June 2021
WFSLayer
The WFSLayer allows you to create a layer from an OGC Web Feature Service (WFS). The next generation of WFS, OGC API - Features, is supported via the OGCFeatureLayer.
You can configure the renderer, popup, and labels of a WFSLayer using a similar pattern as the FeatureLayer. Users can work with features in the WFS service using the same query and Smart Mapping APIs as FeatureLayer.
We also added utility functions that allow you to browse FeatureTypes in the WFS service before creating the layer. You can learn more about these methods in the wfsUtils documentation.
The WFS service must support WFS 2.0.0 and have GeoJSON output format enabled.
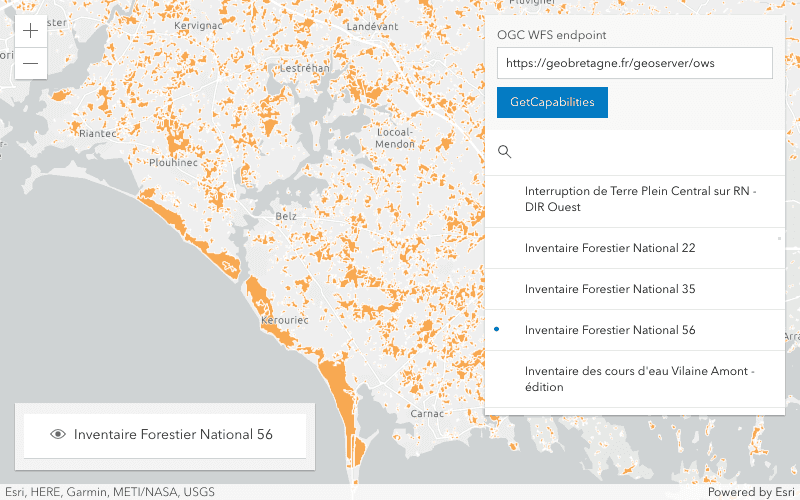
3D updates
Shadow accumulation widget
Analyze the total shadow duration from buildings and other 3D objects in your scene. The new ShadowAccumulation widget provides different visualization types for a selected time range and date. Analysis options like thresholds help to quickly assess if a scene complies with urban shadow impact regulations.
Try out the new analysis widget for different development scenarios using a new sample.
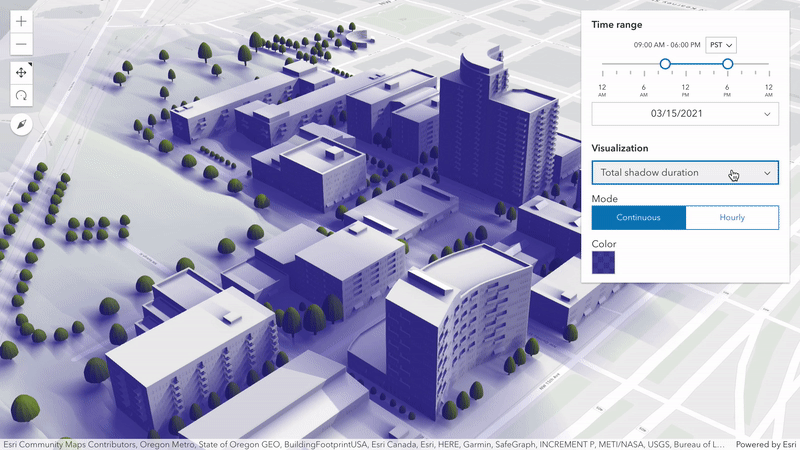
Edge offset
Polygon edges and Polyline
segments can now be offset using new manipulators provided by the
SketchViewModel. The operation
allows you to horizontally push and pull edges while maintaining the shape of the geometry.
In addition, new settings in
Sketch
allow to you configure the available manipulators when updating the overall shape or individual vertices.
The updated Sketch in 3D sample shows how to change these manipulators.
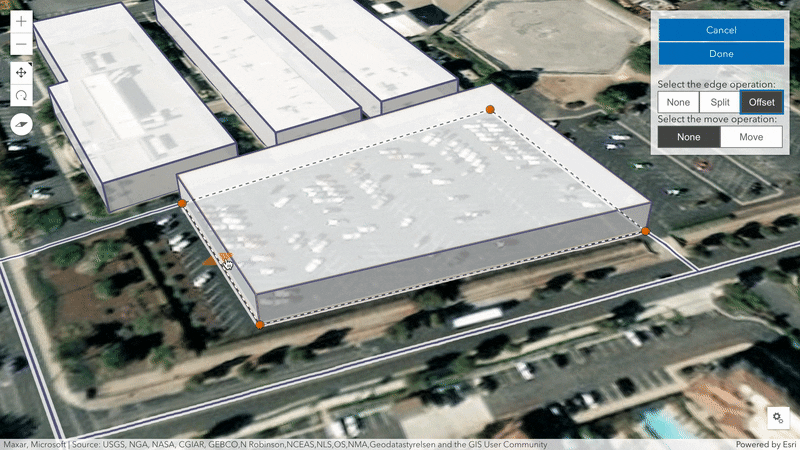
Analysis layers (beta)
Display measurement results using two new analysis layers:
Direct
and
Area
. Create new, custom,
interactive analysis experiences by programmatically providing the measurement points. The
analysis layers will display one or multiple results in the scene using the same visualization known
from DirectLineMeasurement3D and
AreaMeasurement3D.
A new sample was added that shows how to measure parcels using AreaMeasurementLayer.
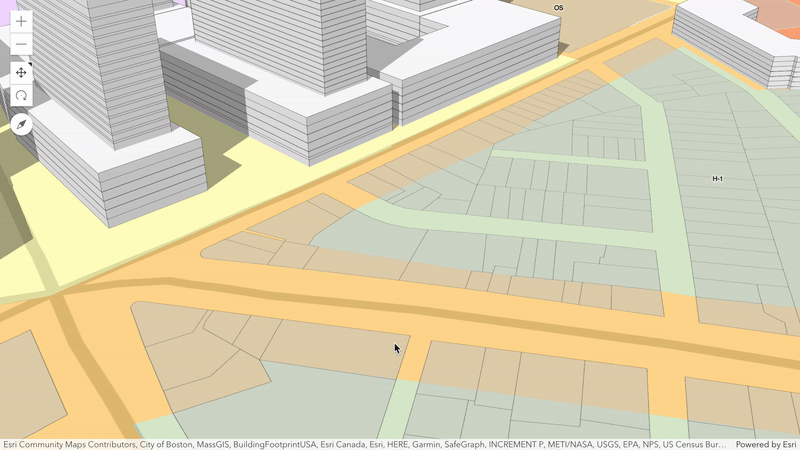
Feature table support for scene layers
Provide a tabular view for the features of a SceneLayer using the FeatureTable widget. Users can interactively select the visible attribute columns and sort them based on the values. The widget requires a scene layer with an associated feature layer. Enable editing on the layer to update the attribute values directly in the table.
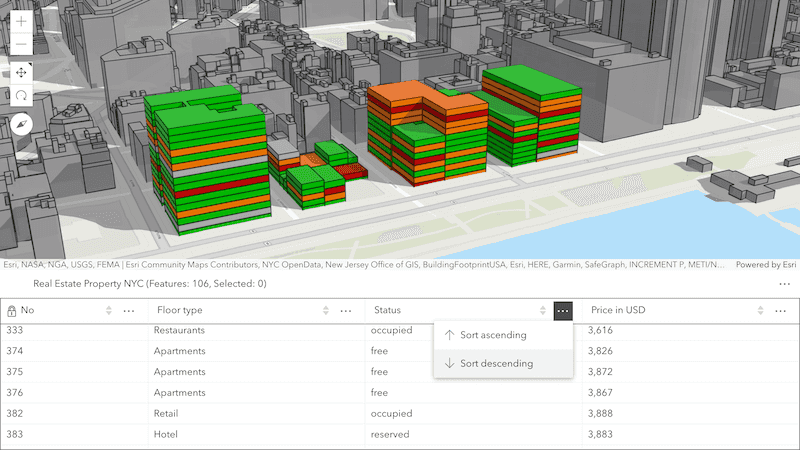
Utility network support
Over the next couple of releases, we are working on a multi-phase project to add support for utility network. In this release, we implemented the first phase, which added initial support for utility network, viewing associations, SubtypeGroupLayer, and simple network tracing through named trace configuration. The subsequent phases will be implemented over the coming releases and will include support for more complete tracing, subnetwork management, and advanced editing.
SubtypeGroupLayer
The SubtypeGroupLayer was added to improve the experience with datasets that currently contain feature layers with many subtypes (such as the utility network datasets).
The Subtype
is a single, composite FeatureLayer that contains a sublayer for each subtype in the individual feature service, known as a SubtypeSublayer.
This allows for configuring layer properties
on each sublayer, such as visibility
, renderer
, popup
, min
, and max
.
The Subtype
is much more efficient than an equivalent set of individual FeatureLayers because it makes less requests.
The following GIF illustrates the performance difference between loading a dataset with each subtype as its own Feature
versus loading a Subtype
.
As you can see on initial load and after zooming, there are less requests sent in much less time using the Subtype
as compared to loading individual layers.
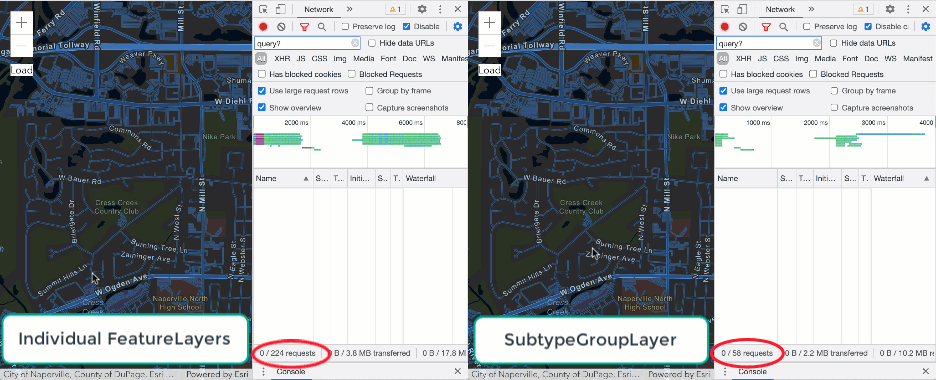
Simple network tracing
We added support for simple network tracing through named trace configuration.
The utility network's tracing function
traces your network to optimize paths for resources to travel. It provides a framework that can be used to help deliver resources to customers, track the health of a network, and identify deteriorating areas.
To perform a trace, load a WebMap containing trace configurations, and use the trace
method.
rest modules
At 4.19, we ported the majority of Task functionality to simple functional rest modules. This made it easier to incorporate into a more modular app design, and also reduced unnecessary dependencies (you only import what you want). At this release, we ported the rest of the Task and Task support classes to rest modules, and deprecated the Task and Task support classes (which will still remain available until early next year, and then will be removed from the API). We also added a new TravelMode class to make it easier to understand how to define how an object, like a vehicle, bicycle, or pedestrian, moves along a street network.
The new rest modules are functions, which do not require constructors, so you can use their methods immediately. Also, because of the more
modular design, you can import just one or more methods of a rest module, instead of having to import the whole module.
Here is an example of using the locator
module where we only want to use the suggest
method:
require([
"esri/rest/locator/suggestLocations", ...
], function(suggestLocations, ... ) {
Now we don't need to import the entire locator
module, just the method of interest.
This speeds up our build time, and makes our code more focused.
Expand the code snippets below to compare the differences between using a Task and a rest module:
Here is an example of using the deprecated Find
:
require([
"esri/tasks/FindTask", "esri/tasks/support/FindParameters", ...
], (FindTask, FindParameters, ... ) => {
let url: "url";
let params = new FindParameters({
layerIds: [1],
searchFields: ["teamName"],
outSpatialReference: { wkid: 4326 },
returnGeometry: true
});
const findTask = new FindTask(url);
findTask.execute(params).then(function(results){
// Results contain FindResults of findTask
});
});
And here is the same example, but using the new find
method:
require([
"esri/rest/find", "esri/rest/support/FindParameters", ...
], (find, FindParameters, ... ) => {
let url: "url";
let params = new FindParameters({
layerIds: [1],
searchFields: ["teamName"],
outSpatialReference: { wkid: 4326 },
returnGeometry: true
});
find(url, params).then(function(results){
// Results contain FindResults of find
});
});
API keys
Over the past couple of releases, we added support for a global API key (4.18) to use with Location Services and fine-grained support for directions & routing (4.19). Version 4.20 builds on top of this by adding fine-grained control of API keys for FeatureLayer, SceneLayer, TileLayer, OGCFeatureLayer, BuildingSceneLayer, IntegratedMeshLayer, and PointCloudLayer. Access to private content via API keys is only for development and testing at this time.
We also improved support for API keys in the Directions and Search widgets.
Now, both the routing and geocoding components default to the appropriate URL if an api
is defined.
Layer updates
FeatureLayer
Support for query top features
At this release, we added queryTopFeatures(), queryTopFeatureCount(), queryTopFeatureObjectIds(), and queryTopFeatureExtent() on FeatureLayer that will allow you to query top features from a server side FeatureLayers. For example, you can use one of the four methods to group counties of United States by state name, order them in descending order by the population, and then return the first three most populous counties in each state.
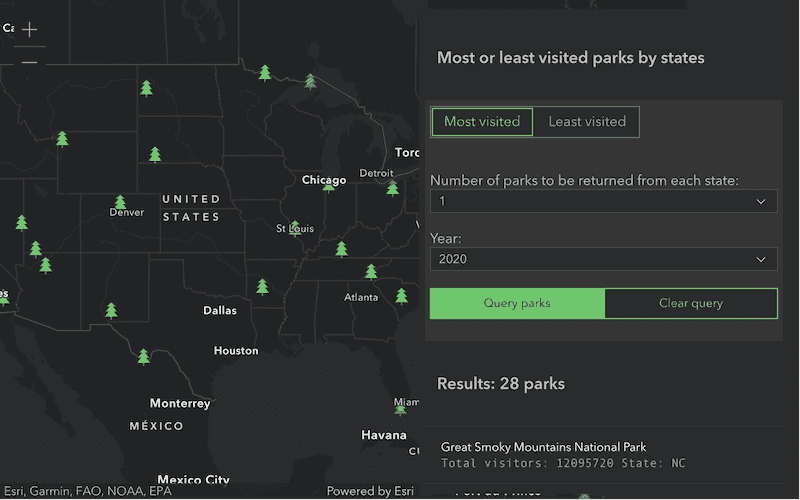
Apply edits
We also updated the applyEdits() method to support edits to features associated with multiple layers or tables in a single call.
The return
and return
parameters were added to make this possible. The return
parameter can return all features edited due to the database behavior
that results from applying the edits. For example, if a feature is deleted and this parameter is set to original-and-current-features
, the deleted destination feature is returned along
with a reference to the deleted origin feature in the response.
VectorTileLayer
At this release, we added a support for SDF dashed lines and data-driven line and fill patterns for VectorTileLayer. We have also improved performance of vector tiles when panning.
OGCFeatureLayer
The OGCFeatureLayer can now be saved in a WebMap.
WMSLayer dimensions
Added support for time dimensions on WMSLayer and WMSSublayer. Time dimensions can be used to set the View.timeExtent, WMSLayer.timeExtent, or to configure a TimeSlider widget.
ImageryLayer.getSamples()
We added getSamples() method on ImageryLayer. This method returns sample point locations, pixel values and corresponding resolutions of the source data for a given geometry. For example, you can construct a wind rose chart that shows the most frequent wind speeds and directions at the specified location for given number of years.
Widget updates
General widget enhancements
- Added a
visible
property to all widgets. When set tofalse
, the widget will not render. - Added a
heading
property to some widgets. This property allows you to change the level for heading elements in the widgets (e.g.Level <h2>
). Depending on the widget's placement in your app, you may need to adjust the heading level for proper semantics. This is important for meeting accessibility standards.Popup title< /h2>
Editor and Sketch widgets - snapping enhancements
Snapping is now implemented when moving 2D point geometries in both the Editor and Sketch widgets.
TimeSlider widget
Starting at 4.20, when the TimeSlider widget settings are invalid, the current time segment and thumbs will be drawn in a red color with a message indicating the issue. TimeSlider's values property is deprecated at 4.20. Use the timeExtent property instead.

FeatureForm widget
The Switch input was added as an additional type to FormTemplate field elements.
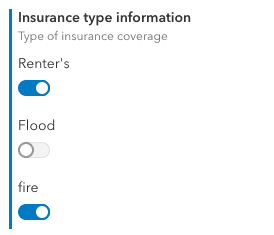
ElevationProfile widget
The ElevationProfile widget now has the ability to clear a drawn line with the click of the trash icon. This can be toggled on with the clear
property on Elevation
. There is also a uniform chart scaling option which toggles a checkbox that allows the profile to be shown on the same scale along the X and Y axis, as if it were a cross section. This is useful to see the real shape of buildings or mountains and can be toggled with the uniform
property on Elevation
.
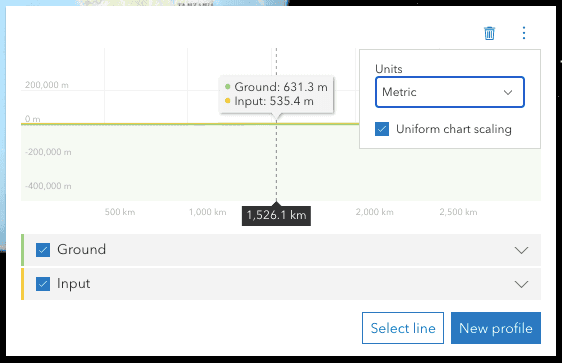
The zoom functionality has changed so that the user can click and drag a rectangle to zoom into, rather than just a range along the x axis. The ElevationProfile also now handles multi part lines, has per-profile toggles and stats and improved slope calculation.
CoordinateConversion widget
The CoordinateConversion widget now uses session
instead of local
to save its state for better memory management.
This is configurable with the new storageEnabled property.
CIMSymbols
You can now set scale values on CIMSymbols using the min
and max
properties on CIMSymbol.data
.
const cimSymbol = new CIMSymbol({
data: {
type: "CIMSymbolReference",
minScale: 750000, // only allow the symbol to be shown at certain scales
maxScale: 80000,
symbol: {
type: "CIMLineSymbol",
symbolLayers: [{ ... }]
},
}
});
Smart mapping updates
ImageryLayer support
We added support for generating the following renderers for imagery layers:
Each of these renderer creator modules can be found in the esri/smart
folder.
Slider enhancements
Smart mapping sliders added an interactive track that allows users to drag each slider's segments. When syncedSegmentsEnabled is true
, you can drag all segments in sync with the primary handle. This is particularly valuable in sliders that represent above-and-below data. Rather than allowing the primary handle modify the positions of other handles (i.e. when primaryHandleEnabled is true), you can keep handle positions independent of one another while still providing a way for the user to modify all handles at once.
Prior to this enhancement, a user could unintentionally modify other slider handles with the primary handle. Note in the image below how modifying the primary handle's value alters the positions of other handles. This can be undesirable if the user already entered a meaningful value on the other handles.
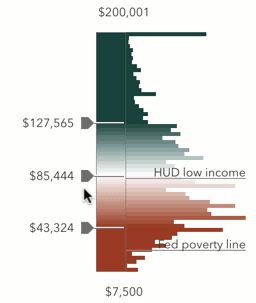
With the interactive track, you can retain the syncing behavior on drag, and allow users to modify handle values without fear of the primary handle moving other values previously set by the user.
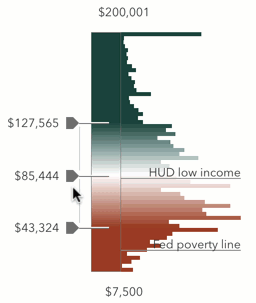
Default label formatting also improved to use compact notation for large numbers. Slider handles are also no longer constrained to the segment boundaries; you can freely move any slider handle to any position along the track.
Increased zoom limits
It is now possible to increase the max zoom level on the map with the addition of the num
parameter on Tile
.
In order to do this, additional LODs can be created via the TileInfo.create() method and passed into the MapView's constraints.
Added classes, properties, methods, events
- esri/form/elements/inputs/SwitchInput
- esri/layers/AreaMeasurementLayer
- esri/layers/DirectLineMeasurementLayer
- esri/layers/ogc/wfsUtils
- esri/layers/SubtypeGroupLayer
- esri/layers/support/SubtypeSublayer
- esri/layers/WFSLayer
- esri/networks/Network
- esri/networks/UtilityNetwork
- esri/rest/networkService
- esri/rest/print
- esri/rest/support/AddressCandidate
- esri/rest/support/AlgorithmicColorRamp
- esri/rest/support/AreasAndLengthsParameters
- esri/rest/support/AttachmentQuery
- esri/rest/support/BufferParameters
- esri/rest/support/ClosestFacilityParameters
- esri/rest/support/ClosestFacilitySolveResult
- esri/rest/support/ColorRamp
- esri/rest/support/DataFile
- esri/rest/support/DataLayer
- esri/rest/support/DensifyParameters
- esri/rest/support/DirectionsFeatureSet
- esri/rest/support/DistanceParameters
- esri/rest/support/FeatureSet
- esri/rest/support/FindParameters
- esri/rest/support/FindResult
- esri/rest/support/GeneralizeParameters
- esri/rest/support/GPMessage
- esri/rest/support/IdentifyParameters
- esri/rest/support/IdentifyResult
- esri/rest/support/ImageHistogramParameters
- esri/rest/support/ImageIdentifyParameters
- esri/rest/support/ImageIdentifyResult
- esri/rest/support/ImageSample
- esri/rest/support/ImageSampleParameters
- esri/rest/support/ImageSampleResult
- esri/rest/support/JobInfo
- esri/rest/support/LegendLayer
- esri/rest/support/LengthsParameters
- esri/rest/support/LinearUnit
- esri/rest/support/MultipartColorRamp
- esri/rest/support/NAMessage
- esri/rest/support/OffsetParameters
- esri/rest/support/ParameterValue
- esri/rest/support/PrintParameters
- esri/rest/support/PrintTemplate
- esri/rest/support/ProjectParameters
- esri/rest/support/Query
- esri/rest/support/RasterData
- esri/rest/support/RelationParameters
- esri/rest/support/RelationshipQuery
- esri/rest/support/RouteParameters
- esri/rest/support/RouteResult
- esri/rest/support/ServiceAreaParameters
- esri/rest/support/ServiceAreaSolveResult
- esri/rest/support/StatisticDefinition
- esri/rest/support/TopFeaturesQuery
- esri/rest/support/TopFilter
- esri/rest/support/TravelMode
- esri/rest/support/TrimExtendParameters
- esri/smartMapping/raster/renderers/classBreaks
- esri/smartMapping/raster/renderers/colormap
- esri/smartMapping/raster/renderers/rgb
- esri/smartMapping/raster/renderers/shadedRelief
- esri/smartMapping/raster/renderers/stretch
- esri/smartMapping/raster/renderers/uniqueValue
- esri/smartMapping/raster/renderers/vectorField
- esri/smartMapping/raster/support/utils
- esri/views/3d/layers/AreaMeasurementLayerView3D
- esri/views/3d/layers/DirectLineMeasurementLayerView3D
- esri/views/layers/WFSLayerView
- esri/widgets/ShadowAccumulation
- esri/widgets/ShadowAccumulation/ShadowAccumulationViewModel
- Added event:
segment-drag
to esri/widgets/smartMapping/ClassedColorSlider, esri/widgets/smartMapping/ClassedSizeSlider, esri/widgets/smartMapping/ColorSizeSlider, esri/widgets/smartMapping/ColorSlider, esri/widgets/smartMapping/OpacitySlider, esri/widgets/smartMapping/SizeSlider, esri/widgets/smartMapping/SmartMappingSliderBase - Added events: max-click, min-click, segment-click, thumb-click, tick-click, track-click to esri/widgets/Slider
- Added method:
get
to esri/layers/ImageryLayer, esri/rest/imageServiceSamples - Added method:
identify
to esri/rest/imageService - Added method:
i
to esri/widgets/support/widgets Activation Key - Added method:
query
to esri/layers/FeatureLayerT o p Feature Count - Added property:
active
to esri/widgets/Sketch/SketchViewModelFill Symbol - Added property:
api
to esri/layers/FeatureLayer, esri/layers/OGCFeatureLayer, esri/layers/TileLayer, esri/portal/PortalItem, esri/layers/SceneLayer, esri/layers/IntegratedMeshLayer, esri/layers/PointCloudLayer, esri/layers/BuildingSceneLayerKey - Added property:
custom
to esri/layers/ImageryLayer, esri/layers/ImageryTileLayer, esri/layers/OGCFeatureLayerParameters - Added property:
dimensions
to esri/layers/support/WMSSublayer, esri/layers/WMSLayer - Added property:
editable
to esri/Map, esri/WebMap, esri/WebSceneLayers - Added property:
extra
to esri/widgets/Print, esri/widgets/Print/PrintViewModelParameters - Added property:
features
to esri/widgets/Popup/PopupViewModelP e r Page - Added property:
fields
to esri/layers/WCSLayer - Added property:
heading
to esri/widgets/BasemapGallery, esri/widgets/BasemapLayerList, esri/widgets/Bookmarks, esri/widgets/BuildingExplorer, esri/widgets/CoordinateConversion, esri/widgets/Daylight, esri/widgets/Directions, esri/widgets/Editor, esri/widgets/Feature, esri/widgets/FeatureForm, esri/widgets/FeatureTemplates, esri/widgets/FloorFilter, esri/widgets/Legend, esri/widgets/Popup, esri/widgets/Print, esri/widgets/SliceLevel - Added property:
highlight
to esri/widgets/ElevationProfile/ElevationProfileViewModelEnabled - Added property:
label
to esri/widgets/SliderElements - Added property:
location
to esri/widgets/CoordinateConversionSymbol - Added property:
max
to esri/widgets/SliderLabel Element - Added property:
min
to esri/widgets/SliderLabel Element - Added property:
multidimensional
to esri/layers/ImageryTileLayerDefinition - Added property:
off
to esri/widgets/FeatureForm/FieldConfig, esri/widgets/FeatureTable/FieldColumnConfigValue - Added property:
o
to esri/widgets/FeatureForm/FieldConfig, esri/widgets/FeatureTable/FieldColumnConfign Value - Added property:
portal
to esri/layers/OGCFeatureLayerItem - Added property:
processed
to esri/tasks/support/ImageIdentifyResultValues - Added property:
raster
to esri/layers/ImageryTileLayerFields - Added property:
segment
to esri/widgets/SliderElements - Added property:
storage
to esri/widgets/CoordinateConversion, esri/widgets/CoordinateConversion/CoordinateConversionViewModelEnabled - Added property:
synced
to esri/widgets/Slider, esri/widgets/smartMapping/ClassedColorSlider, esri/widgets/smartMapping/ClassedSizeSlider, esri/widgets/smartMapping/ColorSizeSlider, esri/widgets/smartMapping/ColorSlider, esri/widgets/smartMapping/OpacitySlider, esri/widgets/smartMapping/SizeSlider, esri/widgets/smartMapping/SmartMappingSliderBaseSegments Enabled - Added property:
thumb
to esri/widgets/SliderElements - Added property:
tick
to esri/widgets/SliderElements - Added property:
uniform
to esri/widgets/ElevationProfile/ElevationProfileViewModelChart Scaling - Added property:
utility
to esri/WebMapNetworks - Added property:
view
to esri/widgets/ElevationProfile/ElevationProfileLine, esri/widgets/ElevationProfile/ElevationProfileLineGround, esri/widgets/ElevationProfile/ElevationProfileLineInput, esri/widgets/ElevationProfile/ElevationProfileLineQuery, esri/widgets/ElevationProfile/ElevationProfileLineViewVisualization Enabled - Added property:
visible
to esri/widgets/AreaMeasurement2D, esri/widgets/Attachments, esri/widgets/Attribution, esri/widgets/BasemapGallery, esri/widgets/BasemapLayerList, esri/widgets/BasemapToggle, esri/widgets/Bookmarks, esri/widgets/BuildingExplorer, esri/widgets/Compass, esri/widgets/CoordinateConversion, esri/widgets/Daylight, esri/widgets/Directions, esri/widgets/DistanceMeasurement2D, esri/widgets/Editor, esri/widgets/ElevationProfile, esri/widgets/Expand, esri/widgets/Feature, esri/widgets/FeatureForm, esri/widgets/FeatureTable, esri/widgets/FeatureTable/Grid/support/ButtonMenu, esri/widgets/FeatureTemplates, esri/widgets/FloorFilter, esri/widgets/Fullscreen, esri/widgets/Histogram, esri/widgets/HistogramRangeSlider, esri/widgets/Home, esri/widgets/LayerList, esri/widgets/Legend, esri/widgets/LineOfSight, esri/widgets/Locate, esri/widgets/Measurement, esri/widgets/NavigationToggle, esri/widgets/Print, esri/widgets/ScaleBar, esri/widgets/ScaleRangeSlider, esri/widgets/Search, esri/widgets/Search/SearchResultRenderer, esri/widgets/Sketch, esri/widgets/Slice, esri/widgets/Slider, esri/widgets/smartMapping/ClassedColorSlider, esri/widgets/smartMapping/ClassedSizeSlider, esri/widgets/smartMapping/ColorSizeSlider, esri/widgets/smartMapping/ColorSlider, esri/widgets/smartMapping/HeatmapSlider, esri/widgets/smartMapping/OpacitySlider, esri/widgets/smartMapping/SizeSlider, esri/widgets/smartMapping/SmartMappingSliderBase, esri/widgets/support/DatePicker, esri/widgets/support/TimePicker, esri/widgets/Swipe, esri/widgets/TableList, esri/widgets/TimeSlider, esri/widgets/Track, esri/widgets/Widget, esri/widgets/Zoom - Added property:
visible
to esri/widgets/smartMapping/ClassedColorSlider, esri/widgets/smartMapping/ClassedSizeSlider, esri/widgets/smartMapping/ColorSizeSlider, esri/widgets/smartMapping/ColorSlider, esri/widgets/smartMapping/OpacitySlider, esri/widgets/smartMapping/SizeSlider, esri/widgets/smartMapping/SmartMappingSliderBaseElements - Added an additional
number
property,num
, to theLods options
passed into TileInfo.create().
Deprecated classes, properties, methods, events
The following are deprecated and will be removed in a future release:
- AddressCandidate deprecated since version 4.20. Use AddressCandidate instead.
- AlgorithmicColorRamp deprecated since version 4.20. Use AlgorithmicColorRamp instead.
- AreasAndLengthsParameters deprecated since version 4.20. Use AreasAndLengthsParameters instead.
- AttachmentInfo deprecated since version 4.19. Use AttachmentInfo instead.
- AttachmentQuery deprecated since version 4.20. Use AttachmentQuery instead.
- BasemapLayerList.statusIndicatorsVisible deprecated since version 4.15. Use BasemapLayerList.visibleElements.statusIndicators instead.
- Bookmark.extent deprecated since 4.17. Use viewpoint instead.
- Bookmarks.bookmarkCreationOptions deprecated since 4.18. Use
default
instead.Create Options - BufferParameters deprecated since version 4.20. Use BufferParameters instead.
- ChartMediaInfoValueSeries.x deprecated since version 4.17. Use value instead.
- ChartMediaInfoValueSeries.y deprecated since version 4.17. Use tooltip instead.
- ClosestFacilityParameters deprecated since version 4.20. Use ClosestFacilityParameters instead.
- ClosestFacilitySolveResult deprecated since version 4.20. Use ClosestFacilitySolveResult instead.
- ClosestFacilityTask deprecated since version 4.20. Use closestFacility instead.
- ColorRamp deprecated since version 4.20. Use ColorRamp instead.
- DataFile deprecated since version 4.20. Use DataFile instead.
- DataLayer deprecated since version 4.20. Use DataLayer instead.
- decorators.cast(classFunction) deprecated since version 4.14. Parameter decorators won't be supported by JavaScript decorators.
- decorators.declared deprecated since version 4.16.
declared()
is not needed to extend Accessor anymore. See Implementing Accessor for updated information. - DensifyParameters deprecated since version 4.20. Use DensifyParameters instead.
- DirectionsFeatureSet deprecated since version 4.20. Use DirectionsFeatureSet instead.
- DistanceParameters deprecated since version 4.20. Use DistanceParameters instead.
- FeatureForm.description deprecated since version 4.20. Set it via the FormTemplate.description.
- FeatureForm.fieldConfig deprecated since version 4.16. Use FieldElement and/or GroupElement instead.
- FeatureForm.title deprecated since version 4.20. Set it via the FormTemplate.title.
- FeatureFormViewModel.description deprecated since version 4.20. Set it via the FormTemplate.description.
- FeatureFormViewModel.fieldConfig deprecated since version 4.16. Use FieldElement and/or GroupElement instead.
- FeatureFormViewModel.title deprecated since version 4.20. Set it via the FormTemplate.title.
- FeatureSet deprecated since version 4.20. Use FeatureSet instead.
- FindParameters deprecated since version 4.20. Use FindParameters instead.
- FindResult deprecated since version 4.20. Use FindResult instead.
- FindTask deprecated since version 4.20. Use find instead.
- GeneralizeParameters deprecated since version 4.20. Use GeneralizeParameters instead.
- GeometryService deprecated since version 4.20. Use geometryService instead.
- Geoprocessor deprecated since version 4.20. Use geoprocessor instead.
- GPMessage deprecated since version 4.20. Use GPMessage instead.
- IdentifyParameters deprecated since version 4.20. Use IdentifyParameters instead.
- IdentifyResult deprecated since version 4.20. Use IdentifyResult instead.
- IdentifyTask deprecated since version 4.20. Use identify instead.
- ImageHistogramParameters deprecated since version 4.20. Use ImageHistogramParameters instead.
- ImageIdentifyParameters deprecated since version 4.20. Use ImageIdentifyParameters instead.
- ImageIdentifyResult deprecated since version 4.20. Use ImageIdentifyResult instead.
- ImageIdentifyTask deprecated since version 4.20. Use imageService instead.
- ImageServiceIdentifyParameters deprecated since version 4.18. Use ImageIdentifyParameters instead.
- ImageServiceIdentifyResult deprecated since version 4.18. Use ImageIdentifyResult instead.
- ImageServiceIdentifyTask deprecated since version 4.18. Use imageService instead.
- JobInfo deprecated since version 4.20. Use JobInfo instead.
- LabelClass.labelExpressionInfo.value deprecated since version 4.5. Use expression instead.
- LayerList.statusIndicatorsVisible deprecated since version 4.15. Use LayerList.visibleElements.statusIndicators instead.
- LegendLayer deprecated since version 4.20. Use LegendLayer instead.
- LengthsParameters deprecated since version 4.20. Use LengthsParameters instead.
- LinearUnit deprecated since version 4.20. Use LinearUnit instead.
- Locator deprecated since version 4.20. Use locator instead.
- MultipartColorRamp deprecated since version 4.20. Use MultipartColorRamp instead.
- NAMessage deprecated since version 4.20. Use NAMessage instead.
- OffsetParameters deprecated since version 4.20. Use OffsetParameters instead.
- ParameterValue deprecated since version 4.20. Use ParameterValue instead.
- PathSymbol3DLayer.size deprecated since version 4.12. Use PathSymbol3DLayer.width or PathSymbol3DLayer.height instead.
- PointDrawAction.coordinates deprecated since version 4.19. Use vertices instead.
- PrintParameters deprecated since version 4.20. Use PrintParameters instead.
- PrintTask deprecated since version 4.20. Use print instead.
- PrintTemplate deprecated since version 4.20. Use PrintTemplate instead.
- projection.isSupported deprecated since version 4.18.
- ProjectParameters deprecated since version 4.20. Use ProjectParameters instead.
- promiseUtils.reject deprecated since version 4.19. Use the native Promise.reject() method instead.
- promiseUtils.resolve deprecated since version 4.19. Use the native Promise.resolve() method instead.
- Query deprecated since version 4.20. Use Query instead.
- QueryTask deprecated since version 4.20. Use query instead.
- RasterData deprecated since version 4.20. Use RasterData instead.
- RelationParameters deprecated since version 4.20. Use RelationParameters instead.
- RelationshipQuery deprecated since version 4.20. Use RelationshipQuery instead.
- RouteParameters deprecated since version 4.20. Use RouteParameters instead.
- RouteResult deprecated since version 4.20. Use RouteResult instead.
- RouteTask deprecated since version 4.20. Use route instead.
- SceneView.constraints.collision deprecated since version 4.8. Use Ground.navigationConstraint instead.
- ServiceAreaParameters deprecated since version 4.20. Use ServiceAreaParameters instead.
- ServiceAreaSolveResult deprecated since version 4.20. Use ServiceAreaSolveResult instead.
- ServiceAreaTask deprecated since version 4.20. Use serviceArea instead.
- SizeVariable.expression deprecated since version 4.2. Use SizeVariable.valueExpression instead.
- Slider.labelsVisible deprecated since version 4.15. Use Slider.visibleElements.labels instead.
- Slider.rangeLabelsVisible deprecated since version 4.15. Use Slider.visibleElements.rangeLabels instead.
Smart
deprecated since version 4.13. UseMapping.params.basemap view
instead.- StatisticDefinition deprecated since version 4.20. Use StatisticDefinition instead.
- symbolPreview.renderPreviewHTML deprecated since version 4.11. Use symbolUtils.renderPreviewHTML instead.
- symbolPreview deprecated since version 4.11. Use symbolUtils instead.
- Task deprecated since version 4.20.
- TimeSlider.values deprecated since version 4.20. Use timeExtent instead.
- TimeSliderViewModel.values deprecated since version 4.20. Use timeExtent instead.
- TrimExtendParameters deprecated since version 4.20. Use TrimExtendParameters instead.
- widget.renderable deprecated since version 4.19. All properties are automatically tracked now and don't need to be decorated with this decorator.
- The light-blue, dark-blue, light-green, dark-green, light-purple, dark-purple, light-red, dark-red themes have been deprecated since 4.19. Please use
light
ordark
instead, or create your own theme.
Breaking changes
- CodedValueDomain.codedValues now returns an array of CodedValue type definition objects. Prior to this, it used an array of generic objects. The properties within the
coded
have not changed.Value - The keyboard shortcut key has changed from
Ctrl
toShift
in Sketch and SketchViewModel for creating apolygon
graphic (rectangle
orcircle
) with predefined shapes. - The keyboard shortcut key has changed from
C
toEnter
in Sketch, SketchViewModel, and Draw for completing apolyline
,polygon
, orpoint
graphic.
The following classes, methods, properties and events have been deprecated for at least 2 releases and have now been removed from the API:
Class/Property/Method/Event | Alternate option | Version deprecated |
---|---|---|
Bookmarks.select-bookmark | Bookmarks.bookmark-select | 4.17 |
Bookmarks | BookmarksViewModel.BookmarkOptions.captureViewpoint | 4.17 |
Feature | FeatureTemplates.visibleElements.filter | 4.15 |
Popup.feature | Popup.visibleElements.featureNavigation | 4.15 |
Please refer to the Breaking changes guide topic for a complete list of breaking changes across all releases of the 4x API.
Bug fixes and enhancements
- BUG-000130223: Fixed an issue where some FeatureLayers and MapImageLayers would not draw on the specific Android devices when the opacity was not set to 1.
- BUG-000132202: Fixed an issue where FeatureLayer.refresh() method was throwing an error.
- BUG-000139909: Fixed an issue where map services added to web maps using the root service URL would not display properly on certain Android devices.
- BUG-000135228: Fixed an issue where the
Set scale
option of the Print widget would lock the extent for successive map printouts. - BUG-000135386: Fixed an issue where the StreamLayer.purgeOptions.ageReceived settings were not properly honored.
- BUG-000136368: Fixed an issue where the popup doesn't request the feature geometry when some geometry functions are used in Arcade expressions.
- BUG-000137657: Fixed an issue where symbols with unique value set to
null
fail to render when there are two fields in UniqueValueRenderer. - BUG-000137883: Fixed an issue where labels would not update correctly after editing their associated values using the Editor widget.
- BUG-000137996: Fixed an issue where simultaneous creation of multiple hierarchical group layers causes UI blocking.
- BUG-000138085: Fixed an issue VectorTileLayer.currentStyleInfo.style was not reflecting changes made to the VectorTileLayer's style.
- BUG-000138460: Fixed an issue where some layers showed an offset when zooming in. This was done by providing the ability to set the MapView's scale 1:1 by creating additional LODs via TileInfo.create() and set the generated LODs in the MapView.constraints.
- BUG-000138666: Fixed an issue where the TimeSlider widget was throwing an error when
full
was set while the TimeSlider widget is invisible.Time Extent - BUG-000139192: Fixed an issue where the multipoint geometries are split into two or more points after zooming past certain scales.
- BUG-000139265: Fixed an issue where ImageryTileLayer is not displayed if it does not have fixed level factors.
- BUG-000139825: Fixed an issue where the performance of VectorTileLayers was impacted when panning.
- BUG-000139849: Fixed an issue with the Sketch widget where it would not remove a graphic via the
esc
key after clicking theundo
button. - BUG-000140487: Fixed an issue where the geodatabase version information was not included in identify and IdentifyTask operations.
- BUG-000140489: Fixed an issue where the sorted graphics in GraphicsLayer lose the order after zooming in or out on the map.
- BUG-000140061: Fixed an issue where passing a layer from ArcGIS Server as a FeatureSnappingLayerSource was causing timeouts.
- BUG-000139631: Fixed an issue where drawing a geometry using Sketch or SketchViewModel was throwing console errors whenever the Map projection was not in Web Mercator or WGS 84.
- BUG-000139485: Fixed an issue where the Legend is not updated when the RasterRenderingRule is changed.
- Esri Community - 1046301: Added a property called
features
on PopupViewModel.P e r Page - Esri Community - 1048724: Added a support for new characters for the coordinateFormatter.fromLatitudeLongitude() method.
- Esri Community - 1051281: Fixed an issue where FeatureLayer.historicMoment was not being honored after the initial change.
- Esri Community - 1052876: Fixed an issue where PictureMarkerSymbols were being cut off along tile edges.
- Esri Community - 1056484: Fixed an issue where ImageryLayer was throwing a warning when UniqueValueRenderer and renderingRule are both set on
Imagerylayer
. - Esri Community - 1058789: Fixed an issue where the OGCFeatureLayer was requesting the wrong CRS for some services, which could cause a slight offset.
- Esri Community - 1058935: Fixed an issue where updates made to a map's FeatureLayer did not reflect within the corresponding FeatureTable widget.
- Esri Community - 1059410: Fixed an issue where ImageryLayer was throwing an error when
lerc
format was requested. - GitHub - 109 - Fixed an issue with
esri-loader
throwing unhandled promise exceptions when used with Angular 12 and zone.js. - GitHub - 296 - Fixed an issue where sometimes the map would not display when adding a ScaleBar widget to a MapImageLayer without a basemap.
- Fixed an issue in 2D MapViews where labels would not display if the where clause used a field that wasn't in the labelExpressionInfo.expression.
- Fixed an issue where a CIMSymbol with CIMPictureMarker symbol layer would not render when the image size was too large.
- Fixed an issue where a multi-level nested CIMSymbol would not render.
- Fixed an issue where CIMSymbol's
primitive
was not working with theOverride color
property of aCIMSolid
symbol layer.Fill - Fixed an issue where features with null geometries could display off the map.
- Fixed an issue where hitTest would fail on a line CIMSymbol with a VectorMarker symbol layer.
- Fixed an issue where printing clustered features with different browser window sizes caused inconsistent print results.
- Fixed an issue where the font color of links in the FeatureTable in
dark
theme did not have enough contrast with the table's background color. - Fixed an issue where the layer fails to draw features when some Arcade geometry functions are used in the renderer.
- Fixed an issue where the Search widget suggestions dropdown could remain open when a user clicks on another HTML element on the page.
- Fixed an issue where time enabled layers were not printed properly with the Print widget and PrintTask.
- Fixed an issue where visual variables were not being honored when set on a DictionaryRenderer.
- Fixed an issue with the geometryEngine.difference() method failing with some line geometries.
- Fixed an issue with the LayerList widget behavior when working with non-dynamic MapImageLayer sublayers.
- Fixed an issue with the z-order of symbol layers in a CIMSymbol.
- Fixed an issue in 3D SceneViews where
Rotate
was not returning correct degree values.Event Info.angle - Esri Community - 1009348: Enhanced the CoordinateConversion widget to use sessionStorage with a new storageEnabled property.
- Enhanced summaryStatistics by adding support for string fields.
- Enhanced summaryStatistics by adding a
nullcount
property inSummary
to return the number of null values stored in a given field.Statistics Result - Enhanced SceneLayer by supporting Basis Texture and 3D extent provided by I3S version 1.8.
Additional packages
Version 4.20 of the ArcGIS Maps SDK for JavaScript uses ArcGIS Arcade 1.13 (since 4.19).
Downloads
Dojo
will no longer be included as part of our API downloads because the API no longer uses it (as of version 4.18).
How to access the SDK
- The API library is available on both CDN and npm, Read more at Get started.
- For supported versions, you can also download both the documentation and the API library. These downloads are typically available 3-4 weeks after release.
Previous releases
- Version 4.29 - February 2024
- Version 4.28 - October 2023
- Version 4.27 - June 2023
- Version 4.26 - February 2023
- Version 4.25 - November 2022
- Version 4.24 - June 2022
- Version 4.23 - March 2022
- Version 4.22 - December 2021
- Version 4.21 - September 2021
- Version 4.20 - June 2021
- Version 4.19 - April 2021