What is a unique types style?
A unique types style assigns distinct symbols to unique data values returned from a field or expression in a layer. You can use this style to visualize what something represents, such as:
- Show points of interest on a map (e.g. schools, parks, places of worship)
- Represent categories of data (e.g. regions, types)
- Visualize ordinal data (e.g. High, medium, and low)
How a unique types style works
This style is configured with a Unique Value Renderer. This renderer requires the following:
- A reference to a data value either from a field name, or an Arcade expression.
- A list of unique value infos that match a unique symbol with an expected value returned from the field or expression.
Code examples
Categorical data
This example demonstrates how to visualize categories using a string value. The app visualizes freeways based on their classification: interstate, state highway, or U.S. highway.
- Create a unique value renderer.
- Reference the field name containing the classification values.
- Create unique value info objects and assign a symbol to each expected value.
- You can optionally add a default symbol to represent all features that don't have a matching value.
const hwyRenderer = {
type: "unique-value",
legendOptions: {
title: "Freeway type",
},
defaultSymbol: otherSym,
defaultLabel: "Other",
field: "RTTYP",
uniqueValueInfos: [
{
value: "I", // code for interstates/freeways
symbol: fwySym,
label: "Interstate",
},
{
value: "U", // code for U.S. highways
symbol: hwySym,
label: "US Highway",
},
{
value: "S", // code for State highways
symbol: stateSym,
label: "State Highway",
},
],
}
Ordinal data
This example demonstrates how to create ordinal categories from a numeric field attribute using an Arcade expression. This app classifies highways by low medium or high truck traffic.
First, write an Arcade expression to classify the data into ordinal categories: low, medium, and high.
var traffic = $feature.AADT;
When(
traffic > 80000, "High",
traffic > 20000, "Medium",
"Low"
);
- Create a unique value renderer.
- Reference the Arcade expression in the
value
property.Expression - Create unique value info objects and assign a symbol to each expected value.
- You can optionally add a default symbol to represent all features that don't have a matching value.
const renderer = {
type: "unique-value",
valueExpression: `
var traffic = $feature.AADT;
When(
traffic > 80000, "High",
traffic > 20000, "Medium",
"Low"
);
`,
valueExpressionTitle: "Traffic volume",
uniqueValueInfos: [{
value: "High",
symbol: {
type: "simple-line",
color: "#810f7c",
width: "6px",
style: "solid"
}
}, {
value: "Medium",
symbol: {
type: "simple-line",
color: "#8c96c6",
width: "3px",
style: "solid"
}
}, {
value: "Low",
symbol: {
type: "simple-line",
color: "#9d978b",
width: "1px",
style: "solid"
}
}]
};
Categorical data (3D)
This example visualizes buildings based on the type of building: residential, commercial or mixed use. A unique value renderer assigns a color to each building based on the building's usage attribute.
Steps
- Create different symbols for each building type.
- Assign the symbols to a unique value renderer.
- Assign the renderer to the scene layer.
const typeRenderer = {
type: "unique-value",
legendOptions: {
title: "Building Type",
},
defaultSymbol: {
type: "mesh-3d",
symbolLayers: [
{
type: "fill",
material: { color: "#FFB55A", colorMixMode: "replace" },
},
],
value: "Others"
},
field: "landuse",
uniqueValueInfos: [
{
value: "MIPS",
symbol: {
type: "mesh-3d",
symbolLayers: [
{
type: "fill",
material: { color: "#FD7F6F", colorMixMode: "replace" },
},
],
},
label: "Office",
},
{
value: "RESIDENT",
symbol: {
type: "mesh-3d",
symbolLayers: [
{
type: "fill",
material: { color: "#7EB0D5", colorMixMode: "replace" },
},
],
},
label: "Residential",
},
{
value: "MIXRES",
symbol: {
type: "mesh-3d",
symbolLayers: [
{
type: "fill",
material: { color: "#BD7EBE", colorMixMode: "replace" },
},
],
},
label: "Mixed use",
},
{
value: "MIXED",
symbol: {
type: "mesh-3d",
symbolLayers: [
{
type: "fill",
material: { color: "#B2E061", colorMixMode: "replace" },
},
],
},
label: "Mixed use without residential",
},
],
}
Tutorials
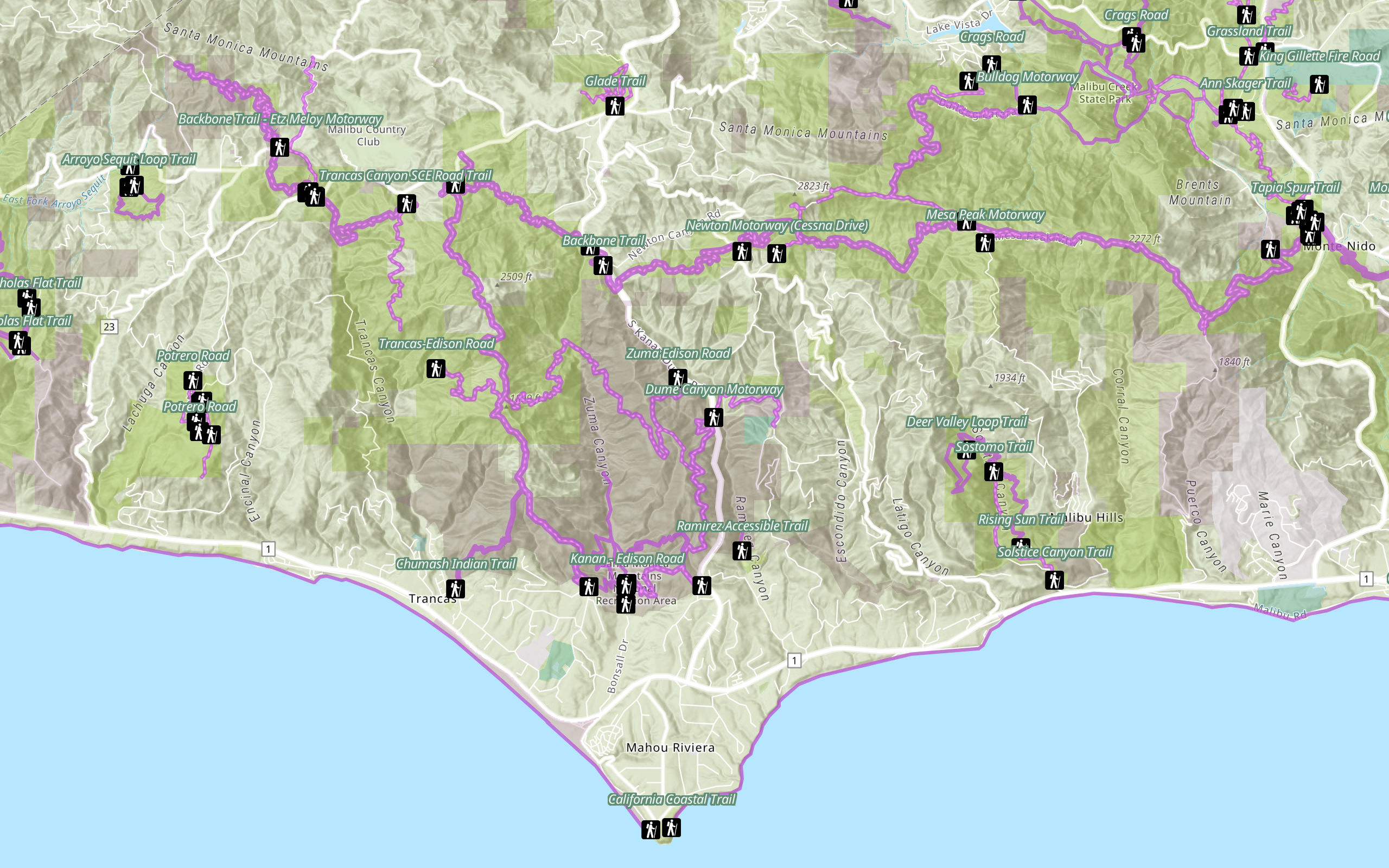
Style a feature layer
Use symbols and renderers to style feature layers.
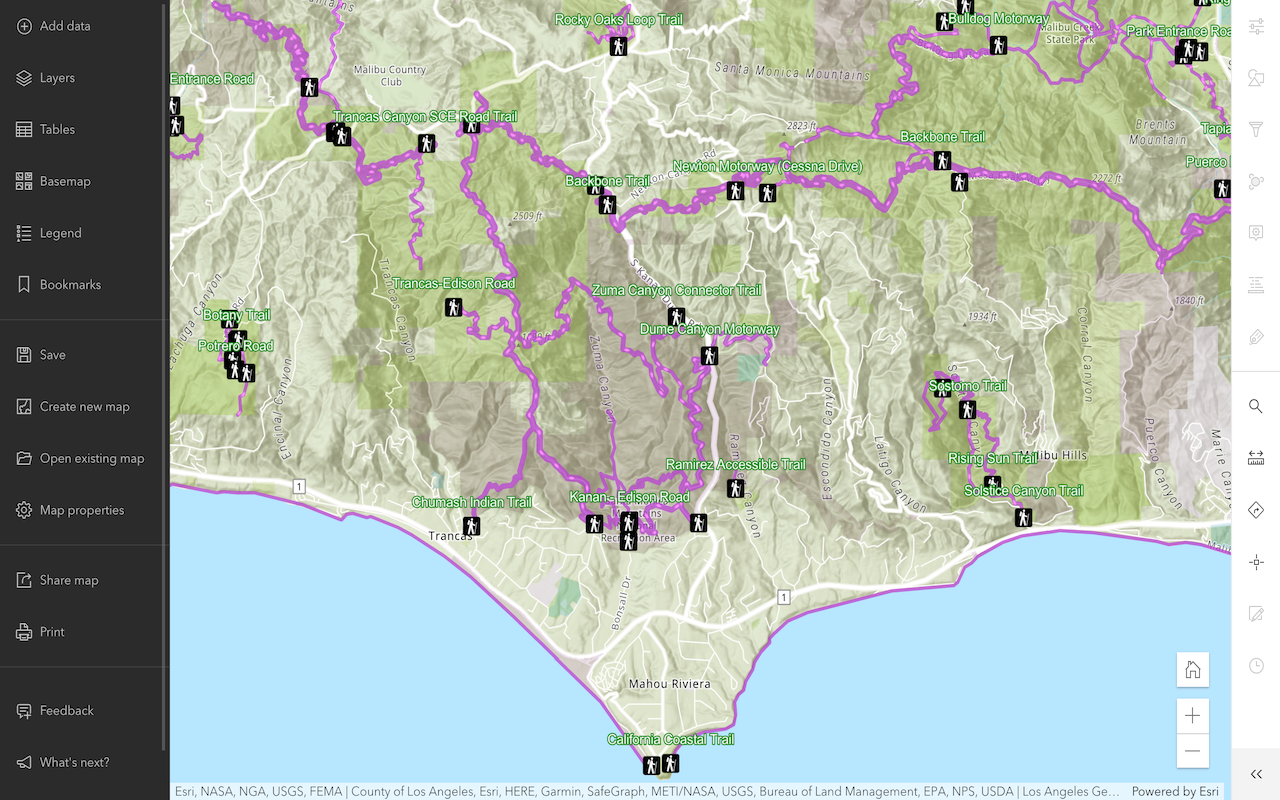
Style layers in a web map
Use Map Viewer to style layers in a web map.
Services
Feature service
Add, update, delete, and query feature data.
API support
Different APIs have different levels of support for data-driven visualization.
Unique types | Class breaks | Visual variables | Time | Multivariate | Predominance | Dot density | Relationship | |
---|---|---|---|---|---|---|---|---|
ArcGIS Maps SDK for JavaScript | ||||||||
ArcGIS Maps SDK for Kotlin | ||||||||
ArcGIS Maps SDK for Swift | ||||||||
ArcGIS Maps SDK for Java | ||||||||
ArcGIS Maps SDK for .NET | ||||||||
ArcGIS Maps SDK for Qt | ||||||||
ArcGIS API for Python |