What is a scene?
A scene is a container for layers. You use a scene together with a scene view to display layers of geographic data in 3D. Most applications contain a basemap layer to display geographic data with streets or satellite imagery, but you can also use data layers and graphics to display additional data.
You can use a scene and a scene view to:
- Display basemap layers such as streets, topographic, light gray canvas, or satellite imagery.
- Display basemap layers with custom styles.
- Display data layers that contain feature, vector tile, or tile layer data.
- Display temporary points, lines, polygons, or text as graphics.
- Display terrain with elevation.
- Display real-world objects such as buildings, cars, and trees.
- Display 3D visualizations of 2D objects.
- Provide an interface with which your users can zoom, pan, and interact.
- Inspect layers and display attribute information.
- Display layers in a web scene.
How a scene works
A scene and a scene view work together to display layers in 3D. A scene manages layers and a scene view displays layer data. A scene typically contains a basemap layer that references an elevation layer (or service), and one or more data layers. A scene view combines all of the layers (and graphics) into a single display.
Data sources
Each layer in a scene references a data source. A layer's data source provides the geographic data that is displayed in the scene view. The main data source for a basemap layer is the basemap styles service. The data source for a data layer can be a file, collection of local data, or a data service. Learn more about data sources in Basemap styles service and Data layers.
Scene layers
Scenes can also contain scene layers to provide more advanced 3D visualizations. Scene services provide high-resolution 3D data of landscapes, cities, buildings, and other 3D object types. To learn more about publishing scene services, visit Scene services in the ArcGIS Enterprise documentation.
Layer order
When a scene view displays a scene, the layers and graphics are displayed from the bottom up, therefore, it is important to add a basemap layer and elevation layer (or service) first, then data layers, and then graphics.
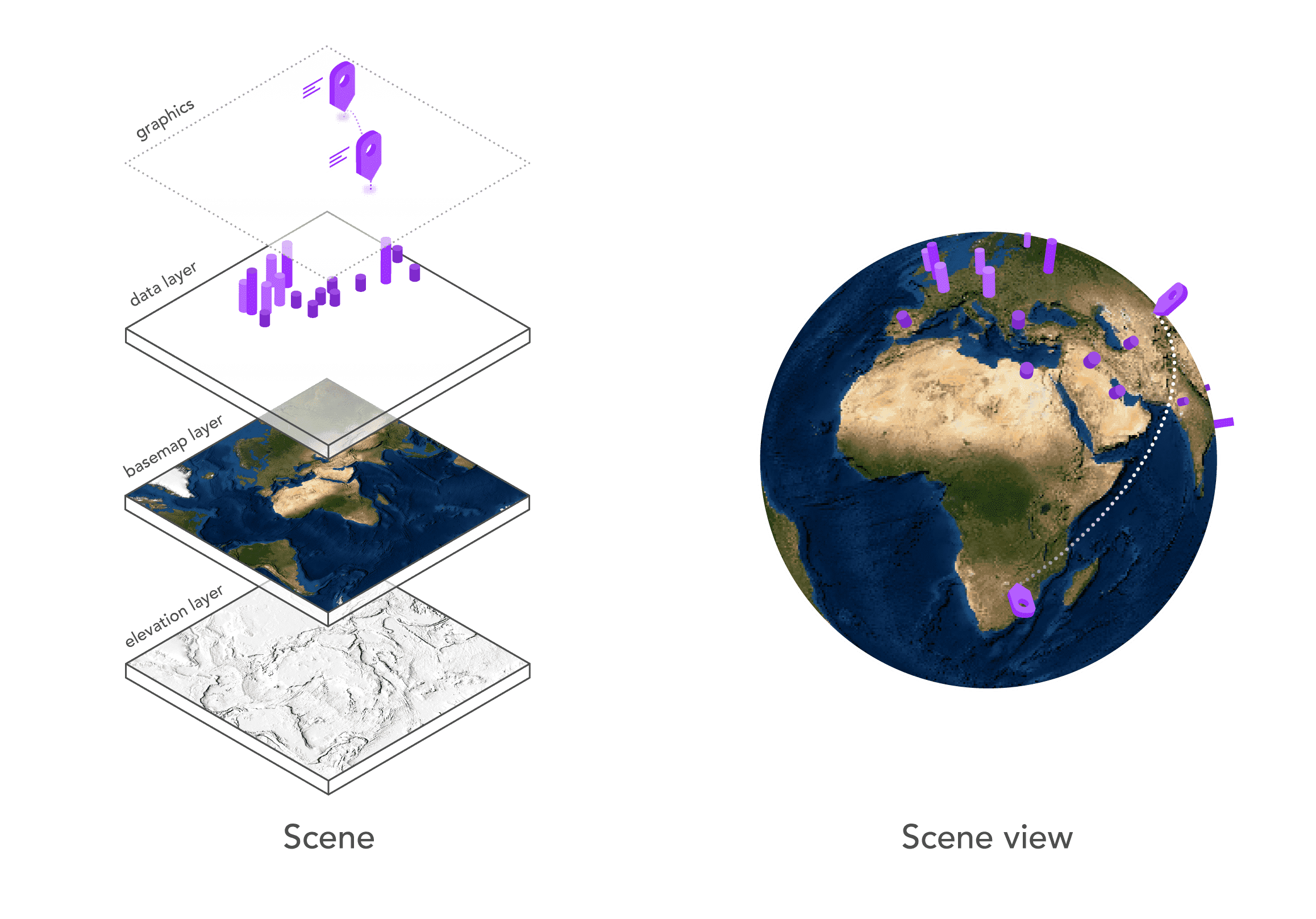
Figure 1: A scene and scene view work together to display layers and graphics.
Scene
A scene contains a collection of layers for a mapping application. You use it to add, remove, order, and set the visibility for all layers you would like to display. For a simple application, you can just add a basemap layer, and optionally, reference an elevation layer (or service). Scenes use values in an elevation layer to determine the ground height (surface). In most cases, all other layers are draped on top of a basemap layer that has elevation defined. Layers can also have a relative position above or below the surface.
A basemap layer is the most important layer in a scene as it provides the overall visual context for the scene. Most APIs provide an enumeration to make it easier to access a basemap layer's data source. Other APIs require you to reference the service URL and style for the desired basemap.
esriConfig.apiKey = "YOUR_API_KEY";
const scene = new Map({
basemap: "arcgis-topographic", // Basemap layer
ground: "world-elevation" // Elevation service
});
Scene view
A scene view is a user interface that displays layers in a scene and graphics in 3D. Its primary role is to display all of the geographic data for a specific area of the scene. The visible area is known as the extent.
A scene view also provides a user interface for the scene and its layers. It supports user interactions such as click, tap, swipe, and pinch. You can use these events to access data in layers or graphics. Click events are typically used to access feature attributes and display them in a pop-up.
To create a scene view, you typically set the scene and the camera position. Unlike a map, a scene uses a camera to define the viewer's perspective and determine the visible extent. The perspective is defined by setting the camera's position, location, altitude (height), heading and tilt. You also set the container to connect the view to a visible component in the application.
const view = new SceneView({
map: scene,
camera: {
position: {
x: -118.808,
y: 33.961,
z: 2000 // meters
},
tilt: 75
},
container: "viewDiv"
});
Code examples
Display a scene with elevation
This example uses a scene and scene view to displays the topographic basemap layer. The basemap layer is draped on the elevation layer to create a 3D view. The camera for the scene is set to -118.808, 33.961 (longitude, latitude) at an altitude of 2000 meters. The tilt is set to 75 degrees. The second example displays the same scene with the satellite imagery basemap layer.
Steps
- Create a map or scene and add a basemap layer and elevation layer (or service).
- Create a scene view and set the camera position.
Topographic basemap layer
esriConfig.apiKey = "YOUR_API_KEY";
const map = new Map({
basemap: "arcgis-topographic",
ground: "world-elevation"
});
const view = new SceneView({
map: map,
camera: {
position: {
x: -118.808,
y: 33.961,
z: 2000 // meters
},
tilt: 75
},
container: "viewDiv"
});
Satellite imagery basemap layer
const map = new Map({
basemap: "arcgis-imagery", //Basemap styles service
ground: "world-elevation" //Elevation service
});
const view = new SceneView({
container: "viewDiv",
map: map,
camera: {
position: {
x: -118.808, //Longitude
y: 33.961, //Latitude
z: 2000 // Meters
},
tilt: 75
}
});
Display 2D data in 3D
This example illustrates how to use a renderer to display 2D features in 3D with a scene view.
Steps
- Create a map or scene and add a basemap layer and reference an elevation layer (or service).
- Add a data layer or graphics with points, lines, or polygons.
- Set a layer renderer style the points with 3D symbols.
- Set the scene to the scene view.
- Set the camera position.
const transitLayer = new FeatureLayer({
url:
"http://services.arcgis.com/V6ZHFr6zdgNZuVG0/arcgis/rest/services/subway_tcl_stations_lines_wgs84/FeatureServer/0",
copyright:
"Data from <a href='https://data.beta.grandlyon.com/en/datasets/lignes-metro-funiculaire-reseau-transports-commun-lyonnais/info'>Data Grand Lyon - Sytral</a>",
elevationInfo: {
mode: "relative-to-ground",
offset: 10
},
title: "Transit lines in Lyon",
definitionExpression: "sens='Aller'",
outFields: ["*"]
});
webscene.add(transitLayer);
//Render unique path symbol for each transit line
function renderTransitLayer() {
const renderer = new UniqueValueRenderer({
field: "ligne"
});
for (let property in colors) {
if (colors.hasOwnProperty(property)) {
renderer.addUniqueValueInfo({
value: property,
symbol: {
type: "line-3d",
symbolLayers: [
{
type: "path",
profile: options.profile,
material: {
color: colors[property]
},
width: options.width,
height: options.height,
join: options.join,
cap: options.cap,
anchor: "bottom",
profileRotation: options.profileRotation
}
]
}
});
}
}
transitLayer.renderer = renderer;
}
Display a scene service with textured buildings
This example shows to display 3D objects such as building, trees, and cars using a scene layer that references a scene service data source. The scene service must be created and published with ArcGIS prior to creating the application. Once created, the service can be referenced by URL or by its item ID.
Steps
- Create a map or scene and add a basemap layer and reference an elevation layer (or service).
- Add a scene layer that references a scene service with textured buildings.
- Set the scene to the scene view.
- Set the camera position.
const sceneLayer = new SceneLayer({
portalItem: {
id: "2342ab7928834076a1240fb93c60e978"
},
elevationInfo: {
mode: "absolute-height",
offset: 6
}
});
// Create Map
const map = new Map({
basemap: "hybrid", //Basemap styles service
ground: "world-elevation", //Elevation service
layers: [sceneLayer]
});
// Create the SceneView
const view = new SceneView({
container: "viewDiv",
map: map,
camera: {
position: [4.84361, 45.75561, 270],
tilt: 82,
heading: 304
}
});
Display a scene service with an integrated mesh
This example displays a 3D integrated mesh surface by using a scene layer that references a scene service data source. The scene service must be created and published with ArcGIS prior to creating the application. Once created, the service can be referenced by URL or by its item ID.
Steps
- Create a scene and add an elevation layer and a basemap layer.
- Add a scene layer that references a scene service with an integrated mesh.
- Set the scene to the scene view.
- Set the camera position.
const meshLayer = new IntegratedMeshLayer({
url: "https://tiles.arcgis.com/tiles/cFEFS0EWrhfDeVw9/arcgis/rest/services/Buildings_Frankfurt_2021/SceneServer/layers/0",
copyright: "Aerowest GmbH",
title: "Integrated Mesh Frankfurt"
});
Tutorials

Display a map
Create and display a map with the basemap styles service.
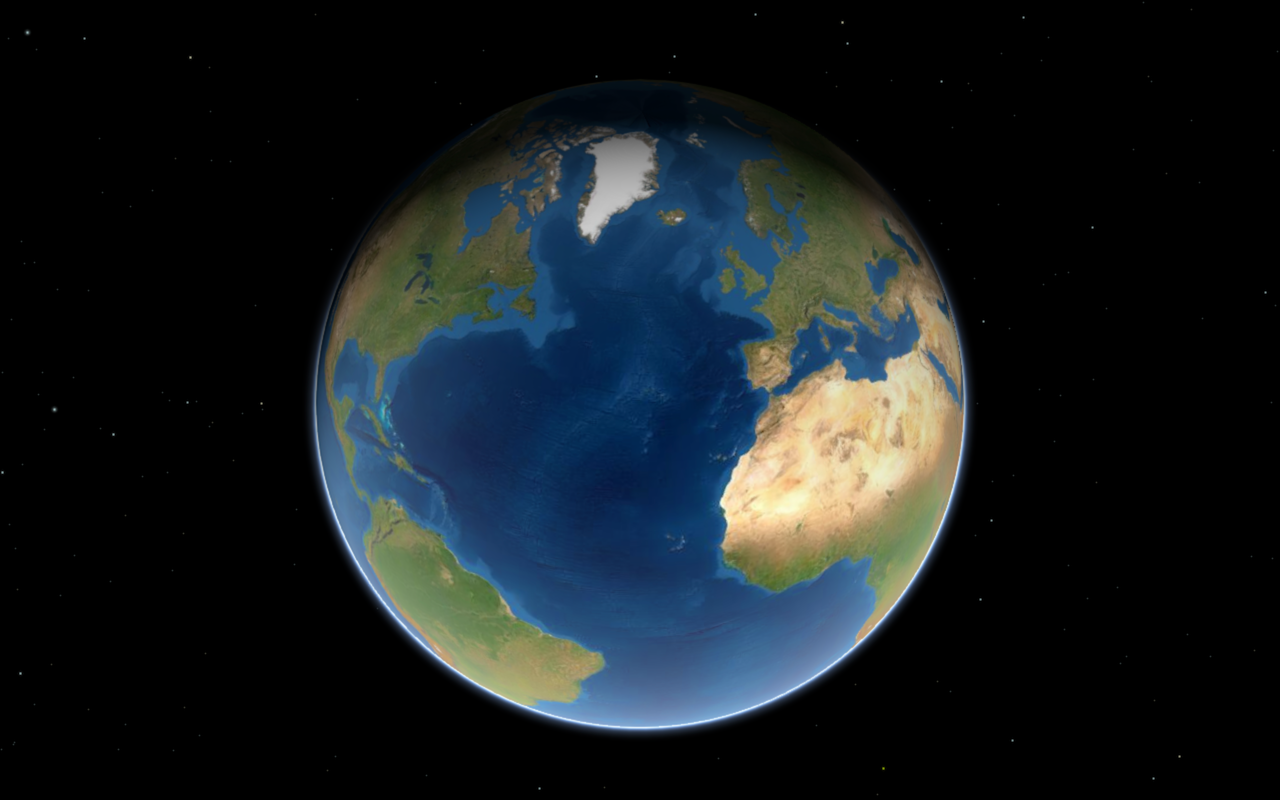
Display a scene
Display a scene with the basemap styles service.
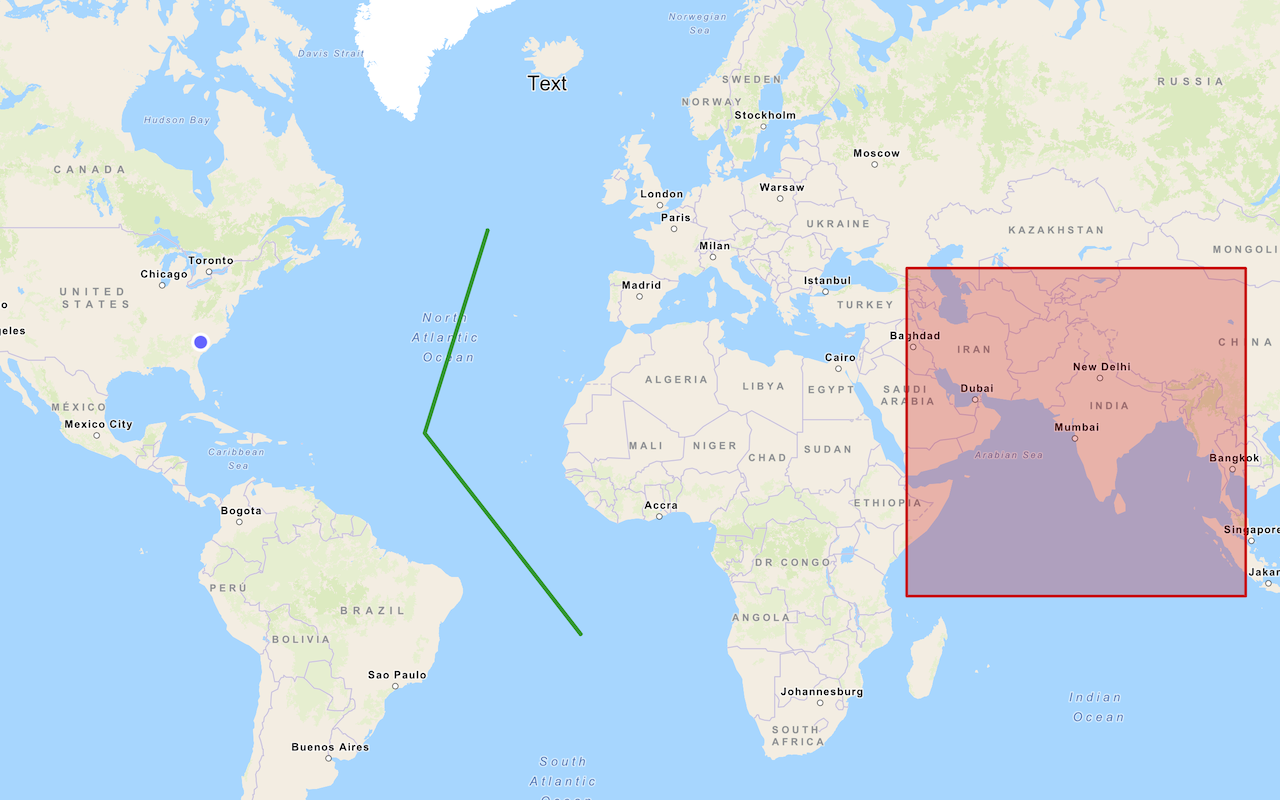
Add a point, line, and polygon
Display point, line, and polygon graphics in a map.
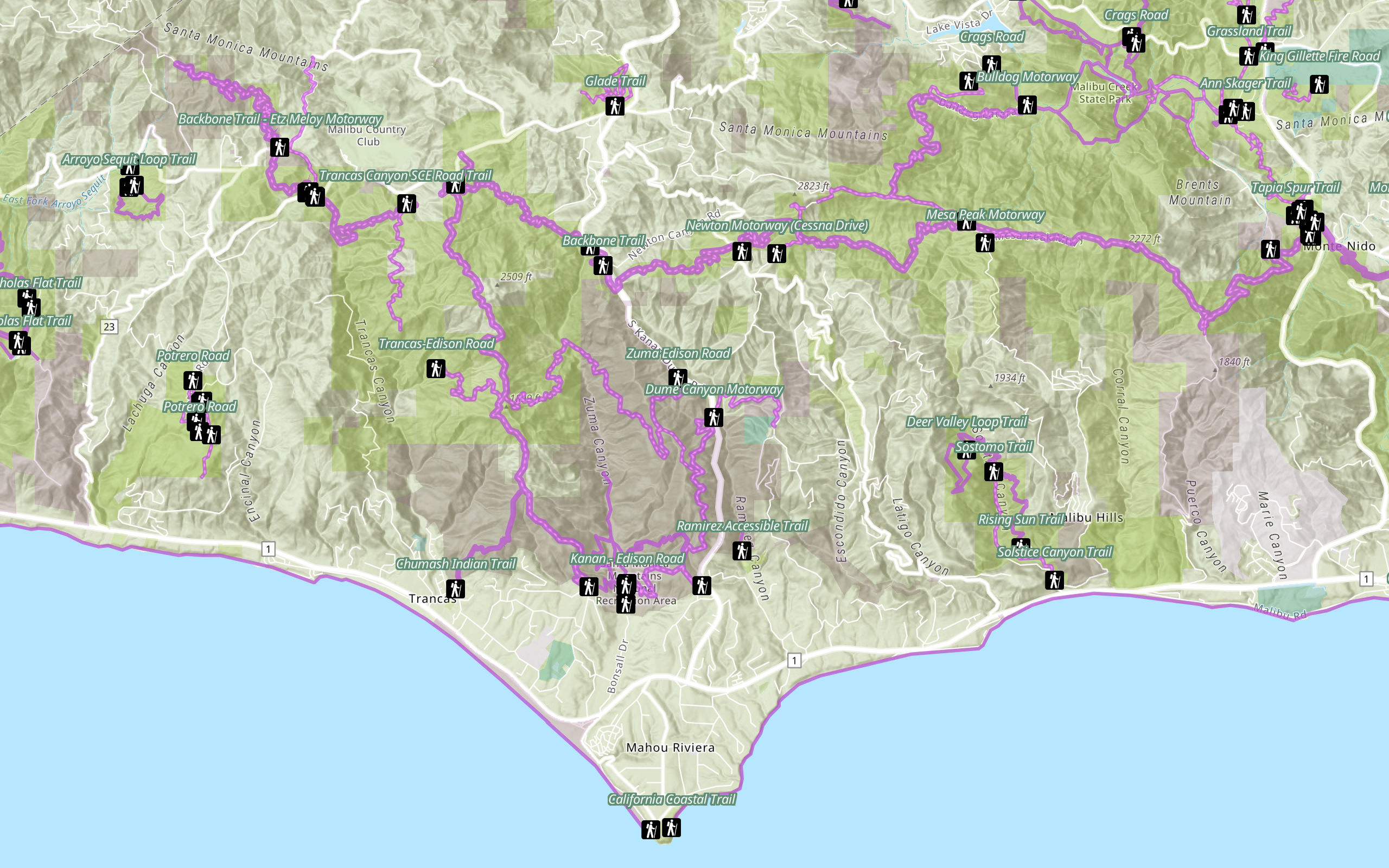
Add a feature layer
Access and display point, line, and polygon features from a feature service.
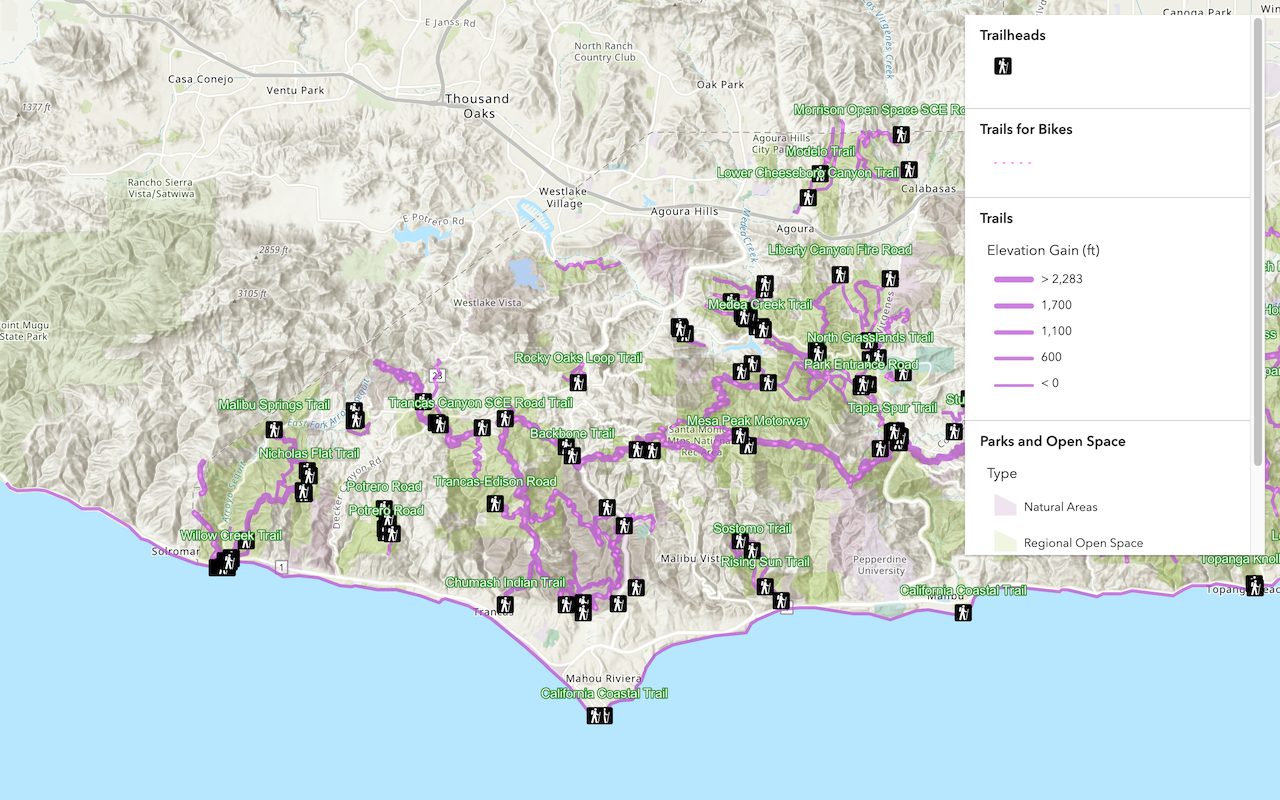
Display a web map
Create and display a map from a web map.
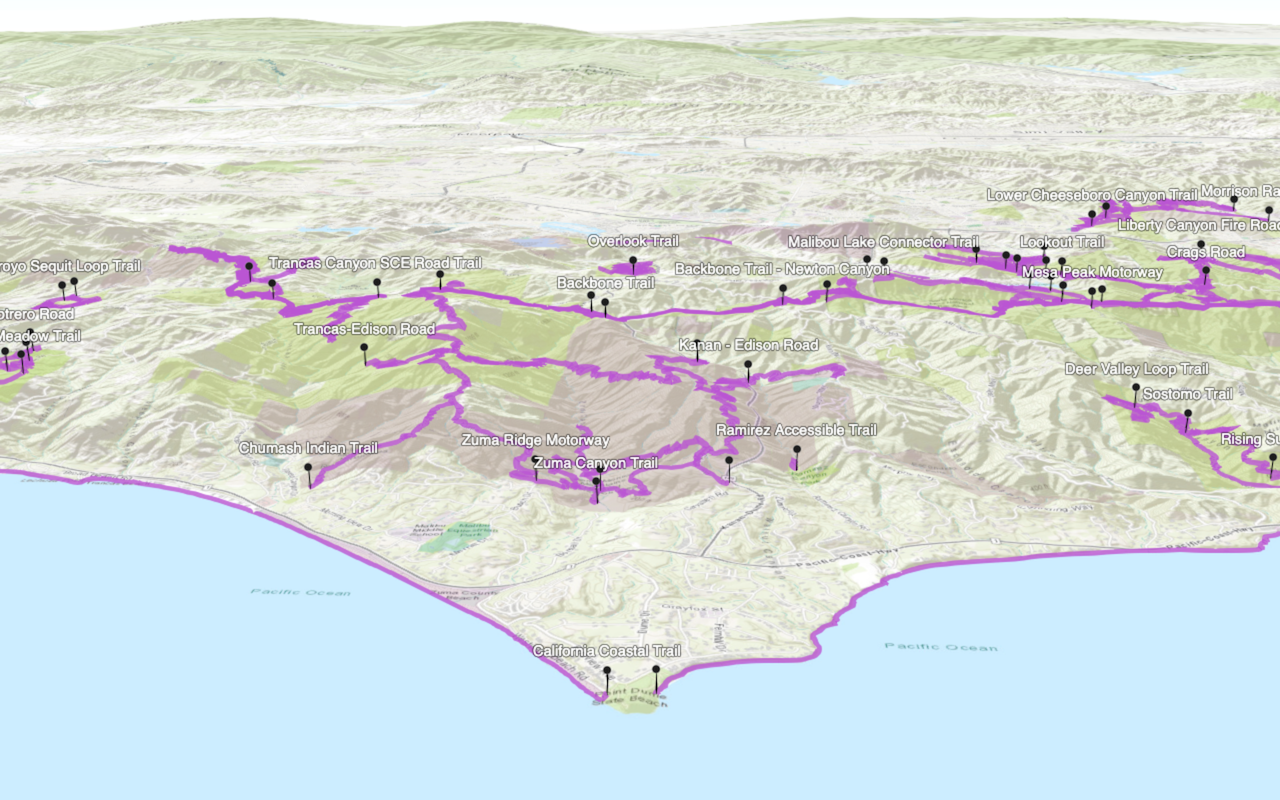
Display a web scene
Create and display a scene from a web scene.
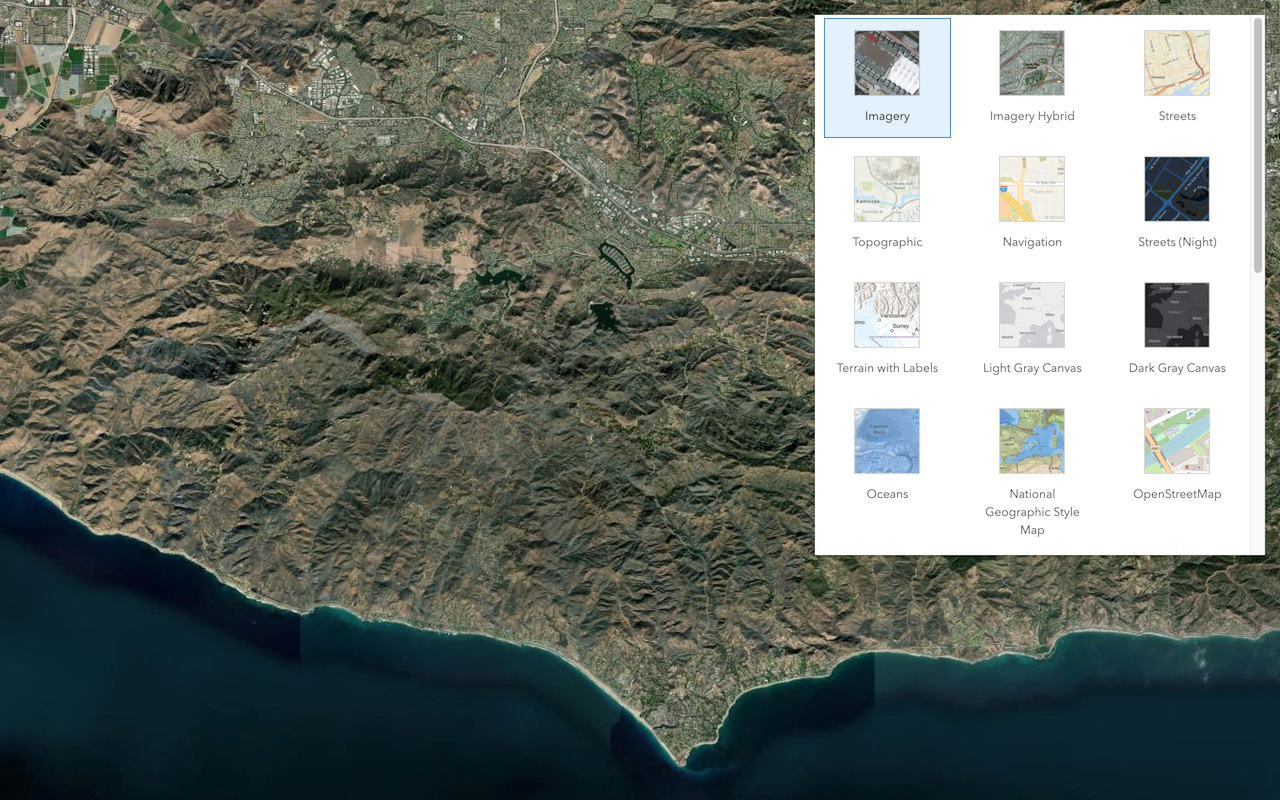
Change the basemap layer
Switch a basemap layer from streets to satellite imagery.
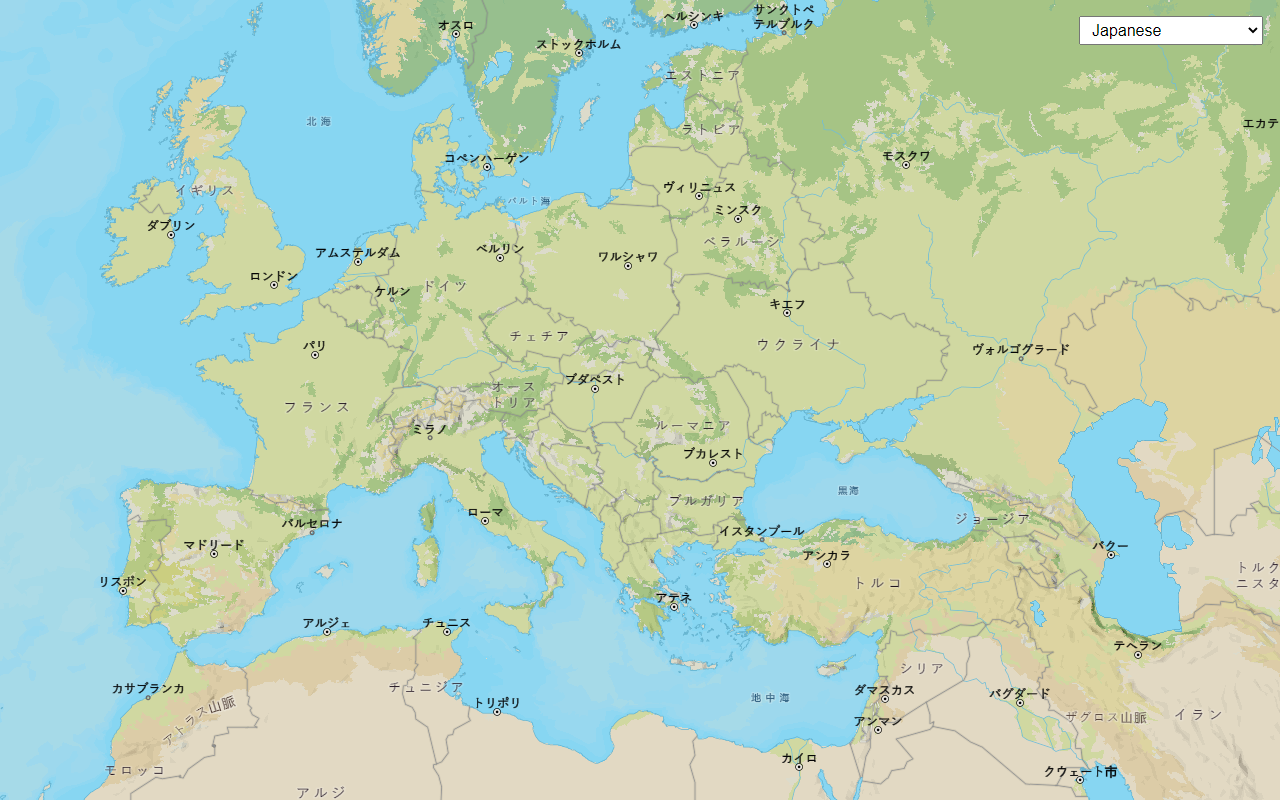
Change the place label language
Switch the language of place labels on a basemap.
Services
Basemap styles service (v1)
Access basemap styles such as streets, navigation, and imagery for maps and scenes.
API support
2D Display | 3D Display | Basemap layers | Basemap places | Data layers | Graphics | Web maps | Web scenes | |
---|---|---|---|---|---|---|---|---|
ArcGIS Maps SDK for JavaScript | 1 | |||||||
ArcGIS Maps SDK for .NET | ||||||||
ArcGIS Maps SDK for Kotlin | ||||||||
ArcGIS Maps SDK for Swift | ||||||||
ArcGIS Maps SDK for Java | ||||||||
ArcGIS Maps SDK for Qt | ||||||||
ArcGIS API for Python | ||||||||
ArcGIS REST JS | 2 | 2 | ||||||
Esri Leaflet | 3 | 4 | ||||||
MapLibre GL JS | 3 | 4 | ||||||
OpenLayers | 1 | 3 | 4 | |||||
CesiumJS | 3 | 4 |
- 1. Display places only.
- 2. Access via HTTP request and authentication.
- 3. Access via Feature layer or Map tile layer.
- 4. Access via layers.