAuthAuthorizeHandler component is used to handle some of // the OAuth details. Specifically, it implements AuthorizeAsync to show the login UI // (generated by the server that hosts secure content) in a WebAuthenticator. When the user // logs in successfully, cancels the login, or closes the window without continuing, the // IOAuthAuthorizeHandler is responsible for obtaining the authorization from the server // or raising an OperationCanceledException. public class OAuthAuthorize : IOAuthAuthorizeHandler { // Function to initiate an authorization request. It takes the URIs for: the secured service, // the authorization endpoint, and the redirect URI. #if MACCATALYST || IOS public Task<IDictionary<string, string>> AuthorizeAsync(Uri serviceUri, Uri authorizeUri, Uri callbackUri) { // Use a TaskCompletionSource to track the completion of the authorization. var tcs = new TaskCompletionSource<IDictionary<string, string>>(); Microsoft.Maui.ApplicationModel.MainThread.BeginInvokeOnMainThread(async () => { try { var result = await WebAuthenticator.AuthenticateAsync(authorizeUri, callbackUri); tcs.TrySetResult(result.Properties); } catch (Exception ex) { tcs.TrySetException(ex); } }); return tcs.Task; } #elif ANDROID public async Task<IDictionary<string, string>> AuthorizeAsync(Uri serviceUri, Uri authorizeUri, Uri callbackUri) { var result = await WebAuthenticator.AuthenticateAsync(authorizeUri, callbackUri); return result.Properties; } #elif WINDOWS // WebAuthenticator does not currently work on Windows without the WinUIEx library. // https://learn.microsoft.com/en-us/dotnet/maui/platform-integration/communication/authentication?tabs=windows public async Task<IDictionary<string, string>> AuthorizeAsync(Uri serviceUri, Uri authorizeUri, Uri callbackUri) { var result = await WinUIEx.WebAuthenticator.AuthenticateAsync(authorizeUri, callbackUri); return result.Properties; } #endif #if ANDROID // Android requires an Intent Filter setup to handle your callback URI. // This is accomplished by inheriting from the WebAuthenticatorCallbackActivity class. // https://learn.microsoft.com/en-us/dotnet/maui/platform-integration/communication/authentication?tabs=android [Activity(NoHistory = true, Exported = true, LaunchMode = LaunchMode.SingleTop)] [IntentFilter(new[] { Intent.ActionView }, Categories = new[] { Intent.CategoryDefault, Intent.CategoryBrowsable }, DataScheme = "my-app", DataHost = "auth")] public class WebAuthenticationCallbackActivity : WebAuthenticatorCallbackActivity { } #endif #endregion OAuth handler } } }
-
Near the top of the file, add your values for
Client ID
(O
) andAuth Client ID Redirect URL
(O
). These were created with your OAuth application metadata in an earlier step and are unique to your application.Auth Redirect Url AuthenticationHelper.csUse dark colors for code blocks // A helper class that manages authenticating with a server to access secure resources. internal static class AuthenticationHelper { // Specify the Client ID and Redirect URL to use for OAuth authentication. // Sign in with your ArcGIS Location Platform or ArcGIS Online account // (https://www.arcgis.com/home/signin.html) to create your OAuth Application item. private const string OAuthClientID = "YOUR_CLIENT_ID"; private const string OAuthRedirectUrl = "YOUR_REDIRECT_URL";
The Authentication
class is now set up to handle OAuth authentication in your app. This class is generic enough that you can use it in any app, as long as valid client ID and redirect URL values are provided.
The last step is to use Authentication
to register one or more server URLs with AuthenticationManager
to authenticate the user for secure resources from those servers.
Register ArcGIS Online for user authentication
Registering a portal with AuthenticationManager
ensures that the user will be authenticated for any secured services on that portal. The portal is registered using its URL and the type of authentication it requires (one of the token authentication types supported for ArcGIS). For OAuth authentication, you must also provide OAuth Application item details, such as the Client ID
and Redirect URL
.
-
In the Visual Studio > Solution Explorer, double-click MapViewModel.cs to open the file.
-
In the
Setup
function, add a line of code that usesMap() Authentication
to register ArcGIS Online for authentication.Helper MapViewModel.csUse dark colors for code blocks private void SetupMap() { // Add the ArcGIS Online URL to the authentication helper. AuthenticationHelper.RegisterSecureServer("https://www.arcgis.com/sharing/rest"); // Create a new map with a 'topographic vector' basemap. var trafficMap = new Map(BasemapStyle.ArcGISTopographic); var mapCenterPoint = new MapPoint(-118.805, 34.027, SpatialReferences.Wgs84); trafficMap.InitialViewpoint = new Viewpoint(mapCenterPoint, 100000); // Create a layer to display the ArcGIS World Traffic service. var trafficServiceUrl = "https://traffic.arcgis.com/arcgis/rest/services/World/Traffic/MapServer"; var trafficLayer = new ArcGISMapImageLayer(new Uri(trafficServiceUrl)); // Handle changes in the traffic layer's load status to check for errors. trafficLayer.LoadStatusChanged += TrafficLayer_LoadStatusChanged; // Add the traffic layer to the map's data layer collection. trafficMap.OperationalLayers.Add(trafficLayer); // Set the view model Map property with the new map. this.Map = trafficMap; }
-
Click Debug > Start Debugging (or press <F5> on the keyboard) to run the app.
If creating apps for Android or iOS, you will need the appropriate emulator, simulator, or device configured for testing (see System requirements for details)
You should again see a map with the topographic basemap layer centered on the Santa Monica Mountains in California. You will be prompted for an ArcGIS Online username and password. Once you authenticate successfully with ArcGIS Online, the traffic layer will appear in the map.
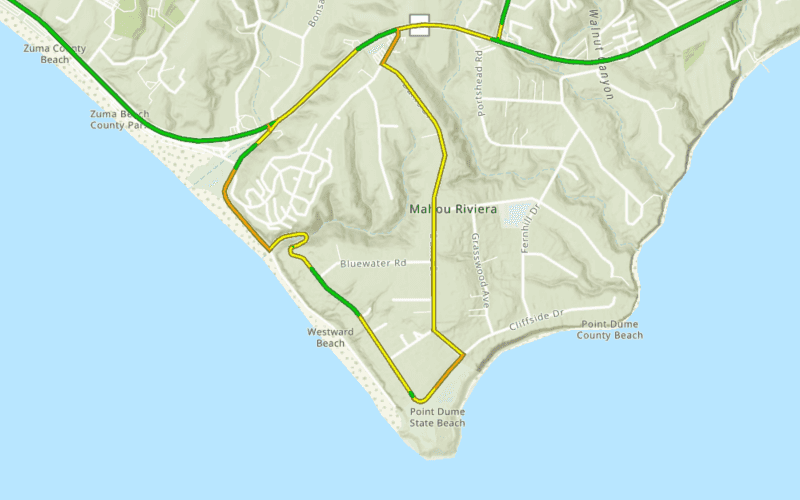
Testing apps that require authentication
When you are testing apps that require authentication for one or more services in the map, it can become monotonous to enter your OAuth credentials repeatedly. You can programmatically add authentication for a server using the temporary token
provided with your OAuth Application item details. As the name suggests, this token is valid for a limited time, but you can always get a fresh token from ArcGIS.com using your ArcGIS Account.
The Authentication
has the following function for applying a temporary token.
// A function that adds a credential to AuthenticationManager based on a temporary token.
// This is useful for testing an app with secured services without having to log in.
public static void ApplyTemporaryToken(string url, string token)
{
// Create a new OAuth credential for the specified URL with the token.
OAuthTokenCredential tempToken = new OAuthTokenCredential(new Uri(url), token);
// Add the credential to the AuthenticationManager.
AuthenticationManager.Current.AddCredential(tempToken);
}
You can call the function with code such as:
AuthenticationHelper.ApplyTemporaryToken("https://www.arcgis.com/sharing/rest", "YOUR_TEMP_TOKEN");
Until the token expires, you can run the app without being challenged to provide your credentials.
Additional resources
If you are implementing OAuth for another .NET platform, you might find some of the following resources helpful.
- ArcGIS Maps SDK for .NET OAuth sample: The WPF version of this sample uses code similar to code used in this tutorial. The sample is available for other .NET platforms (use the switcher control at the top of the page) to illustrate implementing OAuth for apps targeting WinUI, UWP, and .NET MAUI.
- Web authentication broker: Use this authentication broker to implement OAuth in your Universal Windows Platform (UWP) apps.